In this post we cover what are Expo modules in React Native
What are Expo modules in React Native
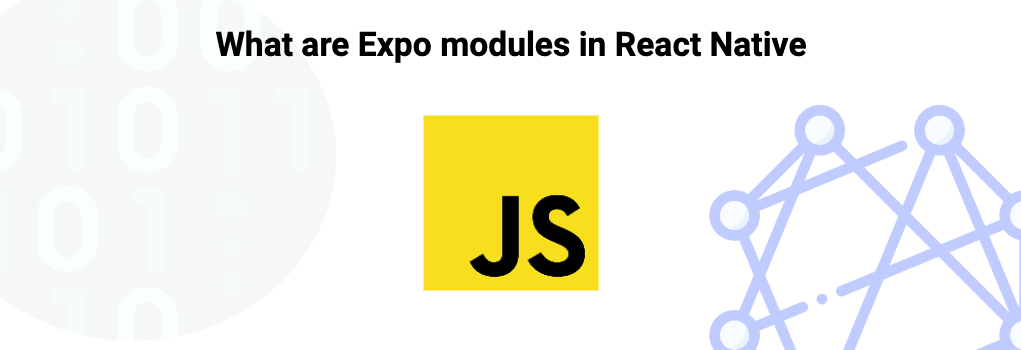
Expo modules are specialised packages that extend the functionality of Expo and React Native applications by providing access to native device features through a set of pre-built APIs. These modules simplify the development process by abstracting the complexities involved in integrating native code, making it easier for developers to add advanced functionalities such as camera access, location services, sensors, and notifications to their applications. The primary advantage of using Expo modules is the streamlined development experience they offer, allowing developers to write and manage their applications with less effort and more consistency.
Why Expo Modules are Useful
Expo modules are incredibly useful because they offer a unified, pre-configured environment that reduces the overhead associated with setting up and managing native code. They allow developers to focus on building features rather than dealing with the intricacies of platform-specific code. Additionally, Expo modules ensure that applications maintain consistent behavior across different devices and operating systems, which is crucial for providing a seamless user experience. The managed workflow, comprehensive documentation, and extensive community support further enhance the usability of Expo modules.
Implementing Expo Modules
Implementing Expo modules is a straightforward process, thanks to the tools and abstractions provided by Expo. Here’s a step-by-step guide to creating and using an Expo module with the requireNativeModule function:
1. Create the Native Module
First, create the native module using native code (Objective-C/Swift for iOS or Java/Kotlin for Android). Ensure that the native module is properly registered.
Example in Swift (iOS):
1// MyNativeModule.swift
2import ExpoModulesCore
3
4public class MyNativeModule: Module {
5 public func definition() -> ModuleDefinition {
6 Name("MyNativeModule")
7
8 Function("doSomething") {
9 // Native functionality
10 }
11 }
12}
2. Use requireNativeModule in JavaScript
In your JavaScript code, use requireNativeModule to load the native module.
1import { requireNativeModule } from 'expo-modules-core';
2
3const MyNativeModule = requireNativeModule('MyNativeModule');
4
5export function useNativeFeature() {
6 MyNativeModule.doSomething();
7}
3. Access Native Features
Call the functions provided by the native module as if they were regular JavaScript functions.
Example of using the native feature in a React component:
1import React from 'react';
2import { Button, View } from 'react-native';
3import { useNativeFeature } from './my-native-feature';
4
5export default function App() {
6 return (
7 <View>
8 <Button title="Do Something Native" onPress={useNativeFeature} />
9 </View>
10 );
11}
Conclusion
Using Expo modules with the requireNativeModule function simplifies the process of accessing and integrating native modules in your Expo projects. This approach eliminates much of the boilerplate code and setup typically required for native modules, allowing developers to focus on building features and enhancing their applications. By leveraging Expo’s robust ecosystem and tools, developers can efficiently create performant, cross-platform mobile applications with a rich set of features. For more detailed information, you can refer to the Expo Modules documentation.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Expo
Expo is a framework and platform for building React Native applications that simplifies the development process for both iOS and Android. It provides a comprehensive set of tools and services, including the Expo SDK, which offers APIs for accessing native device functionalities like cameras, sensors, and notifications. With Expo, developers can write and manage their applications from a single codebase, leveraging a rich library of pre-built components and modules designed to streamline the creation of high-quality mobile apps.
