In this post we cover how to test custom react hooks
How to test custom react hooks
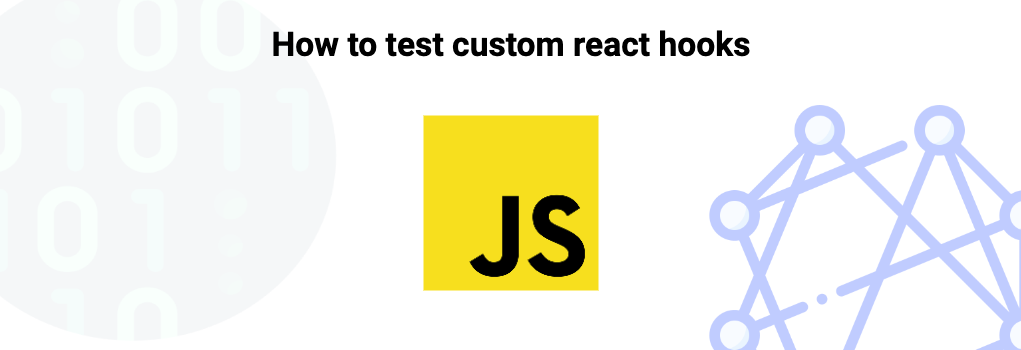
Testing custom react hooks can be done by using the @testing-library/react-hooks
library along with the renderHook
function that comes out of the box.
It is possible to test hooks without the library but for the most part the @testing-library/react-hooks
library will be simpler, easier to use whilst being reliable with a lot of support.
To use the library it is very similar to using the React testing library and making use of it within unit tests.
Here is an example hook:
1import { useState } from 'react';
2
3export function useDate() {
4 const [date, setDate] = useState(null);
5 return {
6 setDate,
7 date,
8 };
9}
And here is a basic unit test for the above custom hook using the @testing-library/react-hooks
library
1import { renderHook, act } from '@testing-library/react-hooks';
2import { useDate } from './useDate';
3
4describe('useDate', () => {
5 it('Should have default values', () => {
6 const { result } = renderHook(useDate);
7 expect(result.current.date).toBeNull();
8 });
9
10 it('Should update the date', () => {
11 const someDate = new Date();
12 const { result } = renderHook(useDate);
13
14 act(() => {
15 result.current.setDate(someDate);
16 });
17
18 expect(result.current.date.toString())
19 .toEqual(someDate.toString());
20 });
21});
React Render
In React a render is used to describe essentially a version/instance/frame of the page based on the values used to create it.
When something changes in a React based application it will trigger a re-render which is where React will re-draw/paint/create/render a page or application based on the latest values it has.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
Unit Test
Unit testing is a testing method where a "unit" is tested. A unit is just a small piece of code, usually the smallest piece that can be isolated by itself with as fewer dependancies as possible.
Unit testing is one of the main and most popular forms of testing in codebases that can also be the most effective and the cheapest form of testing code at scale.
Further resources
Related topics
Save code?
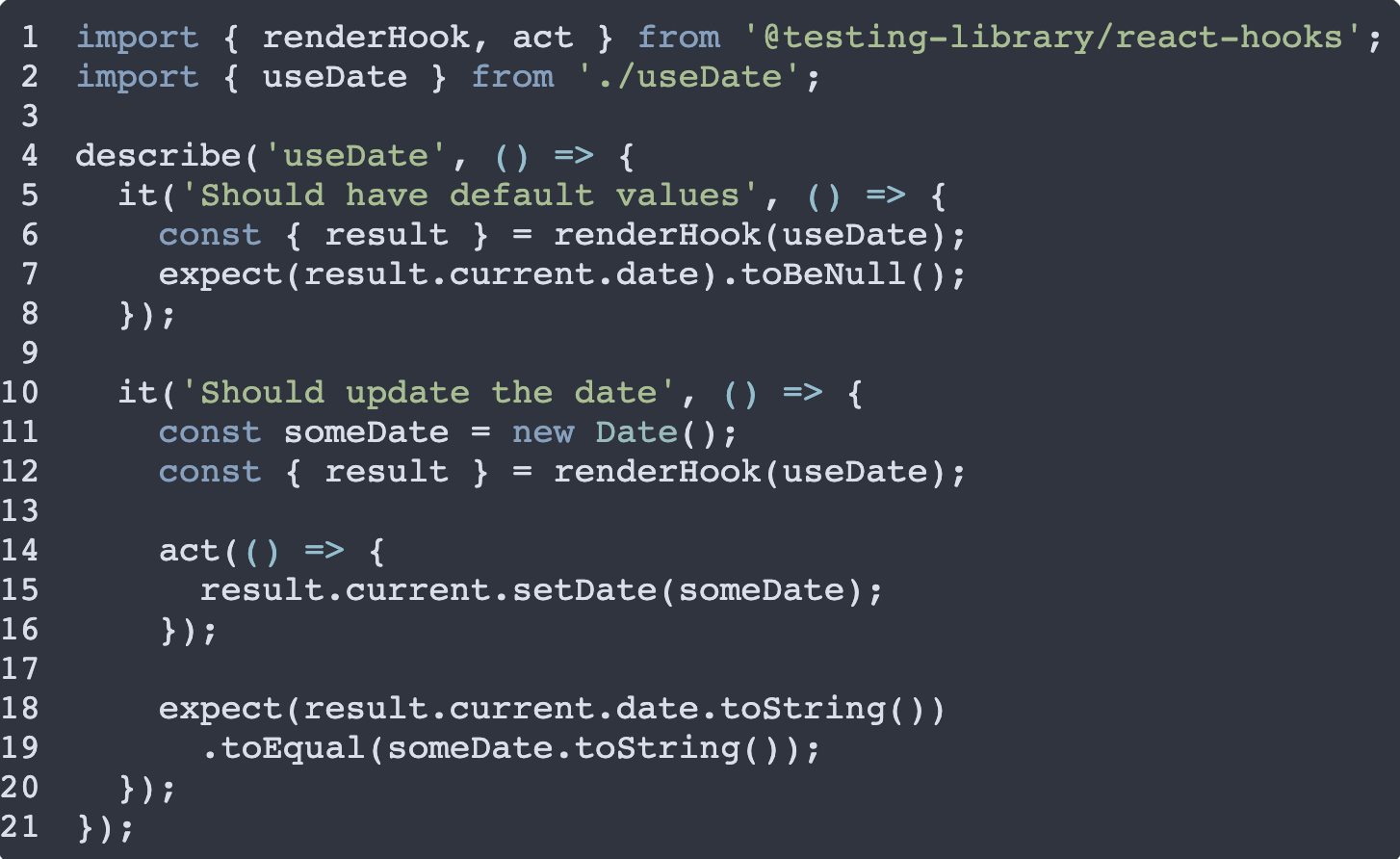