Converting arrays into JSX is a fundamental part of using React. In this post we learn how to render an array of components with Array.prototype.map in React.
How to render an array of components in React
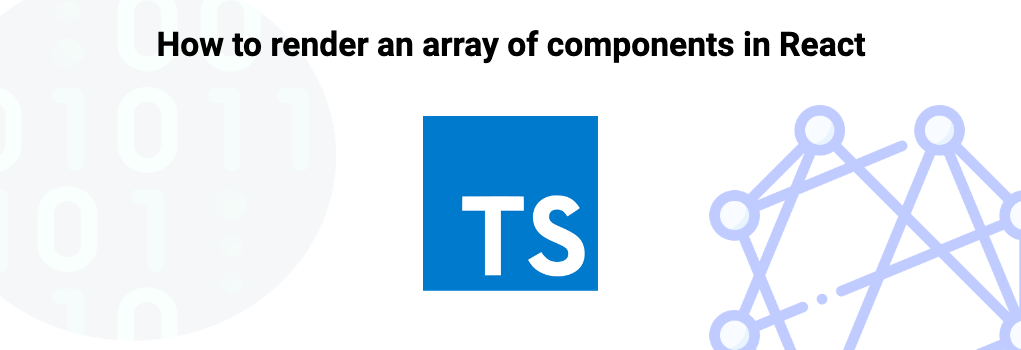
1const snowDaysArray = [
2 <p key="1">It snowed 4 inches on 25/12/2022.</p>,
3 <p key="2">It snowed 2 inches on 24/12/2022.</p>,
4];
5
6export default function Snowdays() {
7 return (
8 <>
9 {snowDaysArray}
10 </>
11 );
12}
Generally, you won't have an array of components like this though. Instead you will have an array of data that needs converting into an array of components that can be rendered by React.
You can convert the array of data into an array of components by using the Array.prototype.map method (Or Array.map for short).
Here is an example of how to create an array of components from an array of data using Array.prototype.map in React:
1const snowDaysArray = [
2 {inches: 4, date: '25/12/2022', id: '1'},
3 {inches: 2, date: '24/12/2022', id: '2'},
4];
5
6export default function Snowdays() {
7 return (
8 <>
9 {snowDaysArray.map(({ inches, date, id }) => (
10 <p key={id}>It snowed {inches} inches on {date}.</p>
11 ))}
12 </>
13 );
14}
To summarise, to render an array of components in React, you need to pass the array of components into JSX to be rendered.
If you don't have the array of components and instead have an array of data then you need to first convert it into an array of components by using the Array.prototype.map method.
Read more about this here: How to render an array of objects with Array.map in React.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
React Key
A React key is something used to track items in an array that gets rendered in React. It helps to keep track of which item is which between renders given that the array items can change.
To prevent issues React asks you to pass in a unique key for each item in the array so if the array changes React knows how to handle it.
Array.prototype.map
Array.prototype.map or Array.map for short is a method on Javascript arrays that allows you to map/convert the array items to something else and does not mutate.
It accepts a function/callback that is run for each iteration with iterations array item, and the return value from that function will become the new item in the new array. Once complete it will return the new array containing the mapped/converted items so that you can store it as a variable.
For example, given an array of numbers, if you wanted to multiply each by 10 then you could call map on your array and pass in a function that accepts an item which will be a number, and then returns a value that uses the multiplication operator on the item to multiply it by 10.
This function would then be run for each item in the array multiplying each by 10, once the whole array has been run the map function will return the new multiplied array ready to be used or stored.
Further resources
Related topics
Save code?
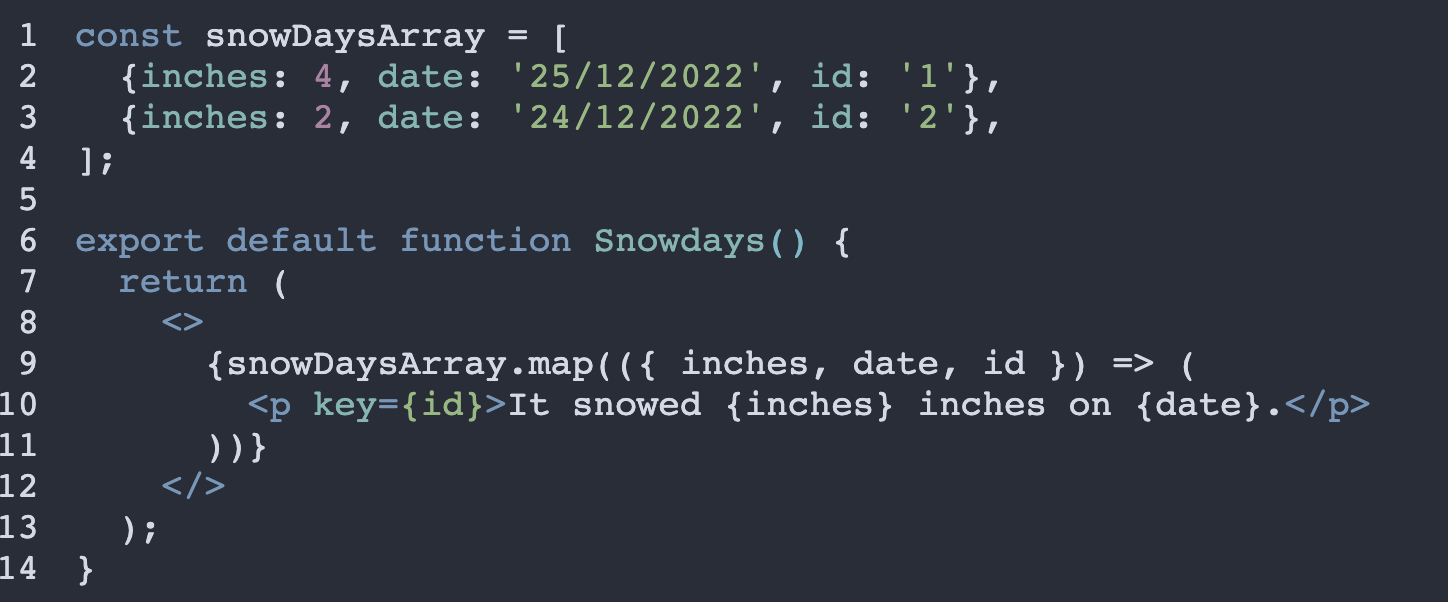