In this post we cover what is the difference between useMemo and useCallback?
What is the difference between useMemo and useCallback?
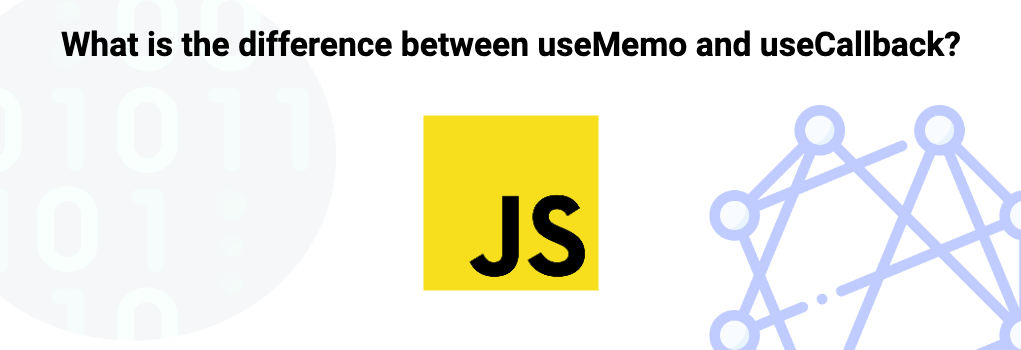
Both useMemo and useCallback are functions in React that can be used in functional components that provide memoization.
Memoization can be thought of as the system remembering something.
The difference between useMemo and useCallback is just what is remembered.
The useCallback hook accepts a callback function and a dependancy array and remembers/memoizes the callback until the dependancies within the dependancy array change.
The useMemo hook accepts the same, a callback and a dependancy array but it remembers the value that is returned from the callback until the dependancies within the dependancy array change.
Both methods are primarily performance enhancements.
1// useMemo
2// numA = 5.
3// numB = 5.
4function CalcComponent({ numA, numB }) {
5 const num = useMemo(
6 () => (numA + numB),
7 [numA, numB]
8 );
9 // num = 10.
10 return (<p>{num}</p>);
11}
12
13// useCallback
14// numA = 5.
15// numB = 5.
16function CalcComponent({ numA, numB }) {
17 const calcNum = useCallback(
18 () => (numA + numB),
19 [numA, numB]
20 );
21 // calcNum = () => (5 + 5).
22 return (<p>{calcNum()}</p>);
23}
Both methods can be used in cases where there is a large computing effort in order to prevent processing the same value for each render in react unless it is absolutely required.
There are other use cases as well which could be to prevent unnecessary calls to other hooks where the values or callbacks are being used.
The useCallback hook can be essential to prevent unnecessary renders of other hooks when using the callback within them.
Usually in this case the best option is creating the function within the specific hook so that it does not need to be passed into the dependancy array. But when that is not an option the useCallback hook is essential due to referential equality.
Identical functions in JavaScript do not have referential equality unless the comparison is comparing two references to the exact same function.
Here is an example of this:
1// referential equality in booleans
2true === true // true
3false === false // true
4
5// referential equality in numbers
60 === 0 // true
71 === 1 // true
8
9// referential equality in functions
10const myFunc = () => 5 + 5;
11myFunc === myFunc // true
12
13// Where functions do not have referential equality
14const myFunc = () => 5 + 5;
15const myOtherFunc = () => 5 + 5;
16
17myFunc === myOtherFunc // false
The reason this is important is because unless a reference to the exact same function is passed into the dependancy array of a hook then it will be seen as a new value causing the hook to be triggered even if it was identical due to how referential equality works with functions.
The useCallback hook solves this by remembering the function between renderers so that it is the same function used for each render until one of its dependancies change.
useMemo
useMemo is a React hook that uses memoization to store/cache/remember a value returned from the function provided to it.
The memoization happens by providing a dependency array, as long as all the values in the dependency array remain the same then the hook will return the stored value for that input set.
When they change, it will process and store the new value for memoization.
This is slightly different to useCallback because useCallback will remember the function passed in whereas useMemo will remember the value returned from it.
In React this comes in handy to prevent extra renders, prevent expensive computations, or to prevent duplicate network calls.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
Functional Component
A functional component in React refers to a normal JavaScript function, that is used as a component and can accept props and return React elements.
This is often used to differentiate between a functional component(ordinary function) and a Class Component in React.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Memoization
Memoization is a term in software development that refers to when a system essentially remembers something. It is a performance optimization where the system will store or cache something and return that value any time it is provided with the same input.
For example an add function given 1, and 1 as parameters will process the parameters by adding them together. A non-memoized version of this function will run that process again and again even if the parameters are the same. Whereas a memoized function will remember the result of 1, and 1 and just return that value rather than processing again.
useCallback
useCallback is a React hook that uses memoization to store/cache/remember a function provided to it.
