In this post we cover how to break a JavaScript forEach loop
How to break a JavaScript forEach loop
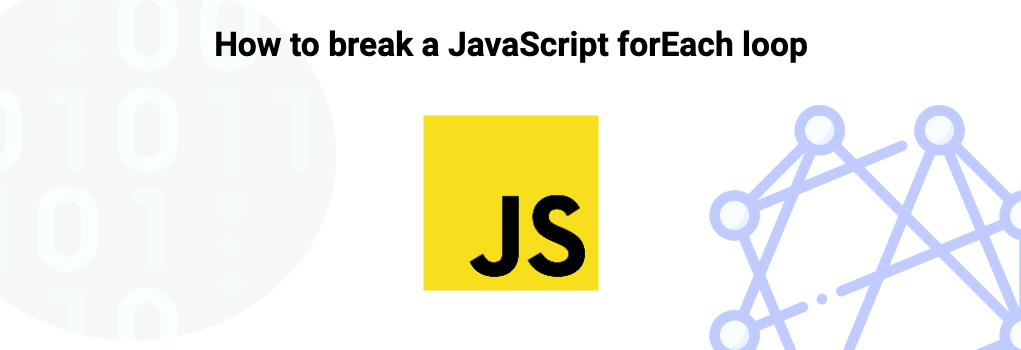
It is not possible to break the Array.prototype.forEach method in JavaScript due to how it works.
Where the method only allows a callback to be passed in there is no "clean" way to end the loop early because it is a syntactical error to use the break statement outside of a loop body causing: "Uncaught SyntaxError: Illegal break statement"
.
This shouldn't be a big issue though because unless very large arrays (length > 1000) are being handled it is unlikely to make a noticeable impact on the performance of the code.
In the case where small enough arrays are being used a condition can be used to return as soon as possible within the callback to reduce the amount of processing done so that it has the same result as breaking the array even though all iterations will still be completed.
Instead, if a break is needed to prevent the entire array being iterated over, then a for loop, a while loop, or Array.prototype.some should be considered instead.
Here are a few examples outlining the above suggestions:
1// conditional early returns
2
3[1, 2, 3, 4, 5].forEach((item) => {
4 if (item > 2) return;
5 console.log(item);
6});
7
8// output:
9// 1
10// 2
1// Array.prototype.some
2
3[1, 2, 3, 4, 5].some((item) => {
4 if (item > 2) return true;
5 console.log(item);
6});
7
8// output:
9// 1
10// 2
Array.prototype.some will return as soon as the callback condition has been met. This means that by returning true in the callback it will end the loop early.
1// for loop
2
3const someArray = [1, 2, 3, 4, 5];
4for (let i = 0; i < someArray.length; i++) {
5 const item = someArray[i];
6 if (item > 2) break;
7 console.log(item);
8}
9
10// output:
11// 1
12// 2
1// while loop
2
3const someArray = [1, 2, 3, 4, 5];
4let counter = 0;
5
6while (counter < someArray.length) {
7 const item = someArray[i];
8 if (item > 2) break;
9 console.log(item);
10 counter += 1;
11}
12
13// output:
14// 1
15// 2
Lastly, as mentioned above there is no "clean" way in which to break a forEach loop early there is however a way to do it that is not recommended and has no real use case because of the many other options that exist like in the examples above.
To do so an exception can be thrown in the callback and caught outside which will prevent further iterations. But again this is not recommended and there is no reason this is ever needed.
Here is how it would look:
1// Exception
2try {
3 [1, 2, 3, 4, 5].forEach((item) => {
4 if (item > 2) throw Error('break');
5 console.log(item);
6 });
7} catch (e) {
8 if (e.message !== 'break') {
9 console.error(e);
10 }
11}
12// output:
13// 1
14// 2
Array.prototype.forEach
Array.prototype.forEach or Array.forEach is a method on a Javascript array that will allow you to use a callback function to loop over each item in the array.
It is essentially syntactic sugar for a for loop with a given array, although it is not an exact replacement for a for loop because of things like async code.
The callback will be called for each iteration of the loop with each item passed in as an argument of the callback.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Loop
A loop in software engineering is reoccurring code that runs a number of times based on what it has been provided.
There are a number of ways to run loops, however for the most part there will be a iterator variable that will be incremented in value for each iteration of the loop. The iterator is usually set to the number 0, to start and after each iteration or run of the loop the variable will be incremented or increased by 1.
In order to prevent an infinite loop which is where the code gets stuck endlessly running the loop over and over again there is often a condition set that when met will end or break out of the loop.
For example if the loop increments by 1 for each iteration, then the condition set could be that while the iterator is less than the value of 10, run the loop otherwise break it.
A common condition is to find the total length of an array and use that to determine when each item has been looped over.
Array.prototype.some
Array.prototype.some is a method in JavaScript that can be used to find out if at least one item in a given array passes the criteria given in the callback provided to it.
It accepts a callback as an argument where the array item, the index and array will be passed into the callback as arguments.
The callback will need to return true or false. If the callback returns true then the element has been found and the iterations will stop, otherwise the method will continue iterating over the array until the element is found or the array is fully iterated over.
The result of the function will be a boolean true or false depending on if at least one element has been found that passes the condition within the provided callback.
For example:
[1, 2, 3].some(item => item > 3); // false
[1, 2, 3].some(item => item > 2); // true
Further resources
Related topics
Save code?
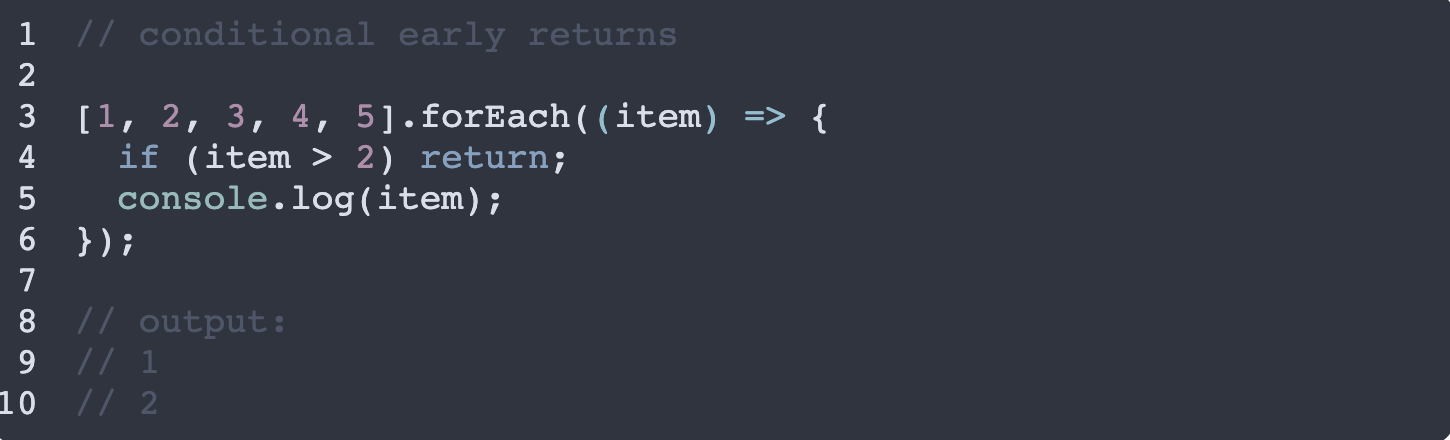