In this post we cover what are the differences between React.Fragment vs <>
What are the differences between React.Fragment vs <>
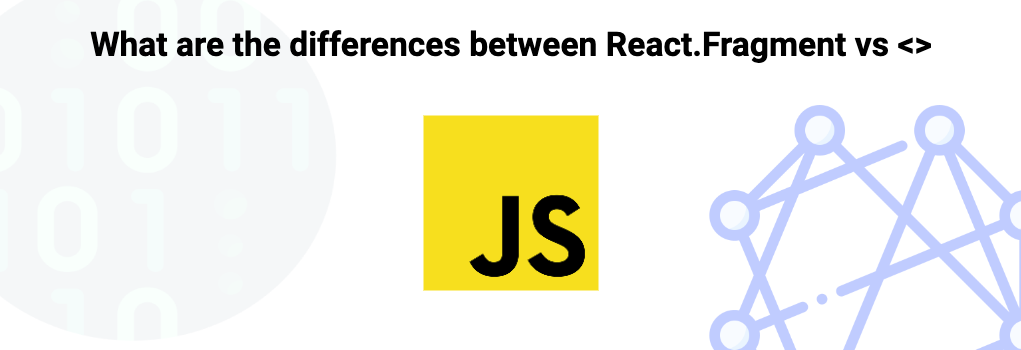
The main difference between React.Fragment vs <> is that one is the longhand version and the other is the short hand version.
The short hand version is typically used more frequently in newer applications due to it being easier to use, and write.
The only other difference is that the longhand version (React.Fragment) supports attributes so that a React key can be assigned when grouping elements or components in an array.
1import React from 'react';
2
3// shorthand
4export function CoffeeListShort() {
5 return (
6 <>
7 <p> Robusta </p>
8 <p> Arabica </p>
9 </>
10 );
11}
12
13// longhand
14export function CoffeeListLong() {
15 return (
16 <React.Fragment>
17 <p> Robusta </p>
18 <p> Arabica </p>
19 </React.Fragment>
20 );
21}
22
23// array
24
25export function CoffeeListLong() {
26 const coffeeList = ['Robusta', 'Arabica'];
27
28 return (
29 <>
30 <h3> Coffee List </h3>
31 {coffeeList.map(item => (
32 <React.Fragment key={item}>
33 <p> Coffee: </p>
34 <p>{item}</p>
35 </React.Fragment>
36 ))}
37 </>
38 );
39}
40
React Key
A React key is something used to track items in an array that gets rendered in React. It helps to keep track of which item is which between renders given that the array items can change.
To prevent issues React asks you to pass in a unique key for each item in the array so if the array changes React knows how to handle it.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React.Fragment
React.Fragment is a built-in component in React that allows you to group multiple elements without introducing an additional wrapping element. It provides a way to return multiple elements from a component's render method without needing to wrap them in a parent element. When rendering JSX code, you typically need to enclose multiple elements within a single parent element. However, there are scenarios where introducing an extra parent element may interfere with the desired layout or structure of the component. React.Fragment solves this problem by providing a lightweight wrapper that doesn't create any additional nodes in the rendered HTML. It allows you to group elements together while maintaining a clean and concise JSX syntax. You can use React.Fragment by either using the <<a href="/definition/react.fragment/">React.Fragment</a>>
syntax or the shorthand syntax <>
. Using React.Fragment helps in avoiding unnecessary DOM nodes and ensures the structure and semantics of your components are not compromised.
Further resources
Related topics
Save code?
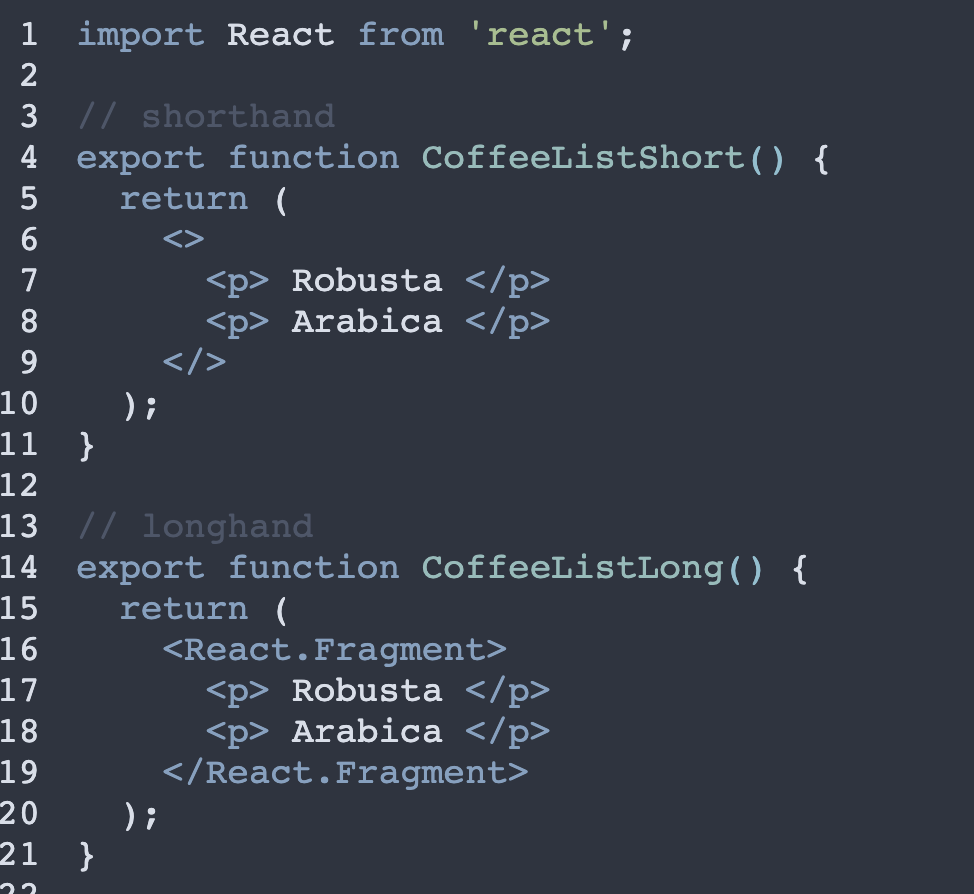