The memoization happens by providing a dependency array, as long as all the values in the dependency array remain the same then the hook will return the stored function for that input set.
When they change it will process and store the new function for memoization.
This is a little different when compared against useMemo because useMemo remembers the value whereas useCallback remembers the actual function.
In React this comes in handy to prevent extra renders, and to allow functions to be defined within a component and still be passed into props or dependency arrays without causing excess re-renders.
Some context for this is that two identical functions are not actually identical because they have different signatures.
This means that each time a render in React happens, the function will be redefined with a new signature which will trigger a re-render everywhere that function is passed as a prop or in a dependency array.
The only way to prevent this is to use the exact same function so the signature is the same. There are a number of ways to do this and useCallback is one of those options.
Referential equality
Referential equality is when a value is comparably the same at any point that it is referenced. For example booleans or numbers are good examples.
For example true is always equal to true just like false is always equal to false.
Further resources
Related topics
Save code?
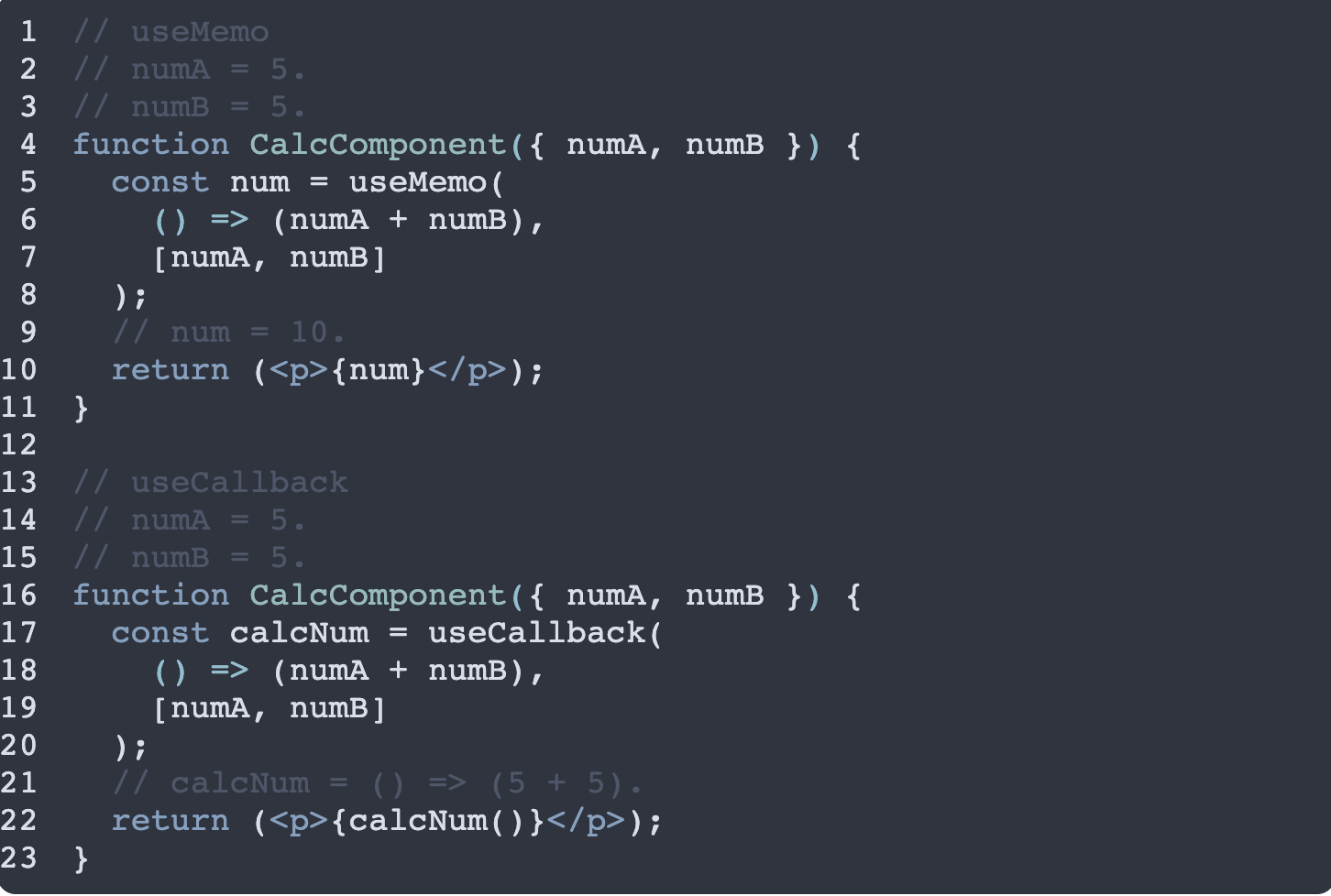