In this post we cover React.Fragment vs div
React.Fragment vs div
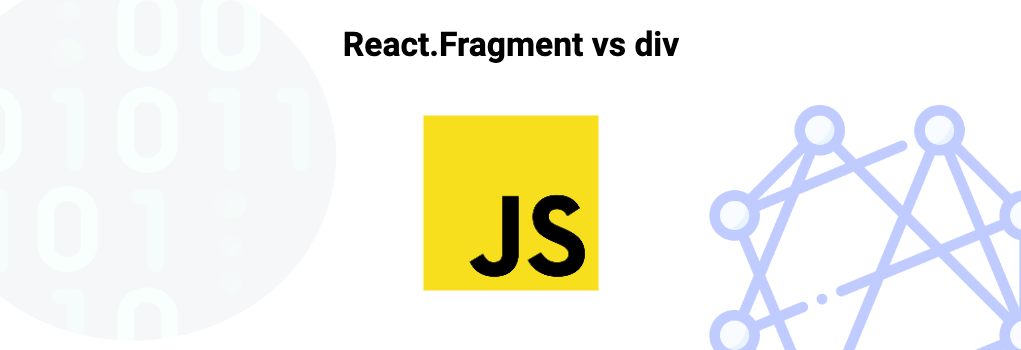
The primary difference between a React fragment and a div html element is that a fragment does not add new elements into the DOM tree. This means that in situations where a containing element is only needed in order to group other elements and components within React then by using a fragment it removes the additional element that would have otherwise been added.
Additionally fragments cannot be styled or have event listeners added and many other attributes and properties that a HTML element can have.
It is worth noting that fragments can however contain a "key" property.
The most common use case of a fragment in React is to be able to group many components to a single return statement without having to create new DOM elements to do it. This is often done in normal components as well as when mapping over an array to create many components.
Here is a basic example of the two side by side:
1// fragment
2export default function App() {
3 return (
4 <>
5 <p>Hello</p>
6 <p>World</p>
7 </>
8 );
9}
10
11// results in the following HTML:
12// <p>Hello</p>
13// <p>World</p>
1// div element
2export default function App() {
3 return (
4 <div>
5 <p>Hello</p>
6 <p>World</p>
7 </div>
8 );
9}
10
11// results in the following HTML:
12// <div>
13// <p>Hello</p>
14// <p>World</p>
15// </div>
1// Shorthand:
2<></>
3
4// Longhand:
5<React.Fragment></React.Fragment>
React Key
A React key is something used to track items in an array that gets rendered in React. It helps to keep track of which item is which between renders given that the array items can change.
To prevent issues React asks you to pass in a unique key for each item in the array so if the array changes React knows how to handle it.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Loop
A loop in software engineering is reoccurring code that runs a number of times based on what it has been provided.
There are a number of ways to run loops, however for the most part there will be a iterator variable that will be incremented in value for each iteration of the loop. The iterator is usually set to the number 0, to start and after each iteration or run of the loop the variable will be incremented or increased by 1.
In order to prevent an infinite loop which is where the code gets stuck endlessly running the loop over and over again there is often a condition set that when met will end or break out of the loop.
For example if the loop increments by 1 for each iteration, then the condition set could be that while the iterator is less than the value of 10, run the loop otherwise break it.
A common condition is to find the total length of an array and use that to determine when each item has been looped over.
Further resources
Related topics
Save code?
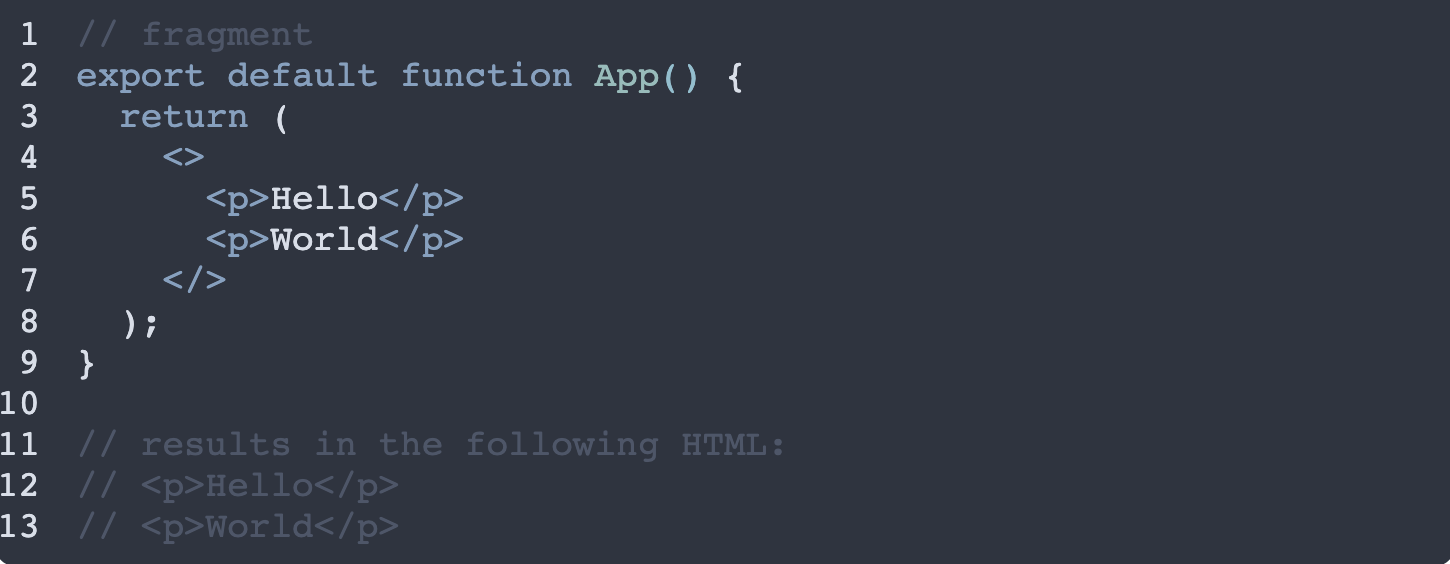