In this post we cover how to use setState from within useEffect
How to use setState from within useEffect
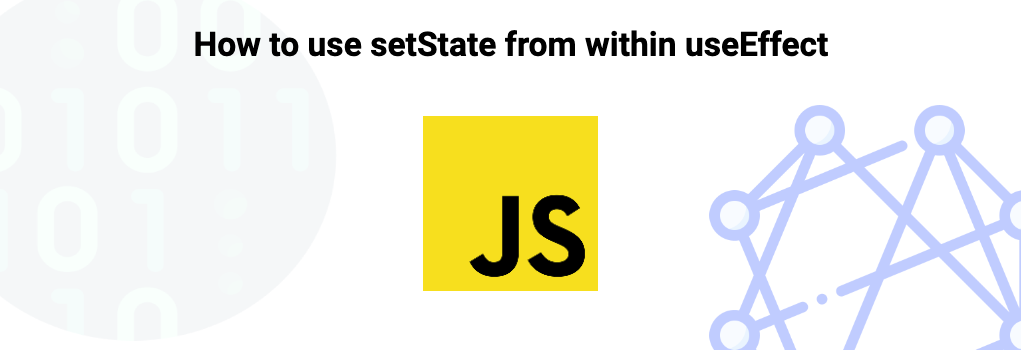
Using a setState function from a useState hook within a useEffect hook is safe to do without needing to include it in the dependancy array because is is guaranteed by React to never change. Read more here.
1import React, { useState } from 'react';
2
3export default function Add({ possibleNumber }) {
4 const [myNumber, setMyNumber] = useState(0);
5
6 useEffect(() => {
7 if(parseInt(possibleNumber, 10)) {
8 setMyNumber(parseInt(possibleNumber, 10);
9 }
10 }, [possibleNumber]);
11
12 return (<p> {myNumber} * 2 = {myNumber * 2}</p>);
13}
As you can see in the above code, we have not included the "setMyNumber" function in the useEffects dependancy array because it is not needed where it will never change.
And because it will never change it will never produce a stale variable.
In summary, to use setState from within useEffect, do do not need to do anything. The setState function is guaranteed by React never to change so it is not needed in the dependancy array of a useEffect because the setState function itself will never be stale because it never changes.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Stale Variables
Stale variables is a phenomenon when using React hooks to develop applications that happens if a state variable or prop has been changed but an effect has not been updated with the new value and is still using an old value.
This can happen because the state variable or prop has not been included in the dependancy array properly.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
Further resources
Related topics
Save code?
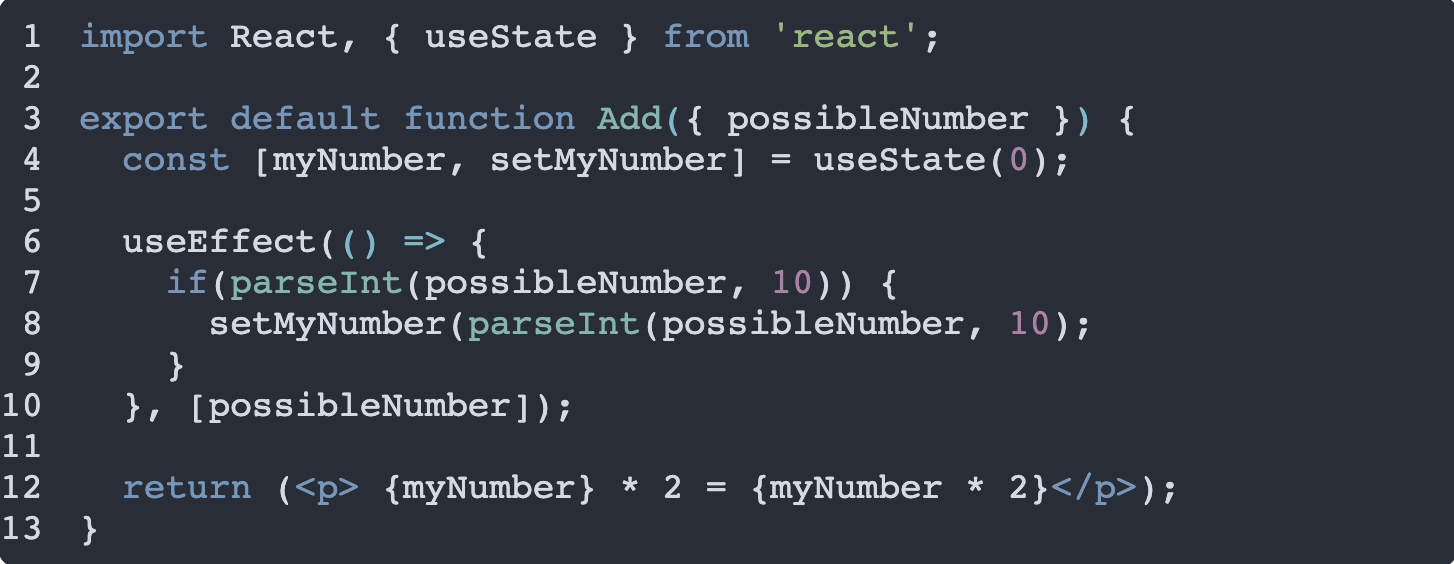