In this post we cover how to programmatically navigate using React Router
How to programmatically navigate using React Router
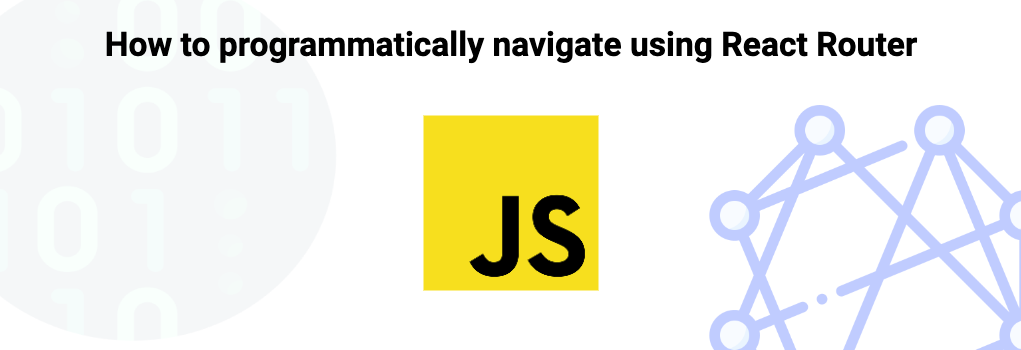
When using React Router it is possible to programmatically navigate using the useNavigate
hook.
1import { useNavigate } from "react-router-dom";
2
3export function CoffeeButton() {
4 const navigate = useNavigate();
5
6 function handleClick() {
7 navigate("/arabica-coffee");
8 }
9
10 return (
11 <button type="button" onClick={handleClick}>
12 Look at our coffee
13 </button>
14 );
15}
React Router
React Router is a client side router library built for use in React that helps with navigation in SPAs (Single Page Applications).
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Further resources
Related topics
How to pass a React component as a prop in TypeScriptWhat is Formik touched?How to use React Router with an optional path parameterHow to use Apollo useQuery with multiple queriesHow to use css variables with ReactHow to use window.addEventListener with react hooksHow to use setState from within useEffectHow to redirect in ReactJSHow to use apollo client without hooks (useQuery, and useMutation)How to use useContext in functional componentsReact.memo vs useMemoHow to make and use a Google autocomplete react hookHow to pass props to children in ReactHow to use dangerouslySetInnerHTML in ReactHow to set the selected option on a select element with React and JSXHow to test custom react hooksReact.Fragment vs divWhat is the difference between useMemo and useCallback?When to use useImperativeHandleWhat are the differences between React.Fragment vs <>What is hydration in React based applications?What are Expo modules in React Native
Save code?
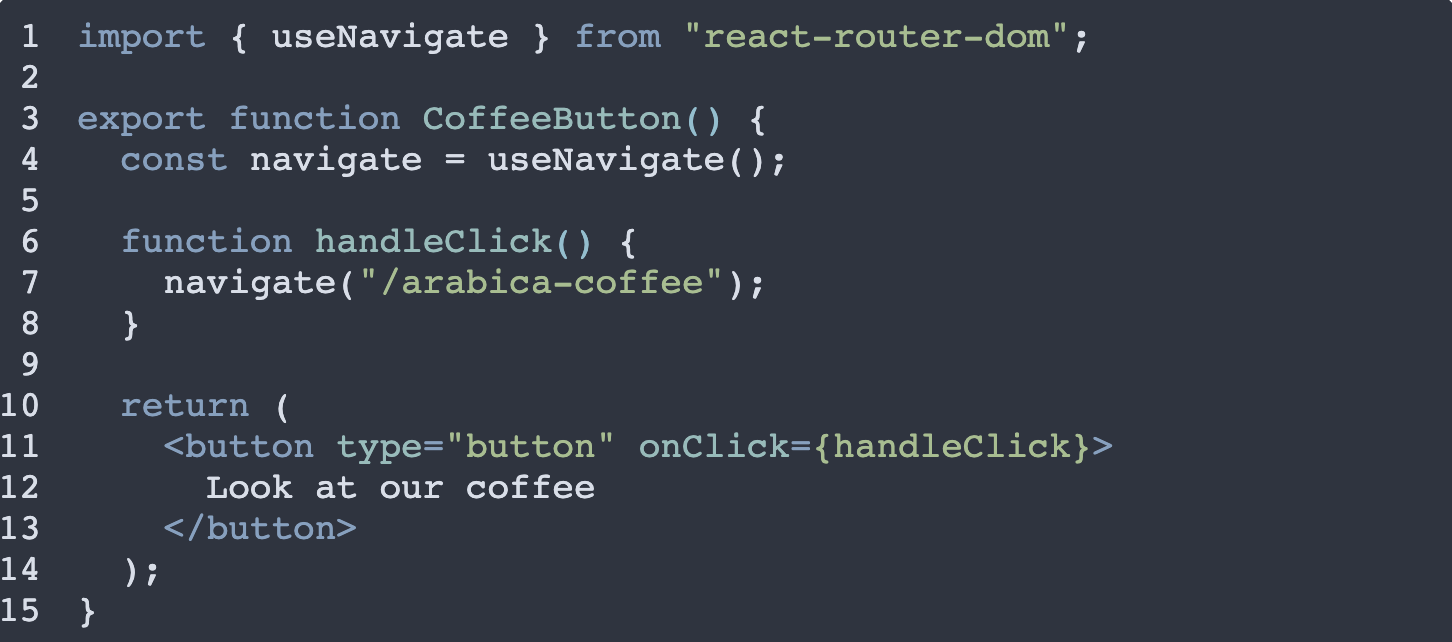