In this post we cover when to use useImperativeHandle
When to use useImperativeHandle
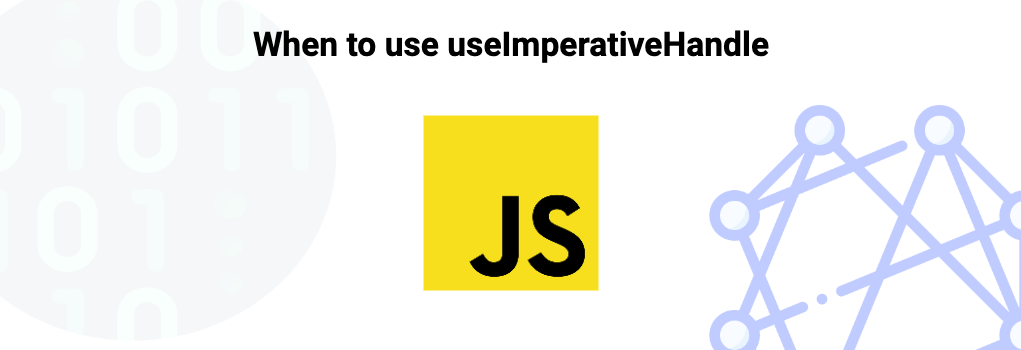
In React references can be created to help control or get data about elements. The react hook useImperativeHandle enables modification to refs in order to allow a child component to selectively expose some of it's API to a parent element.
When a ref is forwarded to a child component, by using useImperativeHandle the ref can be modified in the child component.
Here is an example:
1import React, { useRef, useImperativeHandle, forwardRef } from 'react';
2
3// Child component
4const ChildComponent = forwardRef((props, ref) => {
5 const internalStateRef = useRef(null);
6
7 // Expose only the increment function to the parent component
8 useImperativeHandle(ref, () => ({
9 increment: () => {
10 internalStateRef.current++;
11 }
12 }));
13
14 return <div>Child Component</div>;
15});
16
17// Parent component
18const ParentComponent = () => {
19 const childRef = useRef(null);
20
21 const handleButtonClick = () => {
22 childRef.current.increment(); // Invoke the exposed increment function from the child component
23 };
24
25 return (
26 <div>
27 <ChildComponent ref={childRef} />
28 <button onClick={handleButtonClick}>Increment Child Counter</button>
29 </div>
30 );
31};
useRef
The useRef hook creates a reference as a variable which can be used for a number of things in a React application.
This reference is mutable, unlike stateful variables.
The most common use case is to assign DOM elements to them so you can view and modify them outside of the standard React lifecycle.
However, any value can be assigned to a ref, such as a string, an object, a number and so on.
At its core, a ref is a variable that get's persisted between renders and does not cause any renders of its own.
You can access the value by getting the current field on the ref.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React Ref
In React, a ref is an object that provides a way to reference and interact with individual React components or DOM elements directly. It serves as a means to access or modify properties, call methods, or retrieve information from the underlying elements or instances. Refs are particularly useful when you need to perform imperative actions, manage focus, handle animations, or access DOM-related functionality that goes beyond the typical data flow of React's component-based architecture. By using refs, you can bridge the gap between the declarative nature of React and the need for more imperative operations, providing greater control and flexibility in certain scenarios. It's important to note that refs should be used with caution and only when necessary, as they introduce a level of complexity and can make the code less declarative and harder to reason about.
Further resources
Related topics
Save code?
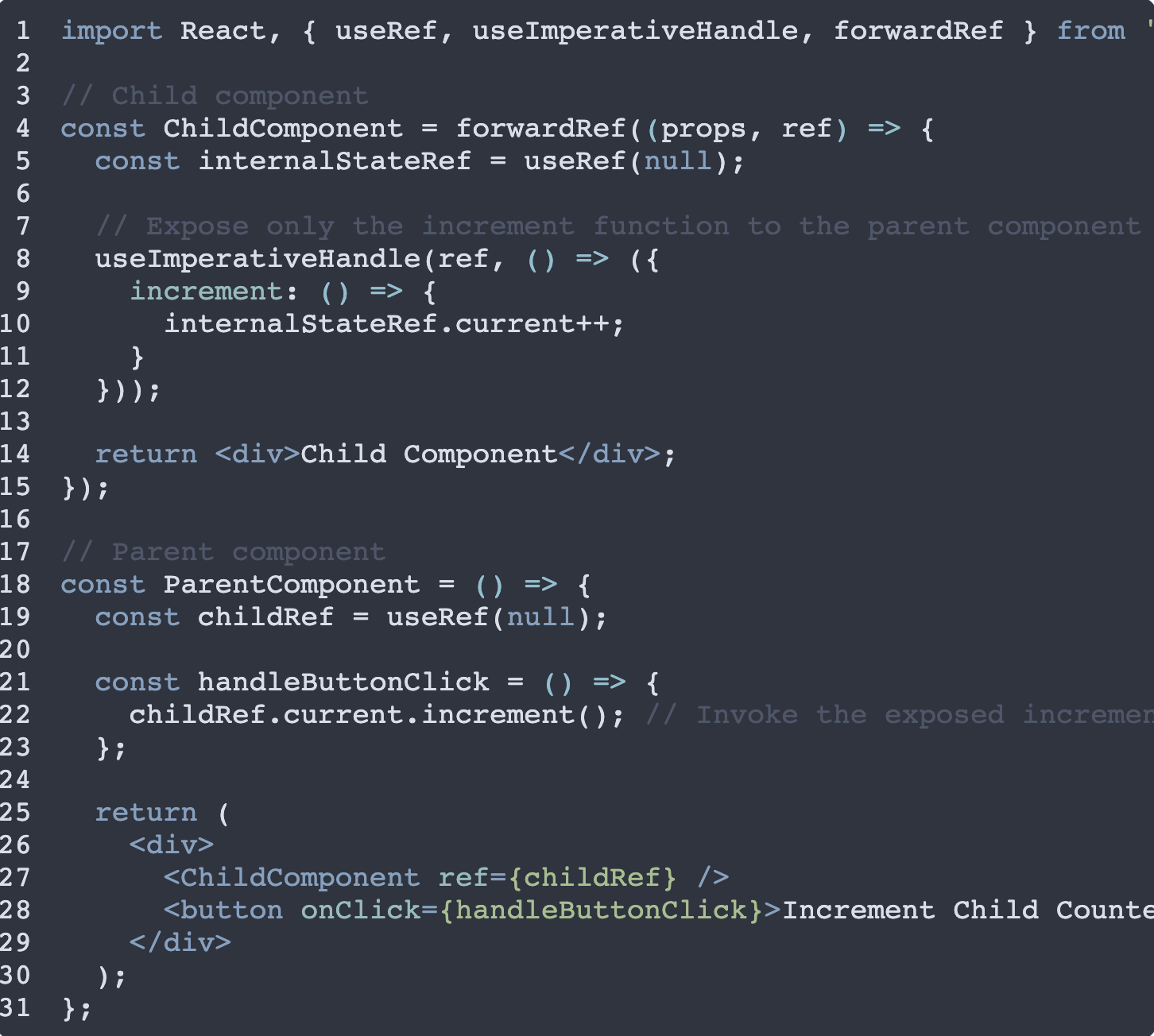