In this post we cover how to use useContext in functional components
How to use useContext in functional components
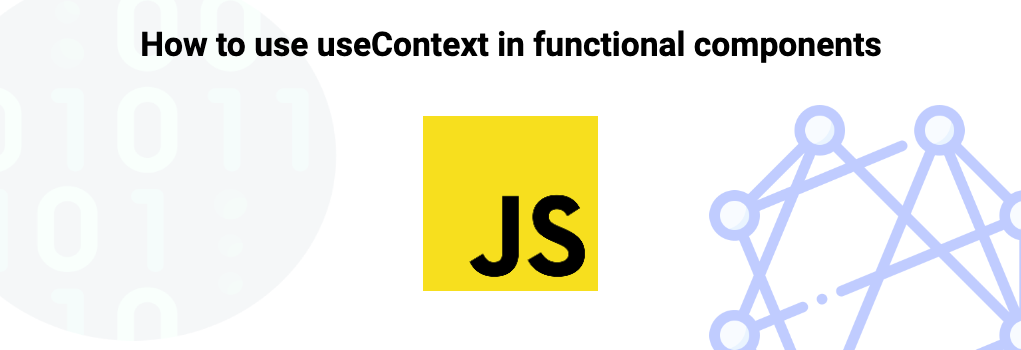
1import React from "react"
2
3// define the context structure/default values
4const CoffeeContextDefaults = {
5 coffee: "robusta",
6 setCoffee: () => {},
7};
8
9// create the context with the default values
10export const CoffeeContext =
11 React.createContext(CoffeeContextDefaults);
12
13export default function Root() {
14 // create a stateful value for the context properties
15 // to enable updating.
16 const [coffee, setCoffee] = useState(
17 CoffeeContextDefaults.coffee
18 );
19
20 return (
21
22 {/* pass the stateful values into the context "Provider"
23 Remember, these are instead of the default context values. */}
24 <CoffeeContext.Provider value={{ coffee, setCoffee }}>
25
26 {/* Components or children in here */}
27 <App />
28 </CoffeeContext.Provider>
29 )
30}
31
32export function App() {
33 // get values from the context be using the useContext hook
34 const { coffee, setCoffee } = useContext(CoffeeContext);
35
36 return (
37 <>
38
39 {/* display coffee value from context */}
40 <p>Selected Coffee: {coffee}</p>
41
42 {/* add button that when it is clicked on will update the "coffee"
43 value in the context and cause a rerender */}
44 <button onClick={() => setCoffee('Arabica')}>
45 Click to change to Arabica
46 </button>
47
48 </>
49 );
50}
To sumarise how to use useContext in functional components and explain what is happening in the above code:
- Create a default structure for the context containing default values
- Create a context using
<a href="/definition/react/">React</a>.createContext
and passing the the default structure/values. - In the parent component where the "Provider" will be created create a stateful version of the default structure/values used in the context.
- Wrap your component in the context provider and pass in the stateful version of the structure/values.
- This is now the context created and can be accessed from any child component.
- To access the context in the child component, use the
useContext
react hook and supply the context (the value created from running<a href="/definition/react/">React</a>.createContext
), in this caseCoffeeContext
. - The value returned from the
useContext
hook will be the full context with all the values within.
Functional Component
A functional component in React refers to a normal JavaScript function, that is used as a component and can accept props and return React elements.
This is often used to differentiate between a functional component(ordinary function) and a Class Component in React.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
Context
Context is a term often used to describe a shared store of data that can be easily accessed, and updated.
Further resources
Related topics
Save code?
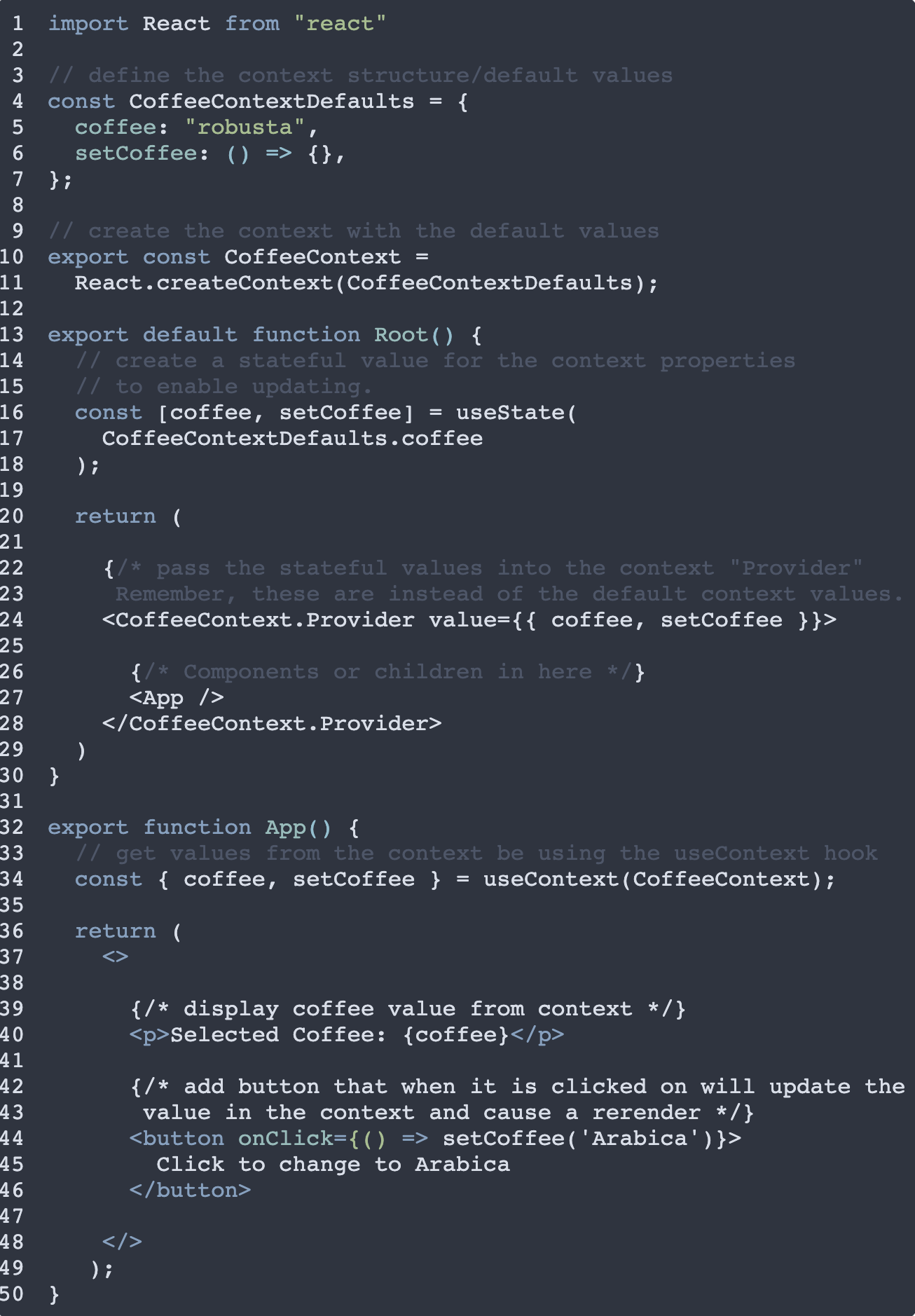