In this post we cover findIndex vs indexOf in JavaScript
findIndex vs indexOf in JavaScript
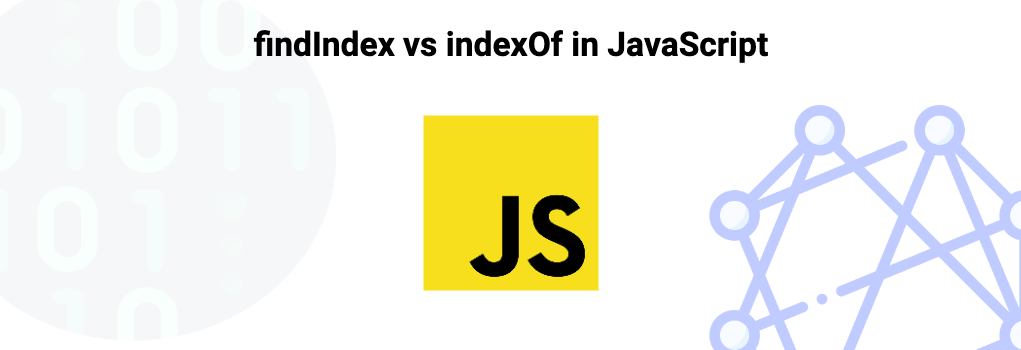
The primary difference between Array.prototype.findIndex and Array.prototype.indexOf in JavaScript is that indexOf accepts a number as an argument whereas findIndex accepts a callback.
Aside from that both are methods to find the index of a value in a given array.
By passing the value directly into indexOf
it will return the index of that value when it finds it.
By passing a callback into findIndex
it provides a way to find the index of a value in an array for more complex use cases.
Here are some examples:
1const someArray = ["a", "b", "c"];
2
3// when the value is found:
4someArray.indexOf("b"); // 1
5someArray.findIndex(item => item === "b"); // 1
6
7// when the value is not found:
8someArray.indexOf("d"); // -1
9someArray.findIndex(item => item === "d"); // -1
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
VanillaJS
VanillaJS is a term often used to describe plain JavaScript without any additions, libraries or frameworks.
In other words when writing with VanilliaJS you are simply writing JavaScript as it comes out of the box.
Array.prototype.indexOf
Array.prototype.indexOf is a method that can be used to find the index of a given value within an array.
If successful the method will return the index, if not then the method will return -1.
The method indexOf accepts a value as an argument. For more complex cases, such as finding an object, <a href="/definition/array.prototype.findindex/">Array.prototype.findIndex</a>
might be needed.
Array.prototype.findIndex
Array.prototype.findIndex is a method used to find the index of a value within an array.
It accepts a callback as an argument where the callback must return a boolean value, true
if the value is the one being searched for, or false
if not.
If the value is found, then the method will return the index, otherwise it will return -1
to indicate that the value was not found.
The callback will be provided with each item in the array until the callback returns true.
This method works well for more complex use cases such as searching for objects within an array, but for simpler cases Array.prototype.indexOf might be another good option.
Further resources
Related topics
Save code?
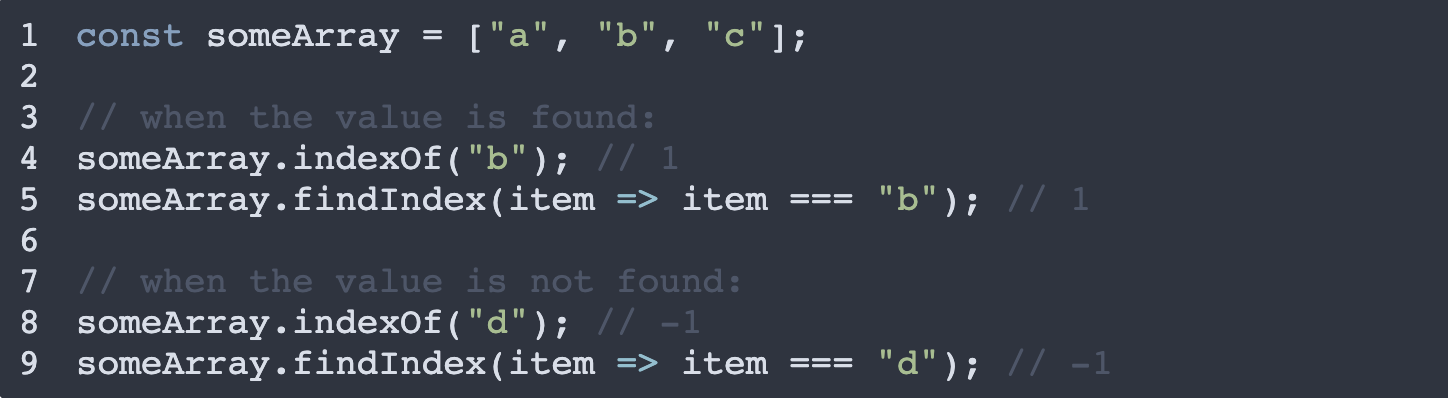