In this post we cover how to redirect in ReactJS
How to redirect in ReactJS
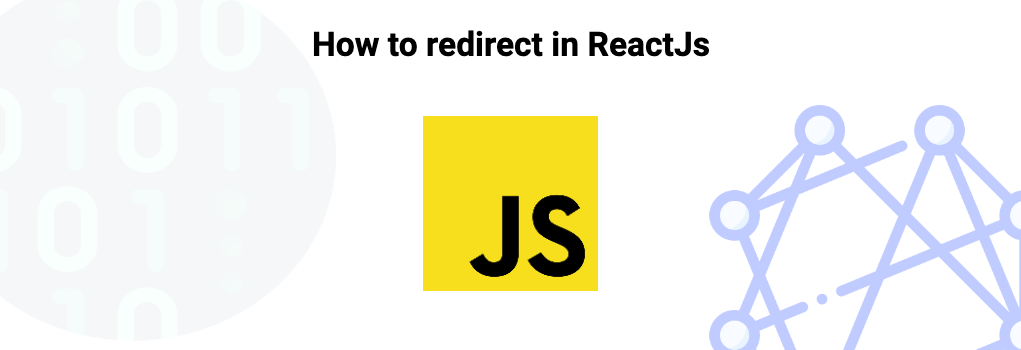
Redirecting in ReactJs or JavaScript can only partially be achieved. Where the technologies are client side they cannot fully replicate a redirection and instead only emulate one.
With that said, there is very little difference for the end user.
In this post we will look into a couple of vanilla JS methods of redirection that can be used in ReactJS as well as some library methods for redirecting as well.
Here are a couple of methods of achieving client side redirection in Vanilla JS that can be used in React JS as well:
1// Location API replace method:
2window.location.replace('https://google.com');
3
4// Location API reassignment of href property:
5window.location.href = 'https://google.com';
Both of the above options make use of the Location API which is present on most browsers.
Both of the options will navigate the user to a new page, the main difference between window.location.replace and window.location.href is that by using the replace method you will replace the current URL in the windows history object with the one you are navigating to, whilst reassigning the href property will add to the history object instead.
What this means is that window.location.replace will navigate the user to the new page with the window keeping no history of the current page, so that if the user attempts to navigate back they will be taken to the page before the replace page. And instead is the href reassignment was used then the user would be taken back to the current page.
Here are some examples:
1// window.location.replace flow:
2// initial flow: route-1 => redirect-route => route-3
3// user clicks the back button
4// user is taken to route-1
5
6// window.location.href flow:
7// initial flow: route-1 => redirect-route => route-3
8// user clicks the back button
9// user is taken to redirect-route
This is important to note because depending on the use case a user could get stuck in a redirection loop never being able to return to the page they want to.
Here are some options for use with popular React navigation libraries:
react-router-dom v6 with React Hooks:
1const navigate = useNavigation();
2navigate("/redirect-to-page", { replace: true });
in the above example the replace property is set to ture and this will do the same as the replace in the Location API by replacing the item in the history rather than creating a new addition.
react-router-dom v5 with React Hooks:
1const history = useHistory();
2history.push("/redirect-to-page");
react-router-dom v5 with JSX:
1import { Redirect } from 'react-router-dom'
2…
3<Redirect to='/redirect-to-page' />
React Router
React Router is a client side router library built for use in React that helps with navigation in SPAs (Single Page Applications).
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
JSX
JSX stands for "JavaScript XML" which in short means that you can use XML in JavaScript which is syntactic sugar for instead of writing out many function calls to create elements, you can just write the elements as if you were writing HTML or XML.
JSX is a concept introduced by React and is used heavily in React applications to build out components.
It is worth noting that the use of JSX is optional and you can still create elements by calling the appropriate functions.
React Hooks
React hooks enable to to manage state in a functional way.
React hooks were introduced in React 16.8. Prior to them to use any kind of state in a component you would need to write your code as class components.
With the addition of React hooks, you can simply create state in a standard functional component by making use of one of the many hooks available.
Two of the basic hooks are useState, and useEffect.
useState enables you to create, set and access a state variable.
useEffect will enable you to run side effects when state variables or props change with the use of a callback containing your code and a dependancy array where anytime a state variable or prop in the dependancy array changes the useEffect hook will run your callback.
As a general rule React hooks always start with the word use
.
Along with all the hooks provided in the React library, you can create custom hooks by making use of a combination of the hooks provided in the library.
Location API
The Location API in JavaScript is used to interact with the URL and navigation elements of document and window objects.
By using it you can assign new URL's which would navigate the page away, redirect, get data about the URL such as the query parameters and more.
The most common uses are to get data from the URL, or navigate the window.
Further resources
Related topics
Save code?
