In this post we cover how to make and use a Google autocomplete react hook
How to make and use a Google autocomplete react hook
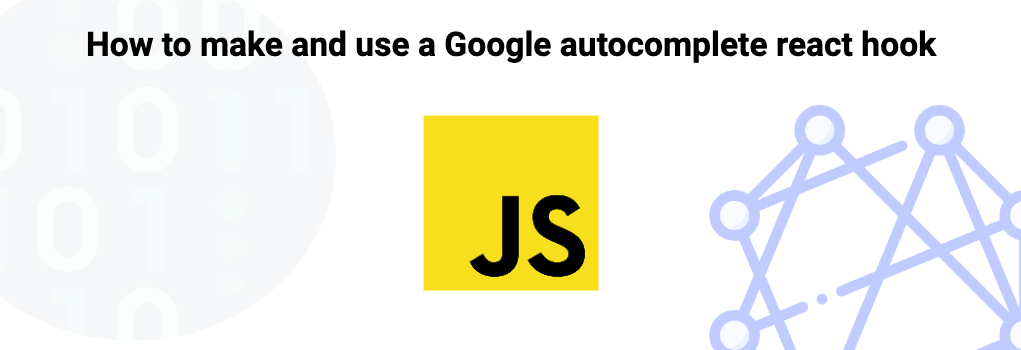
To get autocomplete data from the Google Places API an API Key will be needed which can be obtained here.
Afterwards, the API will need to be loaded or imported into your app. Here is a universal option using a script tag in the page head element:
1<script
2 type="text/javascript"
3 src="//maps.googleapis.com/maps/api/js?key=API_KEY&language=en&libraries=places"
4></script>
Once imported the autocomplete api can be accessed via google.maps.places.AutocompleteService().getPlacePredictions(options, callback)
.
To make use of this method, a helper function can be created to make it easier to use in the app and to convert the promise into an async/await function:
1export const fetchAutocompleteSuggestions = async (input) =>
2 new Promise((resolve, reject) => {
3 if (!input) {
4 return reject("Input is empty");
5 }
6
7 try {
8 new window
9 .google
10 .maps
11 .places
12 .AutocompleteService()
13 .getPlacePredictions({
14 input,
15 componentRestrictions: {
16 country: "gb"
17 },
18 },
19 resolve,
20 );
21 } catch (e) {
22 reject(e);
23 }
24 });
The above code demonstrates a simple helper method that accepts an input of text, passes it into the getPlacePredictions
method and uses a promise to resolve the result once the API returns the data.
To use a different country, a different ISO 3166-1 Alpha-2 country code will need to be provided.
The next thing to do is to use this code in a react application. Whilst there are many options and different ways in which the above code can be used, a react hook will likely prove the easiest/simplest way.
The hook that needs to be created needs to be able to debounce the user input to avoid spamming the API, make the request to the API, and then return the resolved data.
Here is the hook containing all of the above:
1export function usePlacesAutocomplete(userInput = "") {
2 const [suggestions, setSuggestions] = useState([]);
3
4 useEffect(() => {
5 const debounceTimeout = setTimeout(async () => {
6 try {
7 if (!userInput) return;
8
9 const nextSuggestions =
10 await fetchAutocompleteSuggestions(userInput);
11
12 setSuggestions(nextSuggestions);
13 } catch (e) {
14 console.error(e);
15 }
16 }, 400);
17
18 return () => {
19 clearTimeout(debounceTimeout);
20 }
21 }, [userInput, debounceTimeout]);
22
23 return suggestions;
24}
With the above hook, when the user enters some text, once the debounce period has finished (meaning the user has paused whilst typing) then the request will be made to the API and once completed set to the state contained within the hook ready to be accessed in the React application.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
Google Places API
The Google Places API is a HTTP service that can be accessed via network requests and offers location data such as establishments, geographic locations, or prominent points of interest.
Debouncing
Debouncing is a concept where unwanted or extra noise is filtered out and removed from various actions.
A good example is user input searches. When a user is searching for something and you are reacting to the input they are providing, if you made a request for every character the user typed then that could be very costly, and slow the application whilst providing little to no benefit to the user.
