In this post we cover how to get the month name in JavaScript
How to get the month name in JavaScript
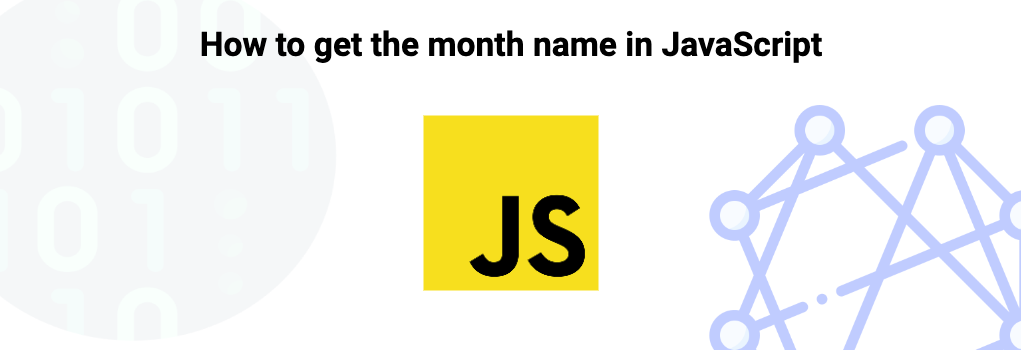
There are a few different ways on how to get the month name in JavaScript. Some with VanillaJS and some with libraries or custom solutions.
Here are a few easy examples showing how to get the month name in JavaScript:
1// VanillaJS - toLocaleString
2
3const date = new Date("09/21/2023");
4date.toLocaleString("default", { month: "long" });
5// September
The above example makes use of the method Date.prototype.toLocaleString
to return the long version of the month which is what the second argument defines.
The first argument is the language that is used. Default will take the default of the browser but other ISO date codes can be supplied instead.
1// VanillaJS - Intl.DateTimeFormat
2
3const date = new Date("09/21/2023");
4const month = new Intl.DateTimeFormat('default', {
5 month: 'long'
6 })
7 .format(date));
8
9console.log(month); // September
10
The above example is almost identical other than instead of using the built in date method, Intl.DateTimeFormat
is used instead which works the same but in reverse.
The date time format is created and then dates can be passed in to be formatted to it.
1// VanillaJs - getMonth
2const date = new Date("09/21/2023");
3date.getMonth(); // 8
The getMonth method works by simply retuning the numerical value for the month (starting from a 0 index so September for example is "8" rather than the normal "09").
This can be used to reference some translations stored in the application to get the month name as well.
1// DateFns - format
2import { format } from "date-fns";
3
4const date = new Date("09/21/2023");
5format(date, "LLLL"); //September
Lastly, the above code is an example using the date-fns library which provides a format method that can be used to customise the format of a date to however it needs to be.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
VanillaJS
VanillaJS is a term often used to describe plain JavaScript without any additions, libraries or frameworks.
In other words when writing with VanilliaJS you are simply writing JavaScript as it comes out of the box.
JavaScript Date Object
The JavaScript Date Object is used to capture a moment in time and to be able to use this time programatically.
Further resources
Related topics
Save code?
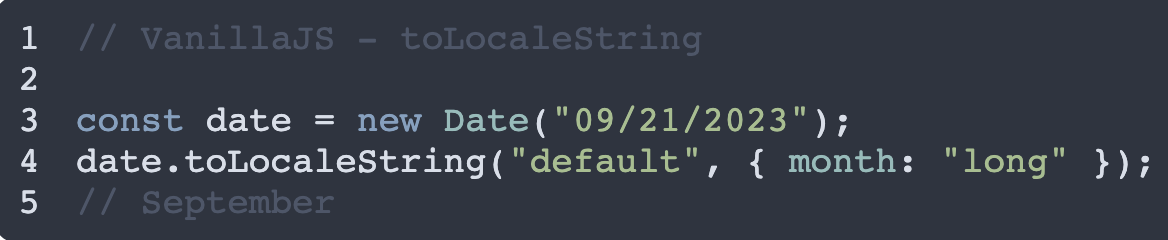