In this post we cover how to use css variables with React
How to use css variables with React

To use css variables in React is not much different than using css variables in any other frontend application because it is handled within the css and not JavaScript.
The only thing that we need to do is ensure that the css variables have been defined, and are loaded onto the page.
After that you can make use of the css variables in any inline style or css style sheet on the page.
1/* variables.css */
2body {
3 --text-color: #000;
4}
1/* textStyles.css */
2.paragraph {
3 color: var(--text-color);
4}
In the above snippets of code you can see that we define the css variable on the body by starting with 2 dashes followed by the name we give the variable and lastly, the value itself.
Later on we make use of this variable in a ".paragraph" selector by applying the variable with var(...)
which will apply the value of the variable to the "color" property.
To make use of this in React you will need to import your variables before you can use them.
1// root component
2import './theme/variables.css';
3
4export default function App () {
5 return (<Text>Hello World</Text>
6}
1// text component
2import './textStyles.css';
3
4export default function Text ({ copy }) {
5 return (<p className="paragraph"> {copy} </p>);
6}
The above code snippets show a fairly standard way in which you can make use of css variables within React.
Css variables can be created and consumed in the same file, or across many. The only requirement is that before you can use a css variable one must be created and imported into a page. To then use the variable in React, you can do so by either applying the class with the variable or by applying the inline-styles directly to your components.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
CSS Variables
CSS variables are something that can be used to share style values across many properties within CSS in a frontend application.
They are defined with a double dash followed by a name and lastly the value. To invoke them you just need to pass them into a var()
.
The only other consideration is that in order to use a variable it must have been defined and remain accessible to anywhere you are using them.
For example if you add them to the body tag of the page all selectors will be able to access them.
Further resources
Related topics
Save code?
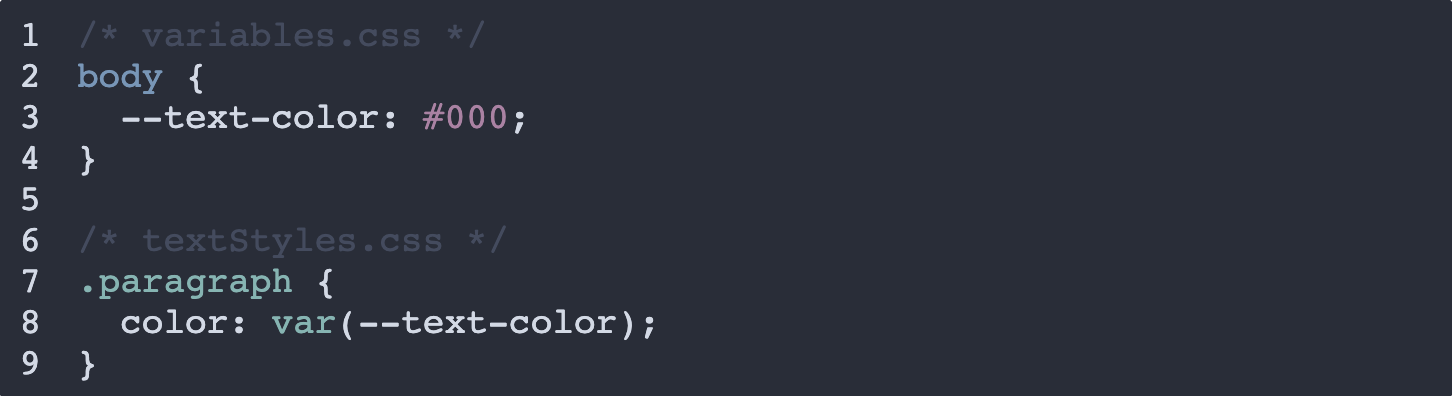