In this post we cover nullish Coalescing vs OR - JavaScript operators (?? vs ||)
Nullish Coalescing vs OR - JavaScript operators (?? vs ||)
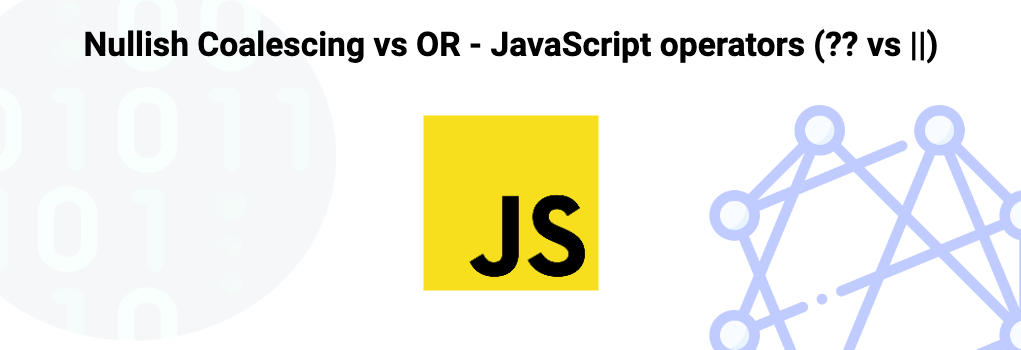
The main difference between the Nullish Coalescing Operator (??) and the Logical OR operator in JavaScript is that the nullish coalescing operator will fallback to the right hand argument only when the left hand argument is either null or undefined (Nullish). On the other hand the logical OR operator will fallback to the right hand argument for any falsy value which would include things like 0
or empty strings as well.
Here is an example of the differences of nullish coalescing vs OR:
1// null values
2
3// Nullish Coalescing Operator
4null ?? "Coffee" // 'Coffee'
5
6// Logical OR operator
7null || "Coffee" // 'Coffee'
8
9// falsy values
10
11// Nullish Coalescing Operator
120 ?? "Coffee" // '0'
13
14// Logical OR operator
150 || "Coffee" // 'Coffee'
In the above examples for the null values both produce the same result. But for the falsy values the results vary because the falsy value of 0
is not null or undefined so the Nullish Coalescing Operator does not default to Coffee
where as the Logical OR operator does default because it checks for falsy values.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Nullish Coalescing Operator
The Nullish Coalescing Operator (??) is a JavaScript operator introduced in ECMAScript 2020 that provides a concise way to handle nullish values.
It allows you to check if a value is null or undefined and provide a default value if it is.
Unlike the logical OR operator (||), which considers falsy values such as an empty string or zero as "falsey" and falls back to the default value, the Nullish Coalescing Operator only checks for null or undefined values.
This operator is particularly useful when you want to assign a default value to a variable only if it is null or undefined, ensuring that other falsy values are not overwritten.
For example, const result = value ?? defaultValue;
assigns defaultValue to result only if value is null or undefined.
Logical OR operator
The Logical OR operator (||
) is a JavaScript operator that evaluates two operands and returns the value of the first truthy operand.
It is typically used in conditional statements or assignments to provide fallback or default values. When the left operand is truthy, the operator returns the value of the left operand without evaluating the right operand.
If the left operand is falsy (e.g., false
, 0
, null
, undefined
, NaN
, or an empty string), the operator evaluates the right operand and returns its value.
This behavior makes the Logical OR operator useful for providing default values or handling optional values in JavaScript.
For example, const result = value || defaultValue;
assigns value
to result
if value
is truthy, but if value
is falsy, it assigns defaultValue
to result
.
Further resources
Related topics
Save code?
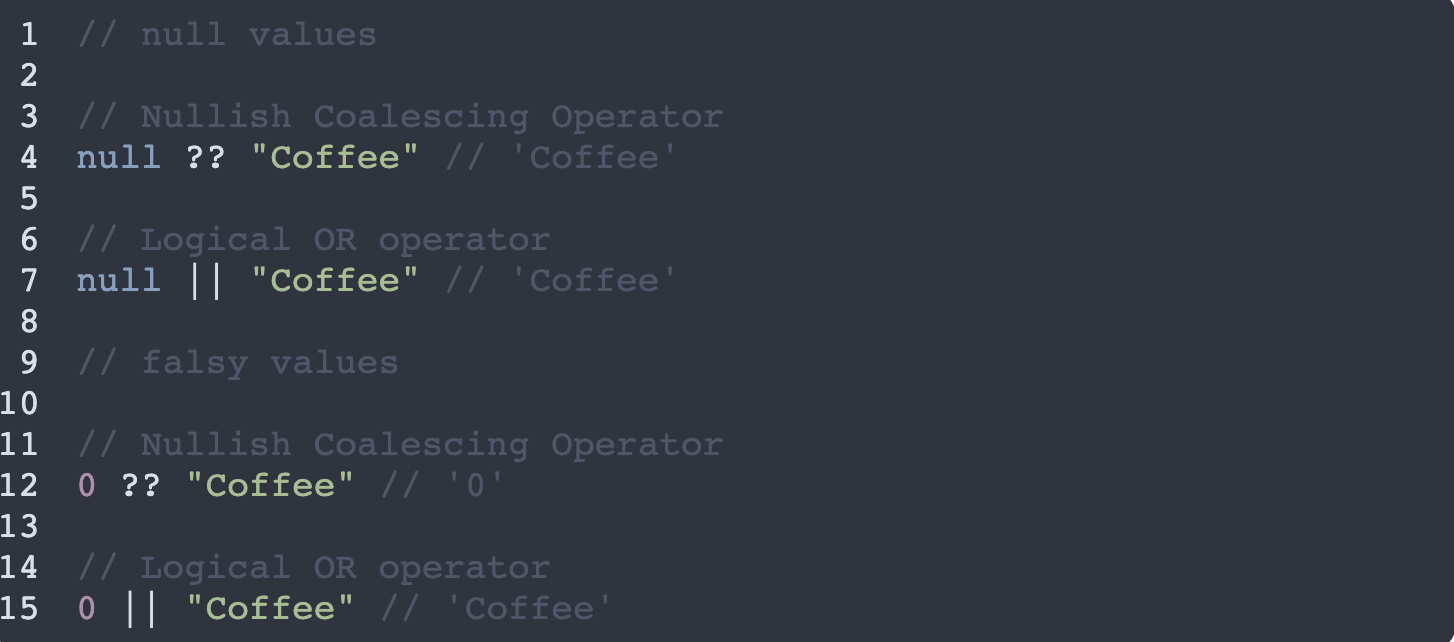