In this post we cover how to compare two dates in JavaScript
How to compare two dates in JavaScript
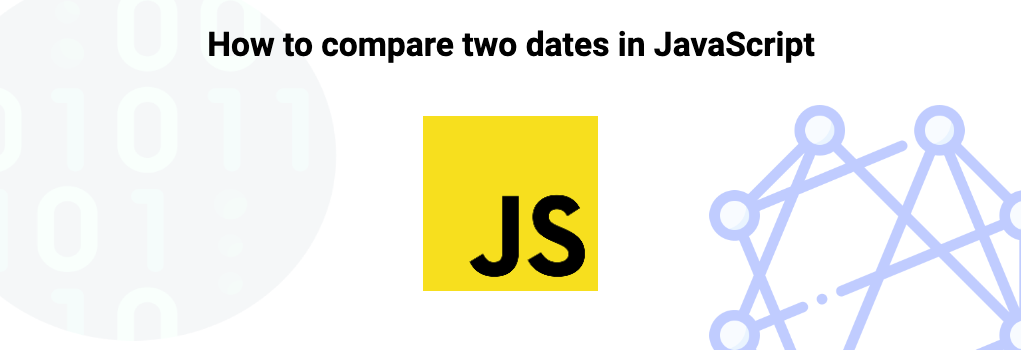
In JavaScript there are many ways to compare dates, but the gotcha is that two date objects/instances cannot directly be compared.
Instead some processing will be required in order to compare two dates.
1// Two new date objects given the same date.
2const dateA = new Date("10/08/2023");
3const dateB = new Date("10/08/2023");
4
5// comparisons of the date objects
6dateA === dateB // false
7dateA === dateA // true
8dateB === dateB // true
The above example illustrates why two date objects cannot be directly compared in JavaScript.
Due to the dates being objects unless it is the same reference being compared the comparison will always return a false value.
This is because in JavaScript two separate objects, even if they are identical will not return true.
In order to compare two different date objects we can process them to get a different data type so we can accurately compare them.
This is usually done by converting the date into either a string or a number.
1const dateA = new Date("10/08/2023");
2const dateB = new Date("10/08/2023");
3
4dateA === dateB // false
5dateA.getTime() === dateB.getTime() // true
6dateA.toString() === dateB.toString() // true
The above example shows both converting to a number and a string. the method getTime
on a date object can be used to convert the date into a timestamp which is the number of milliseconds since January 1, 1970, UTC.
For the string conversion the toString
is used which is a method used to convert the date into a date time string. For example "Sat Apr 15 2023 09:38:56 GMT+0100 (British Summer Time)"
To sort JavaScript dates a comparison function can be used:
1const someDates = [
2 new Date('10/08/2023'),
3 new Date('02/07/2022')
4];
5
6const sortedDates = someDates.sort((dateA, dateB) => {
7 if (dateA.getTime() > dateB.getTime()) {
8 return 1;
9 }
10
11 if (dateA.getTime() < dateB.getTime()) {
12 return -1;
13 }
14
15 return 0;
16});
17
18// sortedDates
19// [
20// new Date('02/07/2022'),
21// new Date('10/08/2023'),
22// ]
Alternatively to the above vanillaJS options, there are several libraries such as date-fns, Luxon, and Day.js. That provide methods out of the box to be able to run comparisons.
Here are a few examples of some helpers from date-fns:
1import { isBefore, isAfter, areEqual, isSameDay } from 'date-fns';
2
3const dateA = new Date('10/08/2023')
4const dateB = new Date('02/07/2022')
5
6isBefore(dateA, dateB) // false
7isAfter(dateA, dateB) // true
8areEqual(dateA, dateB) // false
9isSameDay(dateA, dateB) // false
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
VanillaJS
VanillaJS is a term often used to describe plain JavaScript without any additions, libraries or frameworks.
In other words when writing with VanilliaJS you are simply writing JavaScript as it comes out of the box.
JavaScript Date Object
The JavaScript Date Object is used to capture a moment in time and to be able to use this time programatically.
