In this post we cover how to use apollo client without hooks (useQuery, and useMutation)
How to use apollo client without hooks (useQuery, and useMutation)
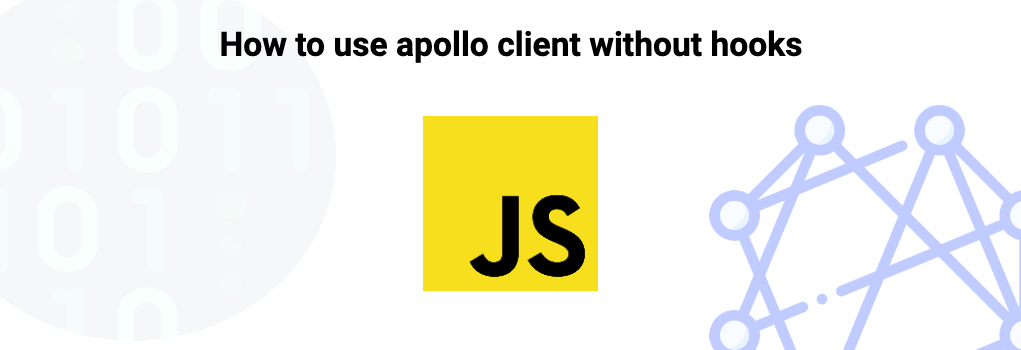
When using apollo client in a ReactJS application to make GraphQL network requests a common way to do this is to use the built in React hooks, useQuery and useMutation.
However, the use of these React hooks doesn't cover all scenarios such as redux middleware's such as sagas or general functional requests.
Fortunately it requires no additional code to be able to make functional network requests using Apollo client in React because you are still using the same client.
Here is an example of a standard Apollo Client setup in ReactJS:
1import React from "react";
2import { ApolloClient, InMemoryCache, ApolloProvider } from "@apollo/client";
3import App from "./App";
4
5export const apolloClient = new ApolloClient({
6 uri: "https://softwareshorts.com/api/",
7 cache: new InMemoryCache(),
8});
9
10export default function Root() {
11 return (
12 <ApolloProvider client={apolloClient}>
13 <MyApp />
14 </ApolloProvider>
15 );
16}
Here the client is passed into the ApolloProvider component which uses a context to allow the normal useQuery and useMutation hooks to run queries and mutations based on props, renders and state changes.
The key element though is that the Apollo client is still having to be provided which is called apolloClient
in the above example.
To make use of this outside of the ApolloProvider component, the client just needs to be imported and then used by calling either .query
or .mutation
on it.
Here is an example of this in use:
1const SoftwareShortsQuery = gql`
2 query SoftwareShortsQuery($software: String!) {
3 getSoftware(software: $software) {
4 name
5 data
6 }
7 }
8`;
9
10
11const response = await apolloClient.query({
12 query: SoftwareShortsQuery,
13 variables: {
14 software: 'JS',
15 },
16})
This function and request can then be used in and outside of functional components allowing for more flexibility. The same applies for mutations.
Lastly, here is an example of the equivalent request but in hook form:
1const { data, loading, error } = useQuery(
2 SoftwareShortsQuery,
3 {
4 variables: {
5 software: 'JS',
6 },
7 }
8);
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
Functional Component
A functional component in React refers to a normal JavaScript function, that is used as a component and can accept props and return React elements.
This is often used to differentiate between a functional component(ordinary function) and a Class Component in React.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
GraphQL
GraphQL is a query language that provides a number of ways that bring improvements when it comes to accessing data.
There are two sides to GraphQL.
On the frontend you will structure your requests in the shape of a graph laid out in a semi JSON style format where you request fields by naming each one you need in the shape they exist in.
On the backend or server side you create these shapes via a schema and ensure that when requested they are sent in the agreed upon shape.
There are many powerful features, such as resolving with id's, only requesting/sending the data that is requested, patching together various API's and so on.
Apollo Client
Apollo Client is a request library that helps to create requests to a GraphQL server.
Apollo Client also has many additional features that helps to manage the data made in requests.
Apollo Server
Apollo Server is an open source GraphQL server. It enables the use of the GraphQL query language to make requests against the server.
Internally it uses concepts such as data sources, resolvers and schemas.
The schemas are written in GraphQL and show what the server provides and can accept in terms of requests.
Resolvers help to tie requests together to connect one graph entity to another.
Data sources are where the server gets its data from which could be other APIs, databases, and various combinations inbetween.
Further resources
Related topics
Save code?
-code.png)