In this post we cover how to pass props to children in React
How to pass props to children in React
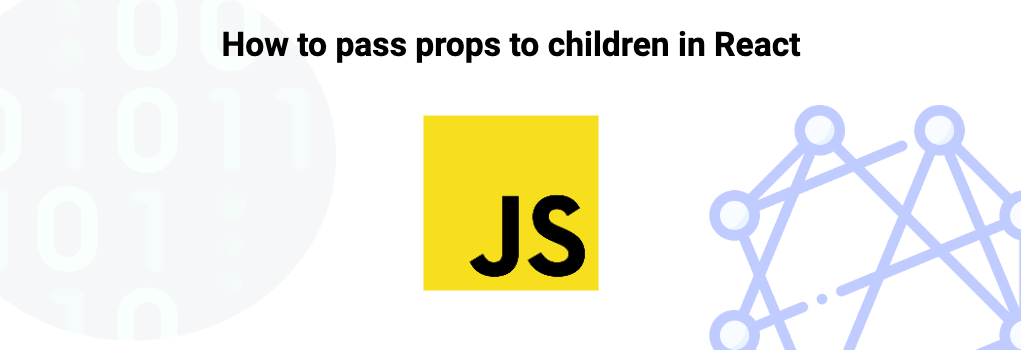
The children
prop in React can be many number of possible items, but the main thing to understand when passing props down is that the prop is that it can contain a single component, or many components.
To get around this in order to pass props into the child components contained within the children prop, both cases must be accounted for.
React does provide a few methods to help with this.
Here is how they can be used to pass props into the components within the children property:
1function addPropsToElement(element, props) {
2 if (React.isValidElement(element)) {
3 return React.cloneElement(element, props);
4 }
5
6 return element;
7}
8
9function addPropsToChildren(children, props) {
10 if (!Array.isArray(children)) {
11 return addPropsToElement(children, props);
12 }
13
14 return children.map(childElement =>
15 addPropsToReactElement(childElement, props)
16 );
17}
The above code has a few main features.
- The first check finds out if the children prop contains one or many elements by checking if it is an array.
- The next check is to ensure that the child in question is a valid React element meaning that props can be applied to it if it is.
- Lastly, if the element is valid, then a copy of the element is made with the newly attached props.
If any of these checks fail then the default element is returned. If the checks pass then the element will now have the attached props.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Further resources
Related topics
Save code?
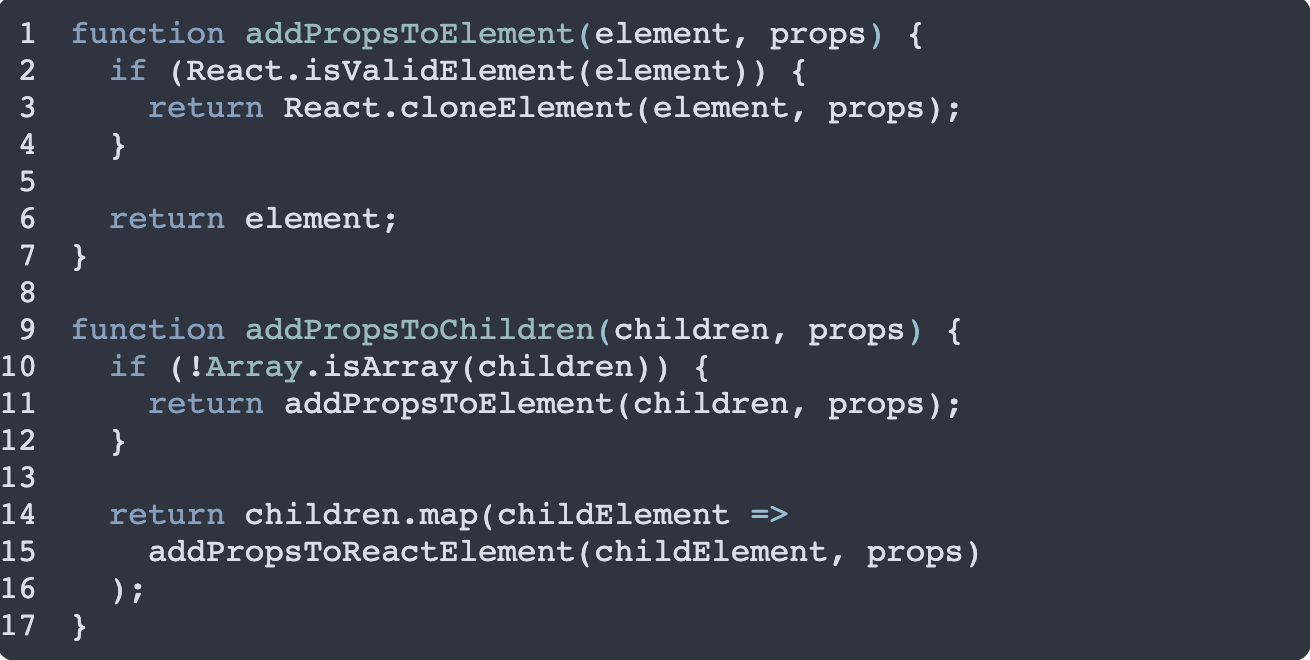