In this post we cover how to sort an array of objects by property value in JavaScript
How to sort an array of objects by property value in JavaScript
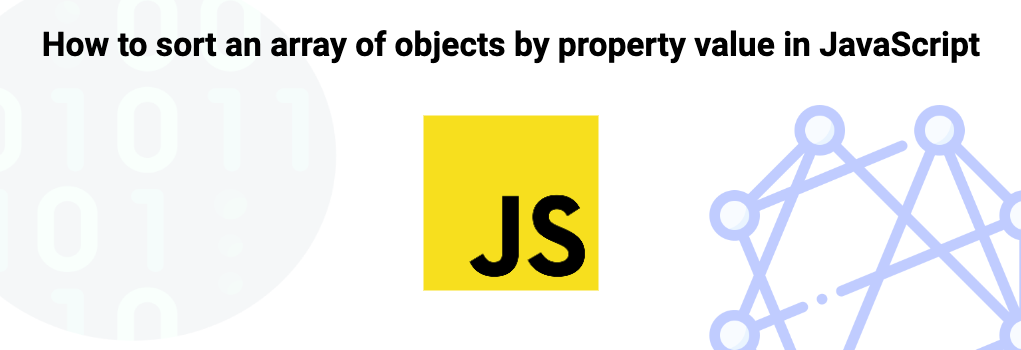
To sort an array of objects by property value in JavaScript the Array.prototype.sort method can be used.
Within the callback method the properties can be used in the conditions used to determine the order.
1const someArray = [{ value: 2 }, { value: 5 }, { value: 3 }];
2
3someArray.sort((a, b) => a.value - b.value);
4// [{ value: 2 }, { value: 3 }, { value: 5 }];
5
6someArray.sort((a, b) => b.value - a.value);
7// [{ value: 5 }, { value: 3 }, { value: 2 }];
To compare multiple/sub properties within an array of objects, the secondary comparison can be done any time that the value 0 would have been returned, but instead more comparisons can be used.
1const coffeeArray = [
2 { price: 3, roast: 5 },
3 { price: 3, roast: 4 },
4 { price: 2, roast: 4 },
5 { price: 2, roast: 1 },
6 { price: 3, roast: 2 },
7];
8
9const subComparison = (a, b) => a.roast - b.roast;
10
11const mainComparison = (a, b) => {
12 const result = a.price - b.price;
13
14 if (result === 0) {
15 return subComparison(a, b);
16 }
17
18 return result;
19};
20
21coffeeArray.sort(mainComparison);
22/*
23[
24 { price: 2, roast: 1 },
25 { price: 2, roast: 4 },
26 { price: 3, roast: 2 },
27 { price: 3, roast: 4 },
28 { price: 3, roast: 5 },
29]
30*/
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Array.prototype.sort
Array.prototype.sort is a method that can be accessed on arrays in JavaScript that can sort the array based on the callback provided.
It uses the "in-place" sorting algorithm.
The callback passed into the method must accept two arguments which will be two different items within the array.
Then within the callback the items can be arranged based on some custom conditions.
The callback must return one of three numbers for each time it is called (for each iteration), 0, greater than 0 or less than 0 (these numbers can be seen as 1, 0 or -1).
This means that in the callback function f(a, b) where a and b are array items:
- Returning 1 will place a after b.
- Returning 0 will leave the order unchanged.
- Returning -1 will place b after a.
When sorting through an array of numbers the same result can be taken by subtracting one number from the other because this will result in the same result.
0 will be for matching numbers, and for greater than 0 results it will be moved to the next item, and less than 0 results will be moved to the previous item.
Inverting the calculation will invert the order of the array.
Further resources
Related topics
Save code?
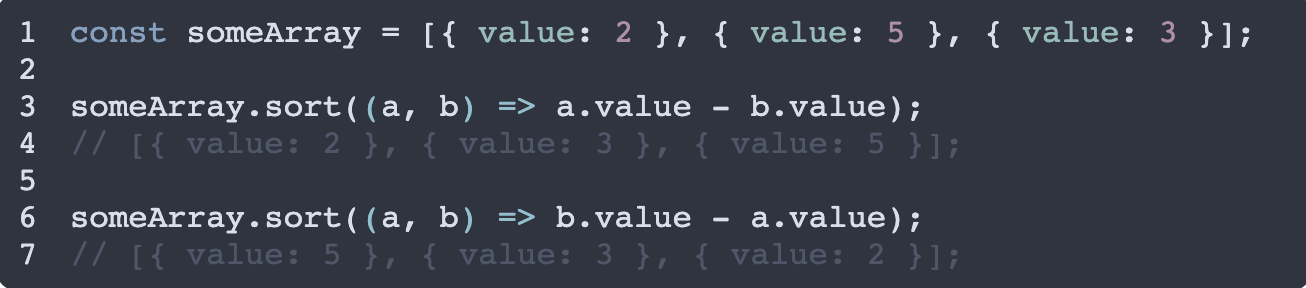