In this post we cover how to continue in a JavaScript forEach loop.
How to continue in a JavaScript forEach loop
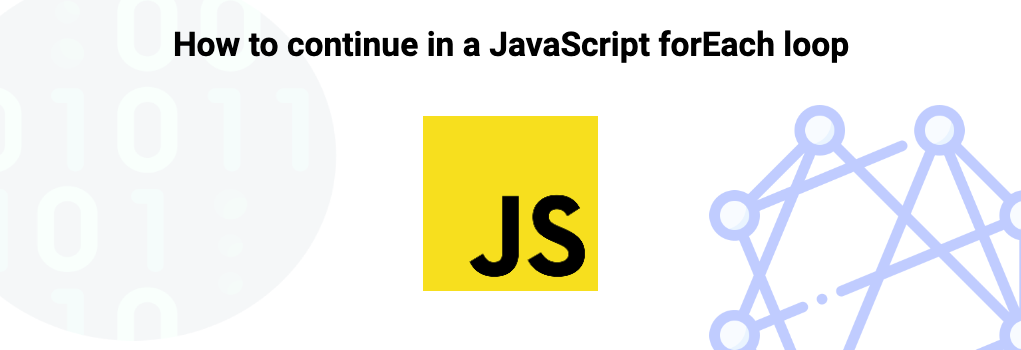
In the Array.prototype.forEach method you are unable to use the continue statement because the Array.forEach method requires you to create a function to be passed in as a callback and the continue statement is only valid within the scope of a loop.
Attempting to will likely provide you with the following error: "Uncaught SyntaxError: Illegal continue statement: no surrounding iteration statement"
Instead, the equivalent is using a return statement. So to continue in a JavaScript forEach loop you need to use a return statement instead of a continue statement.
Here is a working example using the return statement:
1const myArray = [10, 18, 40, 53];
2
3myArray.forEach((item) => {
4 if (item > 20) return; // Will skip/continue on 40 and 53.
5 console.log(item); // 10, 18
6});
And here is an example of the error you would get from attempting with a continue statement:
1const myArray = [10, 40, 53];
2
3myArray.forEach((item) => {
4 if (item > 20) continue;
5 console.log(item);
6});
7
8// Uncaught SyntaxError: Illegal continue statement: no surrounding iteration statement
In summary, to continue in a JavaScript forEach loop you need to use the return statement instead of the continue statement.
Array.prototype.forEach
Array.prototype.forEach or Array.forEach is a method on a Javascript array that will allow you to use a callback function to loop over each item in the array.
It is essentially syntactic sugar for a for loop with a given array, although it is not an exact replacement for a for loop because of things like async code.
The callback will be called for each iteration of the loop with each item passed in as an argument of the callback.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Further resources
Related topics
Save code?
