In this post we cover how to export multiple functions in JavaScript
How to export multiple functions in JavaScript
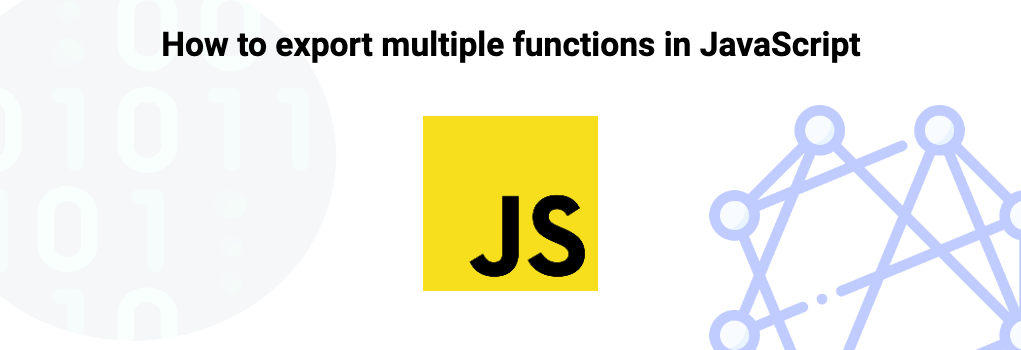
To export multiple functions in JavaScript using ES6 or later (which essentially all projects will be) the simplest way is to add the export
statement before the function.
1// mathHelpers.js
2
3export function add(a, b) {
4 return a + b;
5}
6
7export function multiply(a, b) {
8 return a * b;
9}
10
11export function divide(a, b) {
12 return a / b;
13}
To import these exports you can access them by using the import statement.
1import { add, multiply, divide } from 'mathHelpers';
exporting a default function is slightly different because even though you can have as many exports as you like, you can only have a single default export.
1// mathHelpers.js
2
3export default function add(a, b) {
4 return a + b;
5}
6
7export function multiply(a, b) {
8 return a * b;
9}
10
11export function divide(a, b) {
12 return a / b;
13}
Default exports like in the above code snippet can be imported like so:
1import add, { multiply, divide } from 'mathHelpers';
But it is important to remember that there can only be one default export per file.
You can use the export statement to export anything you like from a file, including strings, numbers, arrow functions, objects and arrays.
1export const add = (a, b) => a + b;
2
3export const multiply = (a, b) => a * b;
4
5export const divide = (a, b) => a / b;
6
7export const pi = "3.14"
8
9export const zero = 0;
Another option is to group the exports together like so:
1const add = (a, b) => a + b;
2const multiply = (a, b) => a * b;
3const divide = (a, b) => a / b;
4
5export { add, multiply, divide };
And lastly, if you cannot use ES6 then you can use module.exports like so:
1const add = (a, b) => a + b;
2const multiply = (a, b) => a * b;
3const divide = (a, b) => a / b;
4
5module.exports = { add, multiply, divide };
In summary, there are many ways to export multiple functions in JavaScript but all of them will make use of the export statement or module.exports.
ES6
ES6 is short for ECMAScript 6, which is a standardisation of JavaScript where 6 is the 6th version of it. It brought many major improvements to JavaScript which has shaped it to be how it looks today.
Arrow Function
An arrow function, or arrow function expression, is a way in JavaScript to define a function that has a few differences when compared to a standard function.
Some of the main differences are as follows:
- An arrow function cannot bind their own "this", "arguments", or "super", and instead it can access the ones from its parents scope.
- The syntax, it is shorter to create an arrow function.
- The return, anything to the right hand side of an arrow function will be returned so you do not always need to wrap your code in curly braces.
- An arrow function cannot be used as a constructor.
Further resources
Related topics
Save code?
