In this post we cover how to wait for all promises to resolve in JavaScript
How to wait for all promises to resolve in JavaScript
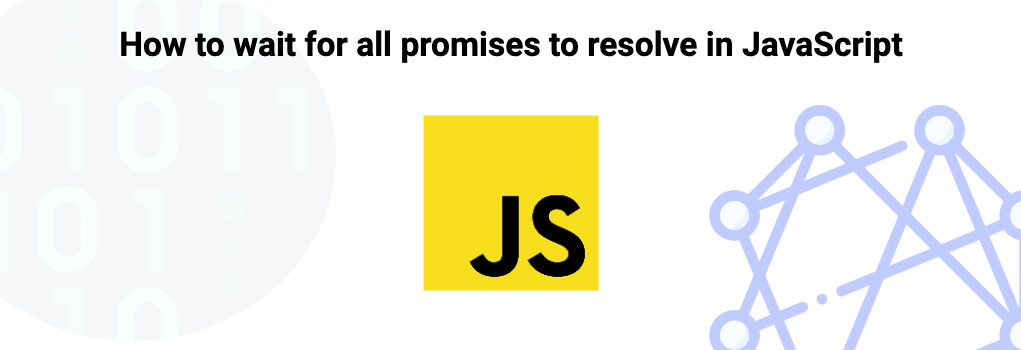
To wait for all promises to resolve in JavaScript Promise.all can be used. Promise.all, on the Promise object, accepts many promises within an array as an argument and only resolves after all promises in provided have been resolved.
1const requestA = fetch('https://softwareshorts.com/a');
2const requestB = fetch('https://softwareshorts.com/b');
3const requestC = fetch('https://softwareshorts.com/c');
4
5// Each request is a Promise that has not yet been resolved.
6const responses = await Promise.all([
7 requestA,
8 requestB,
9 requestC,
10]);
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Asynchronous
When something is asynchronous it just means that something can happen at a later point in time and instead of waiting for it, other things can be done in the meantime.
In software engineering it means the same, but it is applied to code. When a function or block of code is asynchronous it is simply saying that we do not know when this asynchronous action will be completed so the rest of the code can continue being processed whilst we wait for it so that it does not block other actions.
Promise
A Promise in JavaScript represents an asynchronous action or the completion of the asynchronous action.
A Promise can be either of three states, pending, resolved, or rejected.
Further resources
Related topics
Save code?
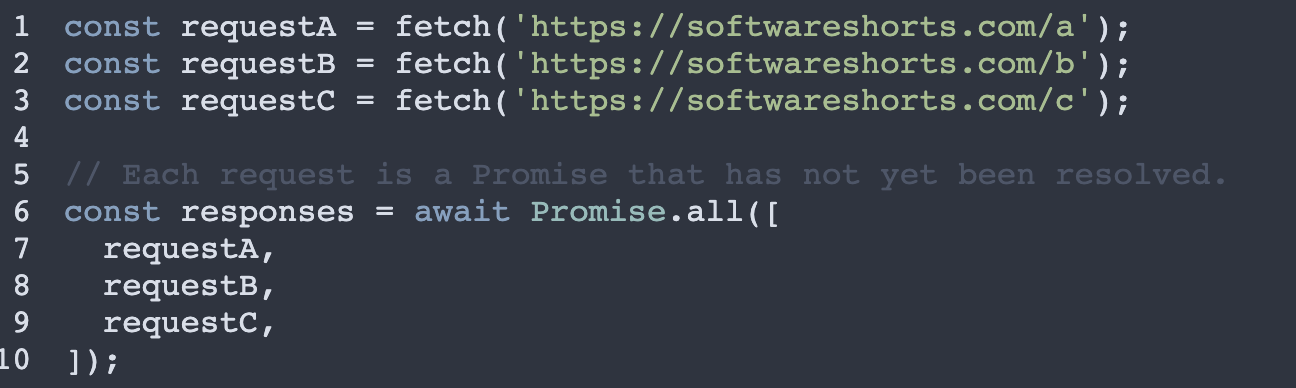