In this post we cover how to use React Router with an optional path parameter.
How to use React Router with an optional path parameter
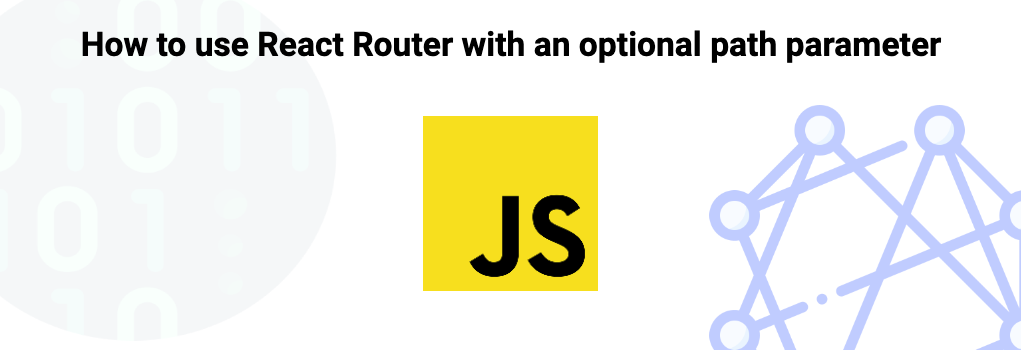
To use React Router with an optional path parameter, all you need to do is to append the path parameter with a ?
question mark character, like so:
1<BrowserRouter>
2 <Switch>
3 <Route
4 path="/hello-world/:name?"
5 component={HelloWorldComponent}
6 />
7 </Switch>
8</BrowserRouter>
Another option is to use URL get parameters instead which gives a little more versatility and is natively supported in JavaScript.
To do the same as above with a GET parameter, it can be done like so:
Firstly, we would define the React Router path without the optional parameter like so:
1<BrowserRouter>
2 <Switch>
3 <Route
4 path="/hello-world/"
5 component={HelloWorldComponent}
6 />
7 </Switch>
8</BrowserRouter>
Secondly, within the component, (or a helper could be extracted) you would need to get the parameter from the URL like so:
1export default function HelloWorldComponent({ location }) {
2 const name = new URLSearchParams(location.search).get("name");
3 return (
4 <p>Hello, {name || "World"}!</p>
5 );
6}
In summary, you can either append your React Router path with a ?
question mark character, or you can make use of URL GET parameters.
React Router
React Router is a client side router library built for use in React that helps with navigation in SPAs (Single Page Applications).
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Client Side Router
A client side router is a method of navigating and managing an applications routes and URL's from the frontend of an application.
It allows you to write a full application that can use various routes and URLS without ever leaving the page you are on.
This can be a useful technique to avoid loading in new static content by loading it in at the start or lazily rather than requesting the new resources by navigating to a new URL.
One important thing to remember about client side routers is that they do not handle "real" routing with status codes and so on by themselves.
Further resources
Related topics
Save code?
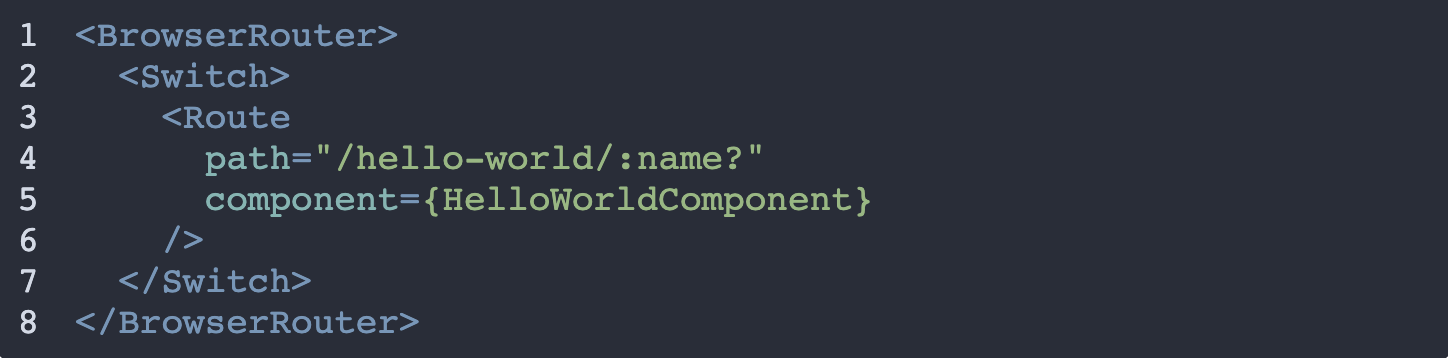