Further resources
Related topics
Save code?
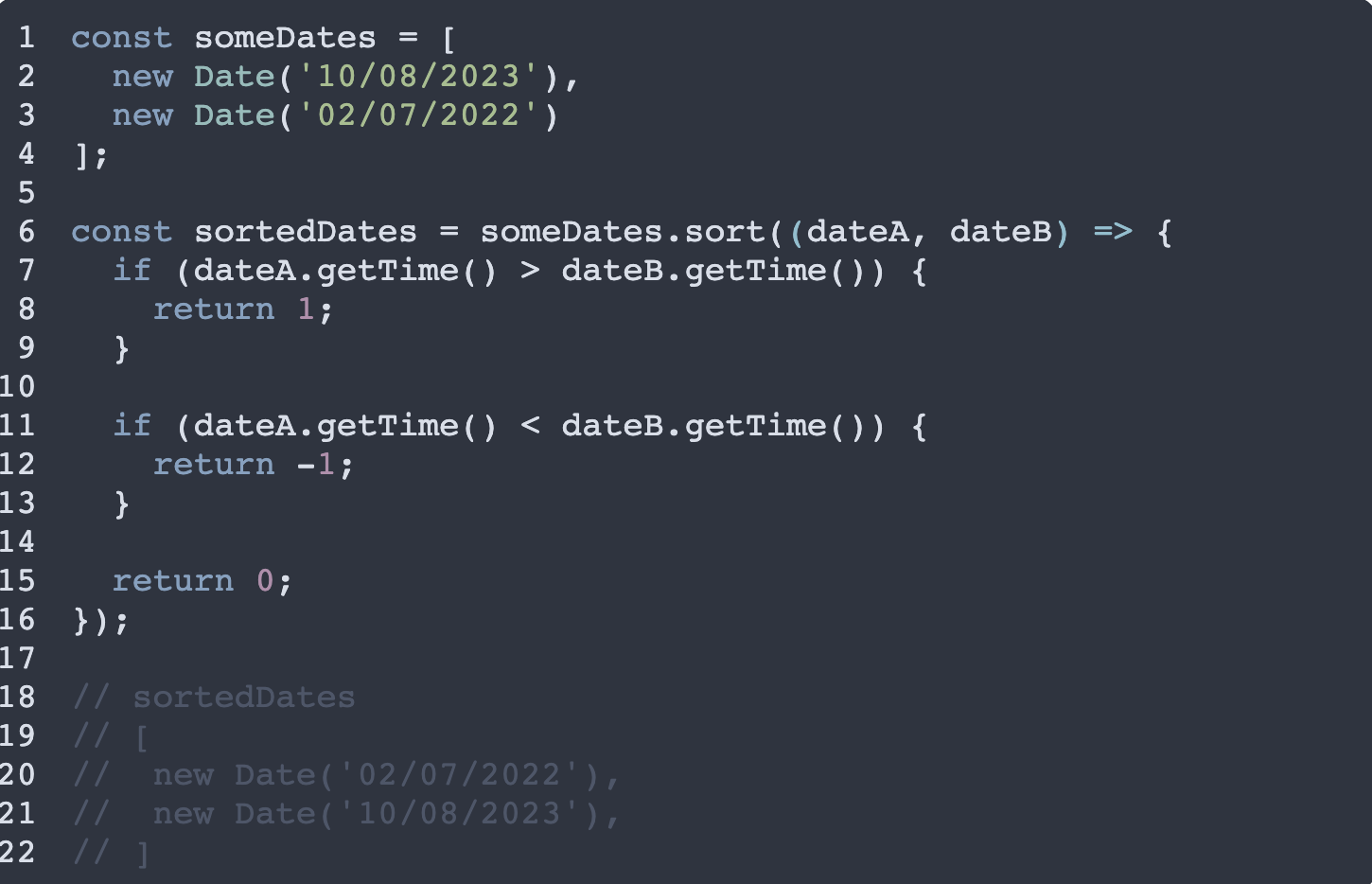