Converting data into components is a fundamental part of using React. In this post we learn how to render an array of objects with Array.map in React.
How to render an array of objects with Array.map in React
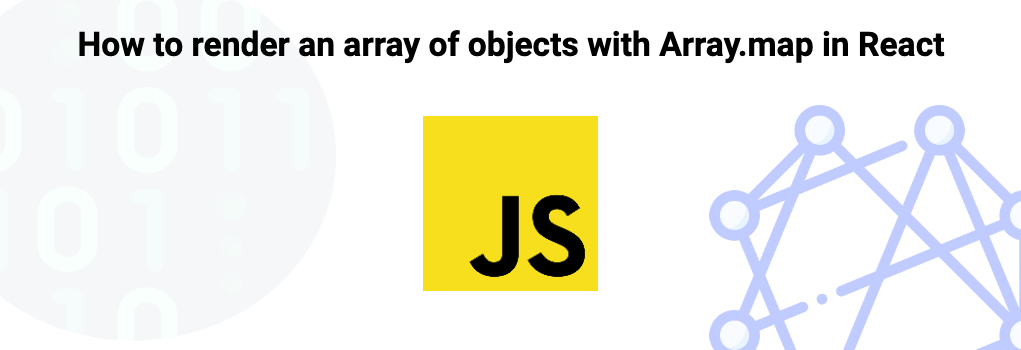
To render an array of data in React, we first need to map/convert the array of data into an array of components, which can be done with Array.prototype.map.
After converting the array of data into an array of components, we simply need to pass the new array of components into the JSX which will render the components containing the data.
Here is a full example:
1const coffeeData = [
2 { coffee: "Americano", size: "small", price: "2.99" },
3 { coffee: "Americano", size: "medium", price: "3.99" },
4 { coffee: "Americano", size: "large", price: "4.99" },
5 { coffee: "Espresso", size: "single", price: "1.99" },
6 { coffee: "Espresso", size: "double", price: "2.99" },
7];
8
9export default function CoffeeList() {
10 return (
11 <>
12 {coffeeData.map(({ coffee, size, price }) => (
13 <p key={coffee + size}>A {size} {coffee} for {price}.</p>
14 ))}
15 </>
16 );
17}
The key prop is used in the paragraph element in the JSX to keep track of the items between renders and needs to be unique in the array. For the above example there is nothing truly unique as it, so we can create a unique set of data by combining the "coffee" and the "size".
The HTML output of the above example will look like the following:
1<body>
2 <p>A small Americano for 2.99.</p>
3 <p>A medium Americano for 3.99.</p>
4 <p>A large Americano for 4.99.</p>
5 <p>A single Espresso for 1.99.</p>
6 <p>A double Espresso for 2.99.</p>
7</body>
Tip: You can use the curly braces to input the data into any JSX component.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
React Key
A React key is something used to track items in an array that gets rendered in React. It helps to keep track of which item is which between renders given that the array items can change.
To prevent issues React asks you to pass in a unique key for each item in the array so if the array changes React knows how to handle it.
Array.prototype.map
Array.prototype.map or Array.map for short is a method on Javascript arrays that allows you to map/convert the array items to something else and does not mutate.
It accepts a function/callback that is run for each iteration with iterations array item, and the return value from that function will become the new item in the new array. Once complete it will return the new array containing the mapped/converted items so that you can store it as a variable.
For example, given an array of numbers, if you wanted to multiply each by 10 then you could call map on your array and pass in a function that accepts an item which will be a number, and then returns a value that uses the multiplication operator on the item to multiply it by 10.
This function would then be run for each item in the array multiplying each by 10, once the whole array has been run the map function will return the new multiplied array ready to be used or stored.
Further resources
Related topics
Save code?
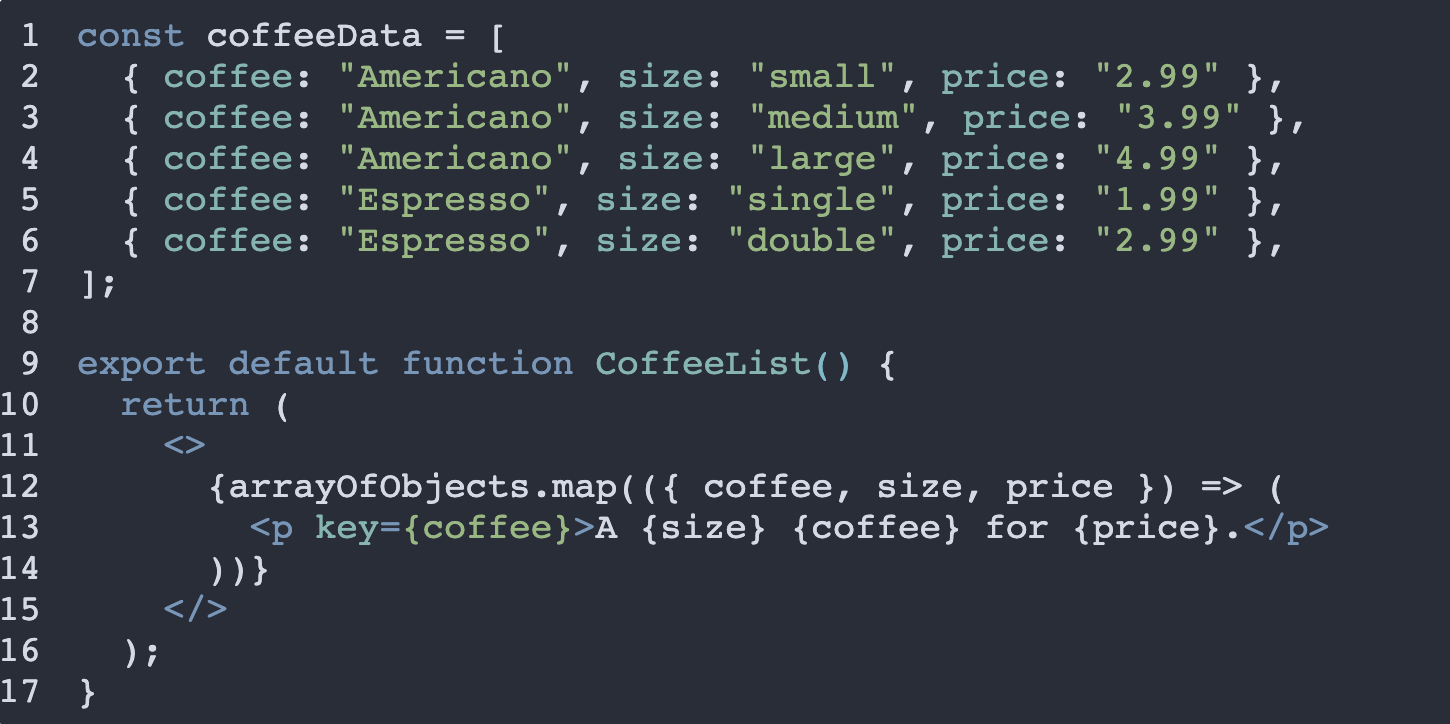