In this post we cover what is hydration in React based applications
What is hydration in React based applications?
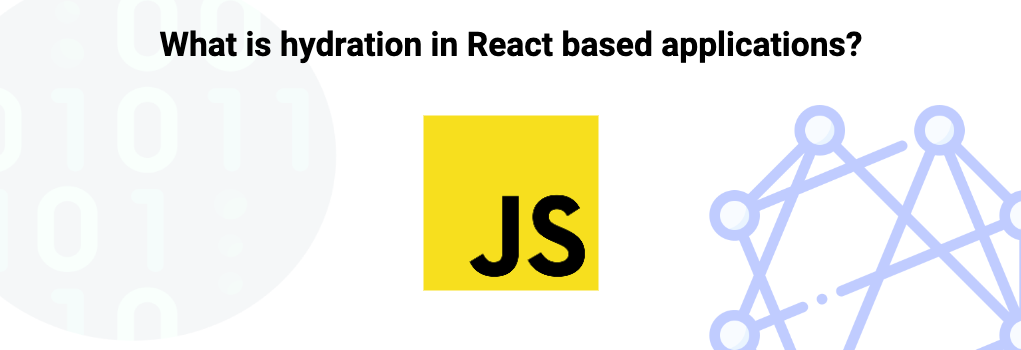
Hydration in React based applications or similar libraries or frameworks is a concept that allows the best of server side rendering as well as dynamically served or generated on the client side from JavaScript.
Generally speaking hydration is used when an application is generated at build time to have a skeleton of the application which has benefits such as better load time for users, better SEO and better accessibility.
The skeleton will have all the static HTML and styling possible at build time that will instantly load for the user. Once the JavaScript on the page is downloaded and run it will then be refreshed with anything dynamically loaded by that JavaScript.
For example, in React this means that the skeleton will now be replaced with the React components and all the various events, click handlers api calls and so on that are built in with the JavaScript.
Here is an example:
1// Basic React application with hydration:
2import React, { useEffect } from 'react';
3import ReactDOM from 'react-dom';
4
5const Main = () => {
6 const [coffeeType, setCoffeeType] = useState(null);
7
8 useEffect(() => {
9 (async () => {
10 const name = await getCoffeeName();
11 setCoffeeType(name);
12 })();
13 }, [setCoffeeType]);
14
15 if (coffeeType) {
16 return (<h1>{coffeeType}</h1>);
17 }
18
19 return (<h1>Coffee loading</h1>);
20}
21
22const root = document.querySelector("#root");
23ReactDOM.hydrate(<Main />, root);
1<!-- Skeleton created at build time -->
2
3<html>
4 <head></head>
5 <body>
6 <div id="root">
7 <h1>Coffee loading</h1>
8 </div>
9 </body>
10</html>
Once the page has been loaded and the JavaScript downloaded the getCoffeeName
api call will be triggered and return a value. Then the value is set to the React state and then used as the test in the h1
element.
In short, the result will mean that the application will be hydrated with the new value from the api and result in the following html:
1<!-- hydrated application in real time -->
2
3<html>
4 <head></head>
5 <body>
6 <div id="root">
7 <h1>Arabica Coffee</h1>
8 </div>
9 </body>
10</html>
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Hydration in React
Hydration in React is the process of attaching event handlers and preserving the existing server-rendered HTML content when a React application is initially rendered on the client-side. When rendering a React application on the server, the resulting HTML markup is sent to the client as a static HTML page. To enable interactivity and dynamic behavior, React needs to take over the rendered HTML and attach event handlers, update the DOM, and manage the component lifecycle. This process is known as hydration. During hydration, React reconciles the server-rendered HTML with the client-side JavaScript representation of the components. It matches the existing DOM nodes with their corresponding React components, sets up event listeners, and preserves any state or props passed from the server. By hydrating the server-rendered content, React can seamlessly take over and continue managing the application's interactivity and state updates on the client-side, providing a smooth and interactive user experience.
Further resources
Related topics
Save code?
