In this post we cover what do multiple arrow functions mean in JavaScript
What do multiple arrow functions mean in JavaScript?
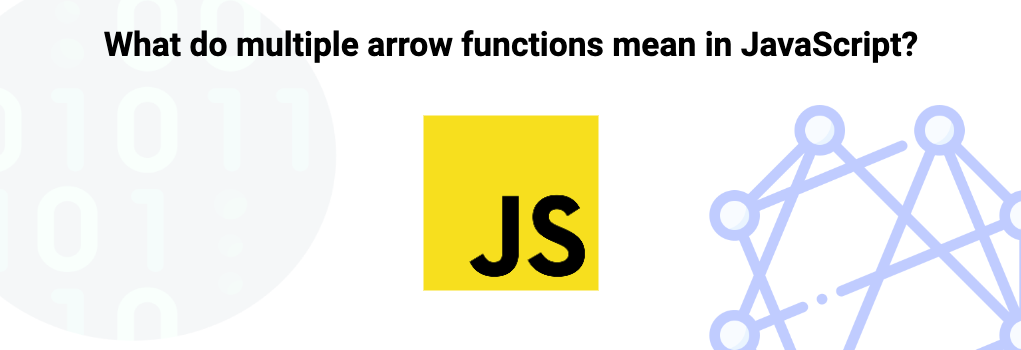
Multiple arrow functions in JavaScript indicate the creation of a closure.
Here is an example of the creation and use of multiple arrow functions:
1const createGreeting =
2 (name) => () => { console.log("Hello ", name); };
3
4const greetWorld = createGreeting("World");
5
6greetWorld(); // Hello World
Arrow Function
An arrow function, or arrow function expression, is a way in JavaScript to define a function that has a few differences when compared to a standard function.
Some of the main differences are as follows:
- An arrow function cannot bind their own "this", "arguments", or "super", and instead it can access the ones from its parents scope.
- The syntax, it is shorter to create an arrow function.
- The return, anything to the right hand side of an arrow function will be returned so you do not always need to wrap your code in curly braces.
- An arrow function cannot be used as a constructor.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Closure
A closure is a concept where a function will return another function in order to create a private scope.
In JavaScript it is a powerful concept that allows a function to remember and access its lexical scope even when it is executed outside of that scope.
In simpler terms, a closure is created when an inner function has access to its parent function's variables, parameters, and even the parent function itself.
The inner function forms a closure, capturing and preserving the state of its surrounding environment.
This enables the inner function to continue referencing and using those variables and values, even after the parent function has finished executing.
Closures are often used to create private variables, encapsulate functionality, and maintain data integrity in JavaScript applications.
They are an essential feature for advanced programming techniques and play a crucial role in functional programming paradigms.
Understanding closures allows developers to write more efficient and modular code, enhancing the flexibility and robustness of their JavaScript applications.
Further resources
Related topics
Save code?