Instead if you wait for the user to pause or stop typing before making the request with their input then the number of requests made will be significantly less.
Further resources
Related topics
Save code?
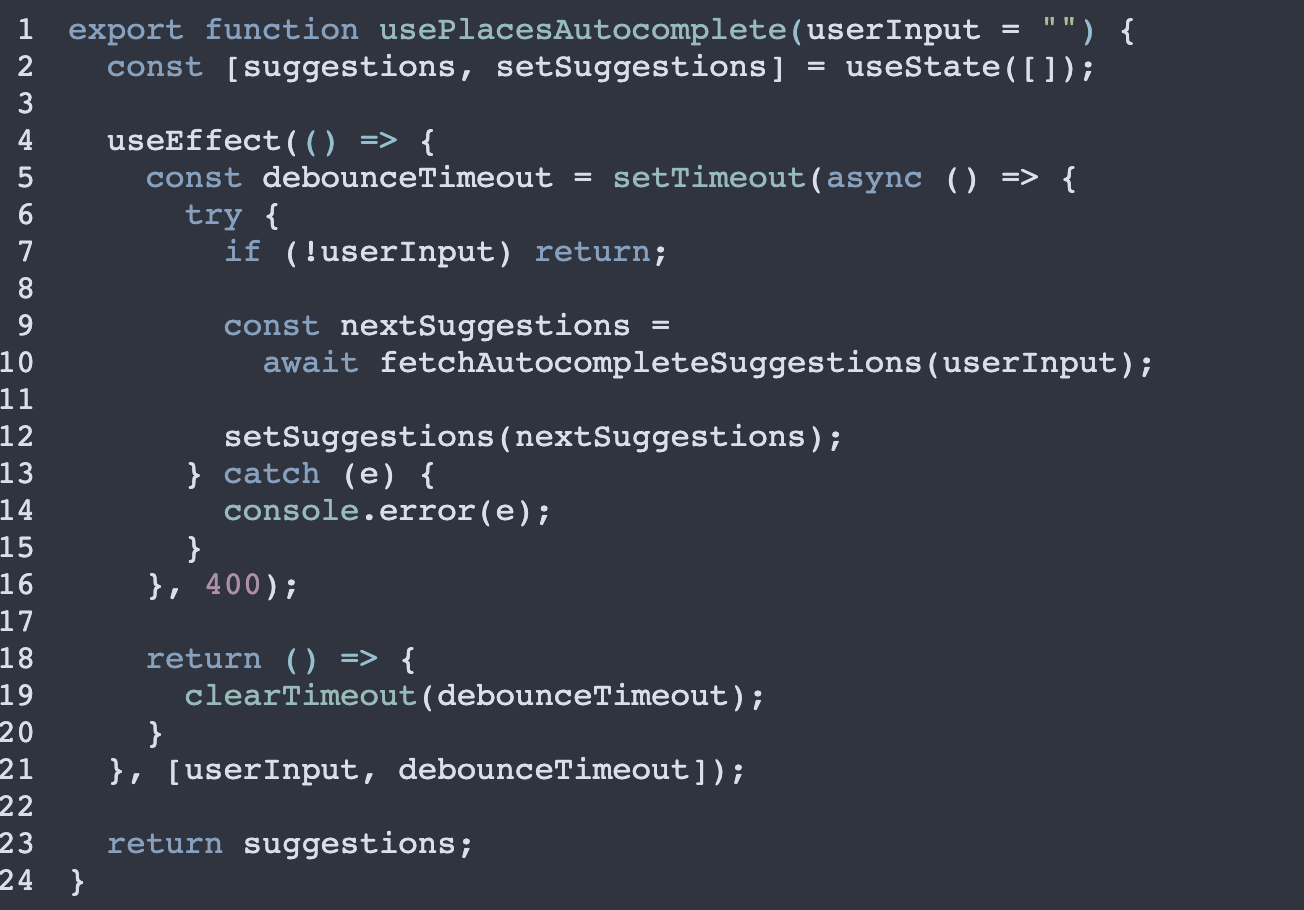