A standout feature of Expo is its Expo Application Services (EAS), which facilitate various aspects of the app development lifecycle. EAS Build allows for cloud-based compilation of iOS and Android apps, eliminating the need for a local build environment. EAS Submit streamlines the submission process to app stores, while EAS Update enables over-the-air updates, allowing developers to push changes to live apps without requiring users to download updates from the app store. These services significantly speed up development and deployment, making it easier to maintain and update applications in production.
Expo also supports integration with various tools and libraries to enhance app functionality. It provides seamless access to native device features and supports popular state management and navigation libraries, making it a powerful and flexible choice for developers. The Expo ecosystem, combined with its ease of use and comprehensive services, makes it an ideal platform for developing robust and scalable mobile applications.
React Native
React Native is a popular open-source framework developed by Facebook for building mobile applications using JavaScript and React. It enables developers to create natively-rendered mobile apps for both iOS and Android platforms from a single codebase. React Native leverages the React library for building user interfaces, and it translates React components into native components, providing a near-native performance and user experience.
One of the key advantages of React Native is its ability to use a single codebase to develop apps for multiple platforms, significantly reducing development time and effort. It also supports hot reloading, which allows developers to see changes in real-time without recompiling the entire app. This accelerates the development process and enhances productivity. Additionally, React Native has a vast ecosystem of libraries and plugins, making it easier to integrate various functionalities and streamline app development.
Expo Modules
Expo modules are targeted packages that extend the capabilities of Expo and React Native applications by offering direct access to native device features through easy-to-use APIs. These modules simplify the addition of functionalities such as camera usage, geolocation, and notifications, eliminating the need for intricate native code configurations. By providing a cohesive development environment and extensive documentation, Expo modules enable developers to efficiently build powerful, cross-platform mobile applications with less complexity and more consistency.
Further resources
Related topics
Save code?
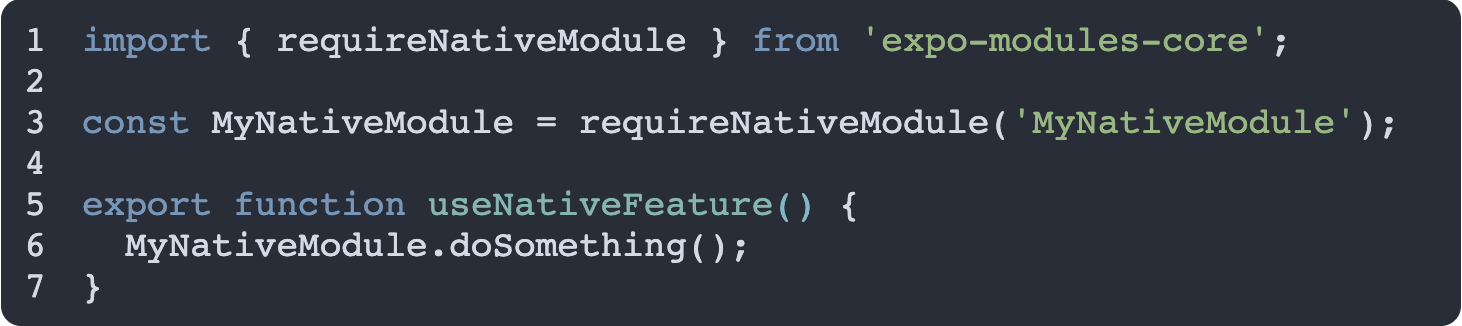