In this post we cover how to set the selected option on a select element with React and JSX
How to set the selected option on a select element with React and JSX
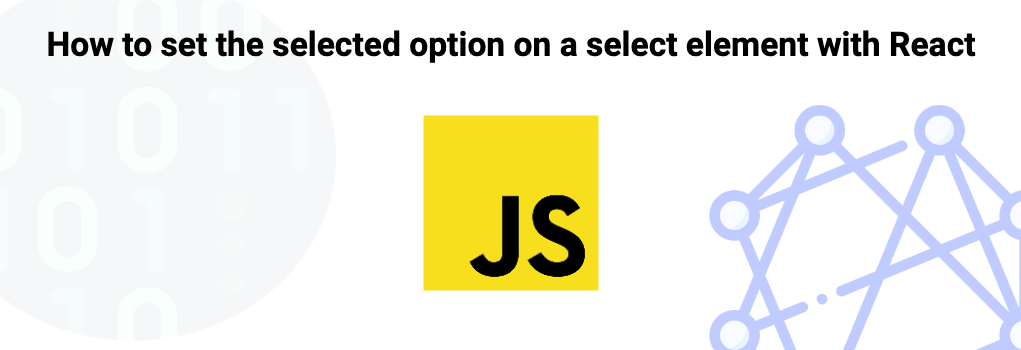
1export default function CoffeeSelect() {
2 const [selected, setSelected] = useState();
3
4 return (
5 <select
6 name="coffee"
7 value={selectedElement}
8 onChange={(e) => setSelected(e.target.value)}
9 >
10 <option value="robusta">robusta</option>
11 <option value="arabica">arabica</option>
12 </select>
13 );
14}
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JSX
JSX stands for "JavaScript XML" which in short means that you can use XML in JavaScript which is syntactic sugar for instead of writing out many function calls to create elements, you can just write the elements as if you were writing HTML or XML.
JSX is a concept introduced by React and is used heavily in React applications to build out components.
It is worth noting that the use of JSX is optional and you can still create elements by calling the appropriate functions.
Further resources
Related topics
Save code?
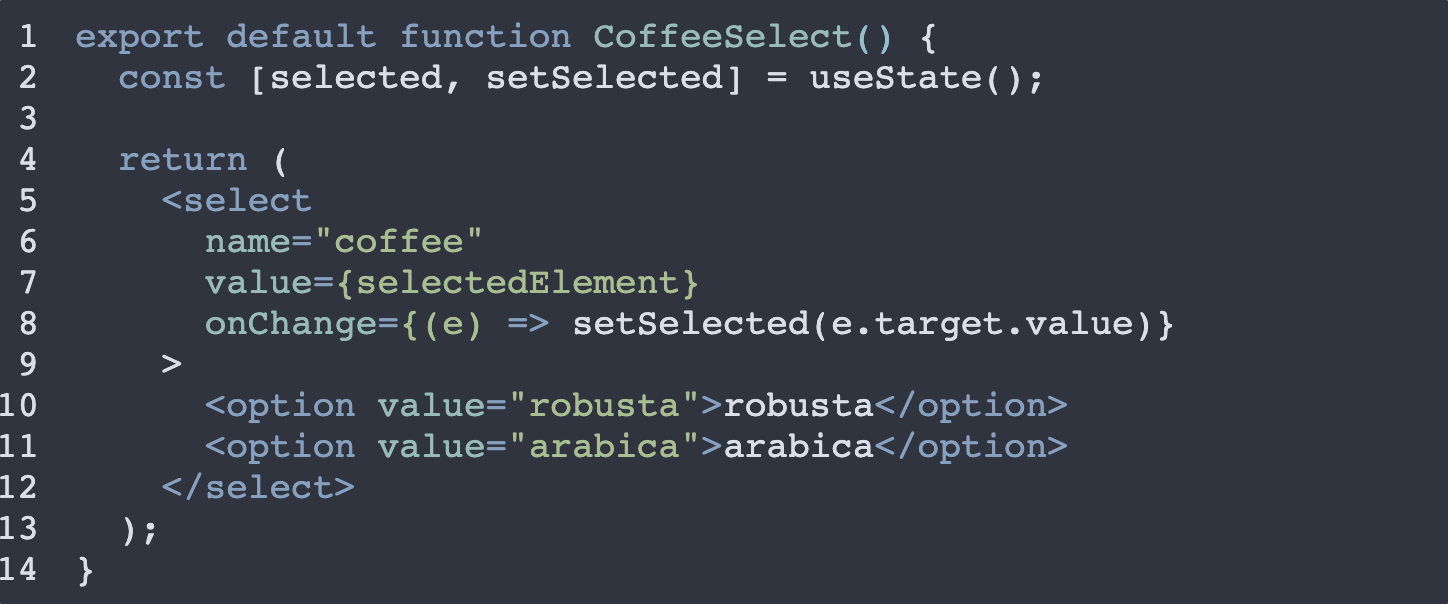