In this post we cover how to show and hide components and elements in React
How to show and hide components and elements in React
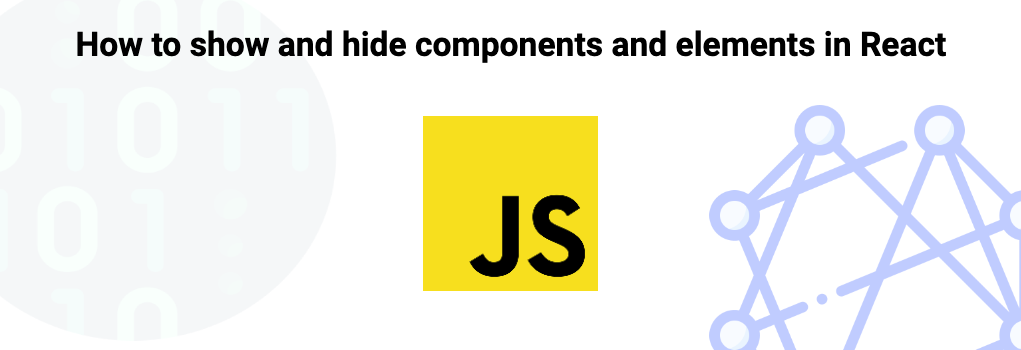
There are a few different ways in which you can show and hide components in React, such as conditional rendering or using css and styles.
Let's look at how we can use conditional rendering in React to show and hide a component.
1import React, { useState } from "react";
2
3export default function MathAnswer() {
4 const [revealAnswer, setRevealAnswer] = useState(false);
5
6 return (
7 <>
8 <h1>What is the answer to 2 + 3?</h1>
9 {revealAnswer && (<p>The answer is 5!</p>)}
10 <button onClick={() => setRevealAnswer(true)}>
11 Reveal Answer
12 </button>
13 </>
14 );
15}
In the above code, we create some logic to show and hide a component using conditional rendering.
The component starts by hiding the element/component from the user which contains the answer to a math problem.
It is hidden by a condition that needs to equate as truthy that uses a state variable as the comparator that we default as false so that the condition fails initially, hiding the component/element.
When the user clicks the "Reveal Answer" button, we set the state variable to be true, which on the next re-render causes the condition to pass and then renders and displays the component/element to the user showing the answer to the question.
Another option is to use css or styles to be able to show/hide a component by toggling the style of class.
You might want to do this if you want to add in a simple transition when showing or hiding the component or if you want to prevent layout shift.
Here is an example of how we can do this:
1.item {
2 z-index: 1;
3 position: relative;
4 height: 100px;
5 width: 100px;
6 display: block;
7 background: rgba(0, 0, 0, 0.5);
8 transition: 0.5s all;
9 opacity: 1;
10 pointer-events: auto;
11}
12
13.hide {
14 opacity: 0;
15 pointer-events: none;
16 z-index: -1;
17}
1import React, { useState } from "react";
2
3export default function ShowHideExample() {
4 const [hideItem, setHideItem] = useState(false);
5
6 return (
7 <>
8 <button onClick={() => setHideItem(true)}>
9 Hide Item
10 </button>
11 <div className={`item ${hideItem ? "hide" : ""}`} />
12 </>
13 );
14}
In the above example, we are using a state variable to hide an item in our JSX by conditionally rendering a css class rather than the component. So, when the button is clicked we render the ".hide"
class which applies styles that will hide the item from the user.
To summarise, to show and hide components and elements in React, you will need to use conditional rendering in your React application. But you can use it in a number of ways to provide a number of different outcomes.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
Conditional rendering
Conditional rendering is a technique used in React to either show or hide a component by rendering it or not rendering it.
Conditional rendering is done by applying a condition to a given component and then if the condition passes then the component will be rendered and the user will see the rendered component. If the condition fails then the component will not be rendered and the user will not see it.
Layout shift
Cumulative Layout shift, or Layout shift for short, is where an element on a page or screen is added or removed which then causes the other elements on the page to shift and move places.
For example if an element is removed from the top of a page then the elements below will have to shift upwards. If an element is added to the top of the page then the existing elements on the page will have to shift down.
Generally, layout shift is something to avoid because it can create a poor user experience. To reduce CLS you can limit the amount of elements that dynamically added or removed from a page, as well as making use of placeholders to keep the positions of the elements on the page intact.
State variable
A state variable is a variable that you can get and set by making use of a React state function to obtain essentially a getter and setter for this variable.
A state variable will persist its value between renders and when the value of it changes, it will trigger a re-render.
An example of this would be using the React hook "useState" which returns an array where the first item is the state variable and the second item is a setter for the state variable.
Further resources
Related topics
Save code?
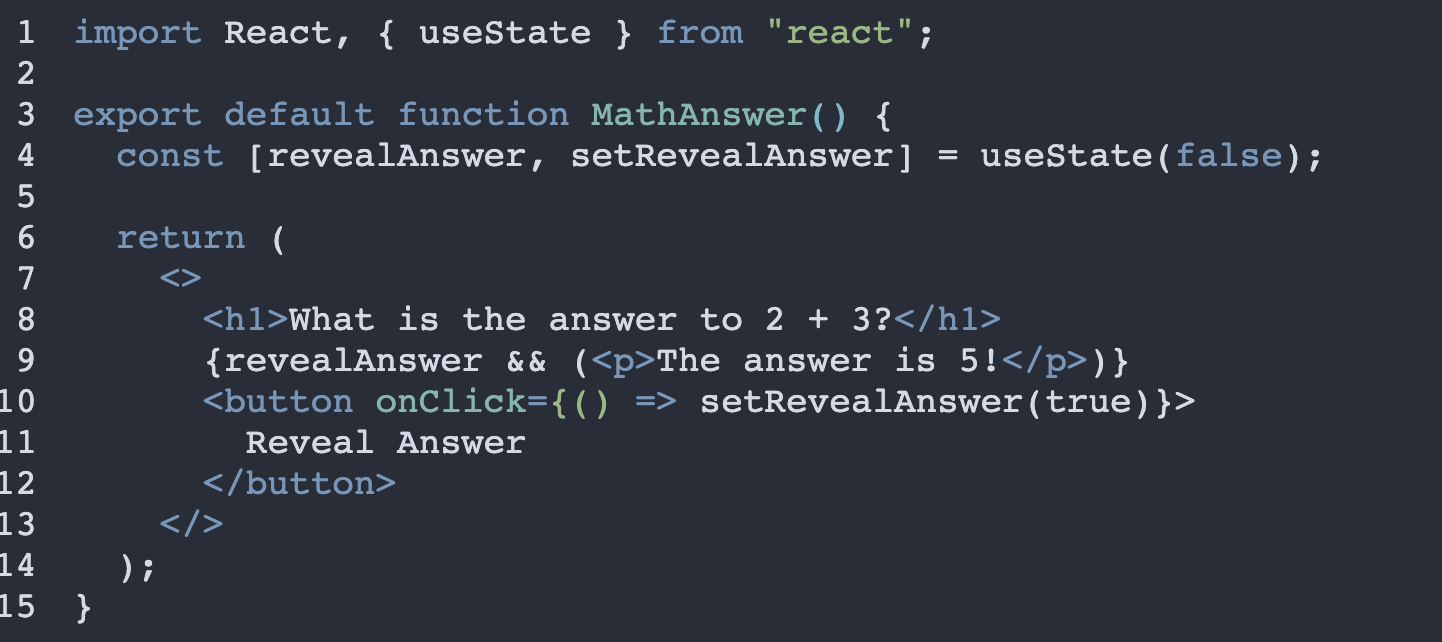