References and the useRef hook are essential tools to effectively write functional React code. Understanding how to use useRef with TypeScript is something you will want in your tool belt.
How to use useRef with TypeScript

To make use of useRef with typescript, it is essentially the same as it is in JavaScript when it comes to React.
The only difference is that you will be able to tell tsc what the value of the ref is allowed to be.
Let's take a look at an example of how to use useRef with TypeScript:
1const someRef = useRef<string>('');
1import React, { useRef } from "react";
2
3interface SayHi {
4 name: string
5}
6export default function SayHi({ name }: SayHi) {
7 const nameProvided = useRef<boolean>(false);
8
9 useEffect(() => {
10 // if the name has already been set initially
11 if (nameProvided.current) {
12 sendAnalyticsLog('The name has been changed');
13 return;
14 }
15
16 // if the name has not yet been set
17 sendAnalyticsLog('The name has been set initially');
18 nameProvided.current = true;
19 }, [name]);
20
21 return <p>Hi, {name}!</p>
22}
In the above code, we are provided with a name prop which is a stateful variable, because of this any time it is changed it will cause a re-render. We are also emitting analytics events in this component as well as returning a message to the user.
The analytics events we are logging in the above code need to be sent at the correct times.
To send them at the correct times we are using a boolean ref to make sure we only send one of the logs once.
We always reference the ref by getting the current
field on the variable that is created. That is because the output of useRef is "MutableRefObject
" along with the value you provide it.
So your variable is MutableRefObject
, and your variable ".current
" is the value you assign to it.
In the above code this would be <a href="/definition/react/">React</a>.MutableRefObject<boolean>
In summary, to use useRef in TypeScript it is identical to how you would use it in JavaScript with the sole exception of providing the value of what the ref can be when you define it.
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
useRef
The useRef hook creates a reference as a variable which can be used for a number of things in a React application.
This reference is mutable, unlike stateful variables.
The most common use case is to assign DOM elements to them so you can view and modify them outside of the standard React lifecycle.
However, any value can be assigned to a ref, such as a string, an object, a number and so on.
At its core, a ref is a variable that get's persisted between renders and does not cause any renders of its own.
You can access the value by getting the current field on the ref.
Mutable
Mutable is where something in a system can be directly modified.
For example, re-assigning the value of a variable would be mutating the variable. Or pushing a new item into an array would be mutating the array.
Further resources
Related topics
Save code?
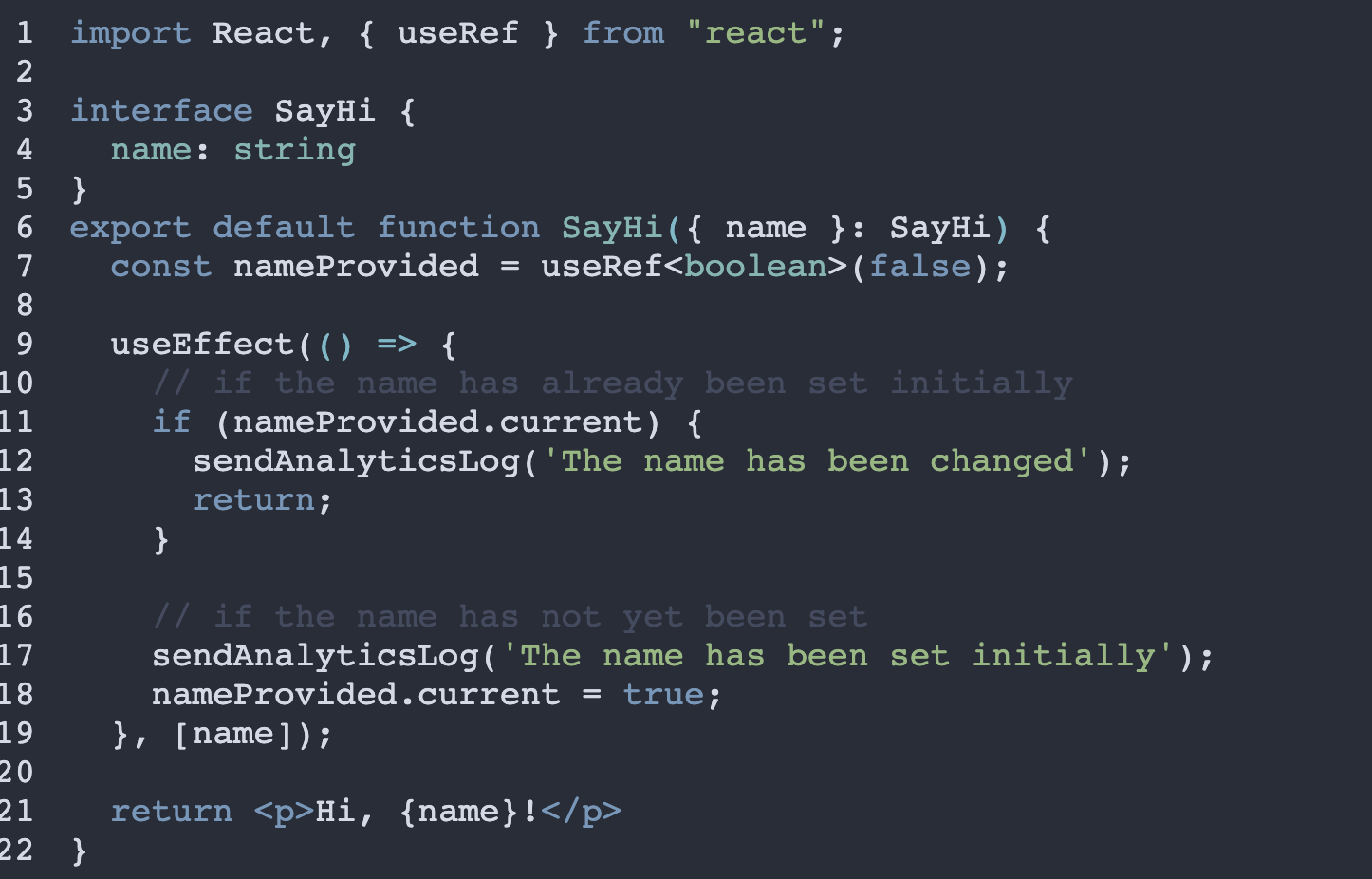