In this post we cover how to rename a destructured variable in JavaScript
How to rename a destructured variable in JavaScript
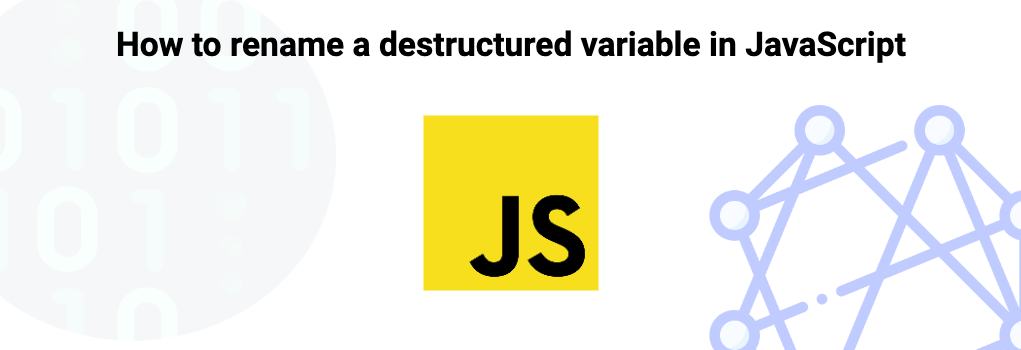
To rename a destructured variable in JavaScript a new name can be applied on a right hand argument after using a colon.
Here is an example of how this looks:
1const data = {
2 coffee: 'robusta',
3 strength: 5,
4};
5
6// coffee gets renamed below to be "coffeeType"
7// strength gets renamed below to be "roastLevel"
8const { coffee: coffeeType, strength: roastLevel } = data;
9
10console.log(coffeeType, roastLevel); // 'robusta', 5.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Destructuring assignment
The Destructuring assignment is JavaScript syntax that allows values to be taken from a compatible datatype without having to use dot notation or indexes.
To use the destructuring assignment an object or array can be opened up, and given names to the values in place to extract them from the datasource.
For the following example: const [itemA, itemB] = [0, 1];
, itemA
and itemB
are now constants that refer to the values 0
and 1
in the array.
Here is another example using an object:
const data = {
coffee: 'robusta',
strength: 5,
};
const { coffee, strength } = data;
Further resources
Related topics
Save code?
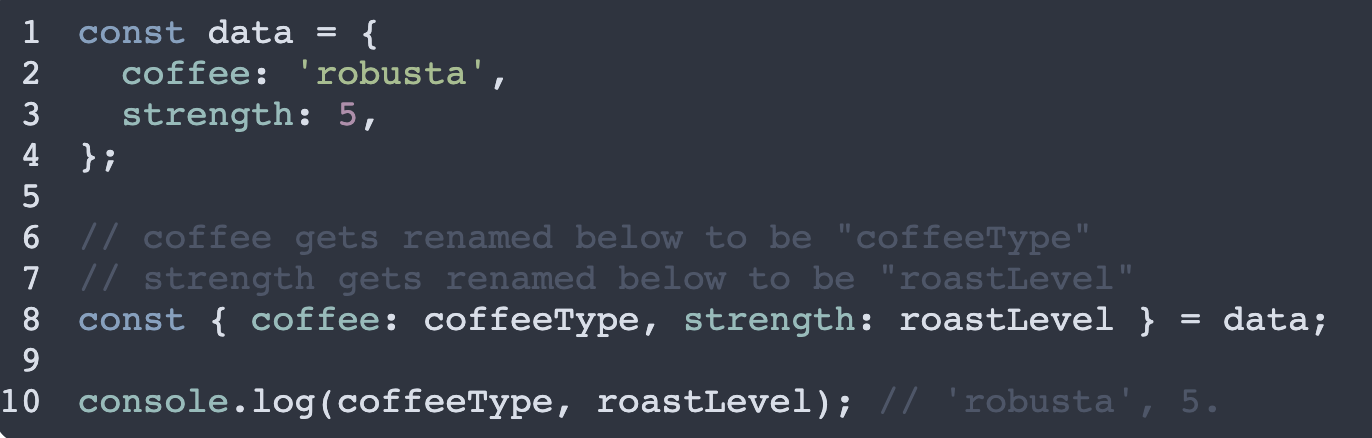