React.memo vs useMemo
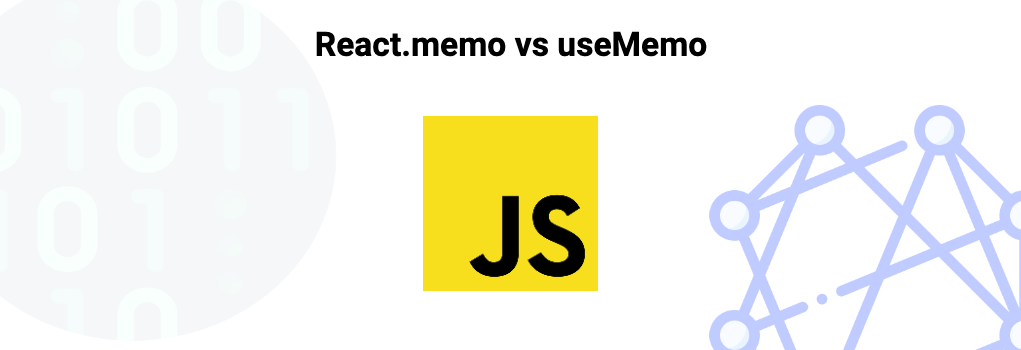
React.memo and useMemo are two functions that can be used in React to provide memoization between renders within your React application.
useMemo is a hook that will memoize or remember the values stored within it until one of the dependancies change.
React.memo provides memoization to entire components by only allowing re-renders based on certain prop changes that is configurable.
There are a number of reasons why memoization might be needed, the main ones are to avoid excessive processing or to avoid unnecessary re-renders of components.
Here is useMemo in use:
1function processNumbers(a, b) {
2 return a * b;
3}
4
5export default function NumberComponent({ numberA, numberB }) {
6 const result = useMemo(
7 () => processNumbers(numberA, numberB),
8 [numberA, numberB],
9 );
10
11 return (<p> {result} </p>);
12}
In the above example the result is the value returned from the processNumbers
function. Any time the props numberA
or numberB
change the useMemo hook will process the values with the new prop values because of the dependancy array. Anything passed into the dependancy array will cause the useMemo function to re evaluate the value/result.
1function processNumbers(a, b) {
2 return a * b;
3}
4
5function NumberComponent({ numberA, numberB }) {
6 return (<p> {processNumbers(numberA, numberB)} </p>);
7}
8
9export default React.memo(NumberComponent, function areEqual(
10 prevProps,
11 nextProps
12) {
13 if (prevProps.numberA !== nextProps.numberA) {
14 // if false is returned then the props are different
15 // and React knows this component needs re-rendering.
16 return false;
17 }
18 if (prevProps.numberB !== nextProps.numberB) {
19 // if false is returned then the props are different
20 // and React knows this component needs re-rendering.
21 return false;
22 }
23
24 // if true that means the previous props are equal to
25 // the current props and so React knows that this component
26 // does not need to be re-rendered.
27 return true;
28})
When being used for performance optimisations, it is important to remember that the processing of memoization often can outweigh the benefits unless the processing is very heavy on the values being memoized.
useMemo
useMemo is a React hook that uses memoization to store/cache/remember a value returned from the function provided to it.
The memoization happens by providing a dependency array, as long as all the values in the dependency array remain the same then the hook will return the stored value for that input set.
When they change, it will process and store the new value for memoization.
This is slightly different to useCallback because useCallback will remember the function passed in whereas useMemo will remember the value returned from it.
In React this comes in handy to prevent extra renders, prevent expensive computations, or to prevent duplicate network calls.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Memoization
Memoization is a term in software development that refers to when a system essentially remembers something. It is a performance optimization where the system will store or cache something and return that value any time it is provided with the same input.
For example an add function given 1, and 1 as parameters will process the parameters by adding them together. A non-memoized version of this function will run that process again and again even if the parameters are the same. Whereas a memoized function will remember the result of 1, and 1 and just return that value rather than processing again.
Further resources
Related topics
Save code?
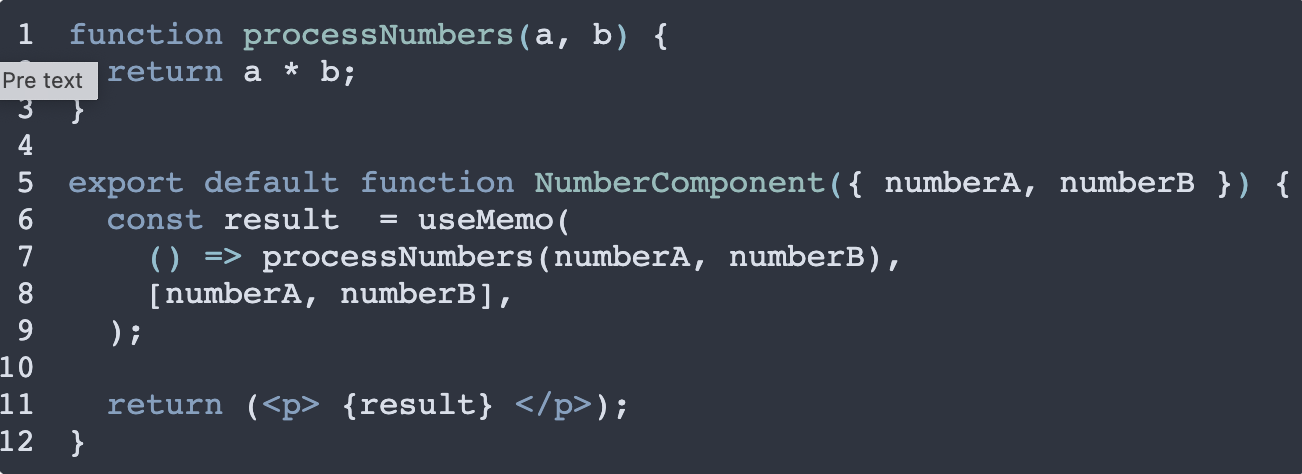