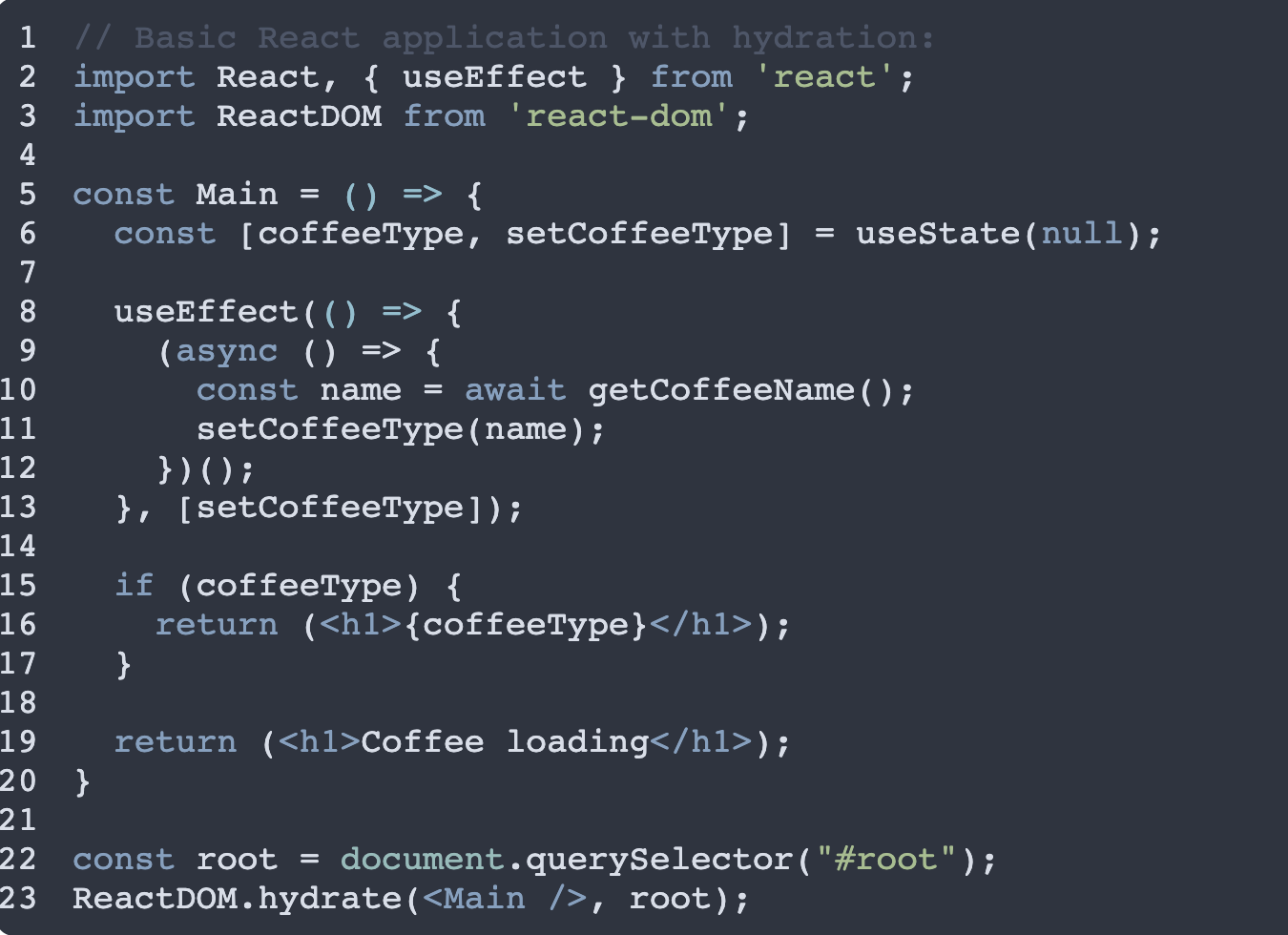