In this post we cover how to use dangerouslySetInnerHTML in React
How to use dangerouslySetInnerHTML in React
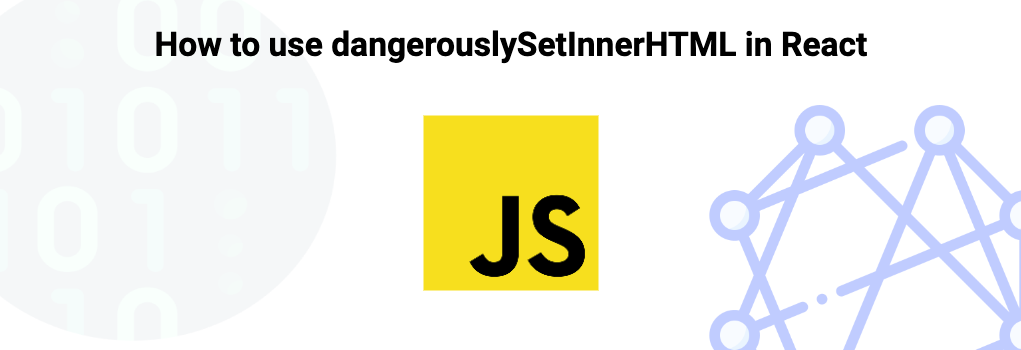
React has the ability to set the inner html of elements from html contained within strings using the dangerouslySetInnerHTML
property on html elements which can be useful in some cases.
Here is an example of using the dangerouslySetInnerHTML
property.
1export function Coffee() {
2 const coffeeHtmlString =
3 "<ul><li>Robusta</li><li>Arabica</li></ul>";
4 return (
5 <div>
6 <h1>Coffee</h1>
7 <div
8 dangerouslySetInnerHTML={{
9 __html: coffeeHtmlString
10 }}
11 />
12 </div>
13 );
14}
The above React code will output the following HTML:
1<div>
2 <h1>Coffee</h1>
3 <div>
4 <ul>
5 <li>Robusta</li>
6 <li>Arabica</li>
7 </ul>
8 </div>
9</div>
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
Further resources
Related topics
Save code?
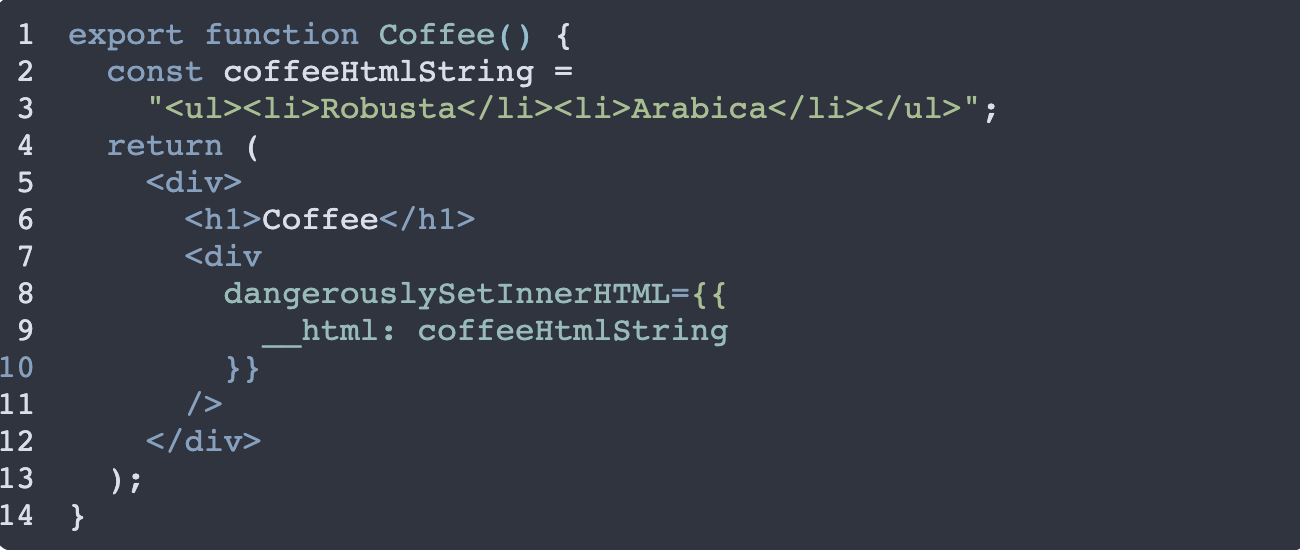