In this post we cover how to get postcodes from Google Places and Google Maps with JavaScript
How to get postcodes from Google Places and Google Maps with JavaScript
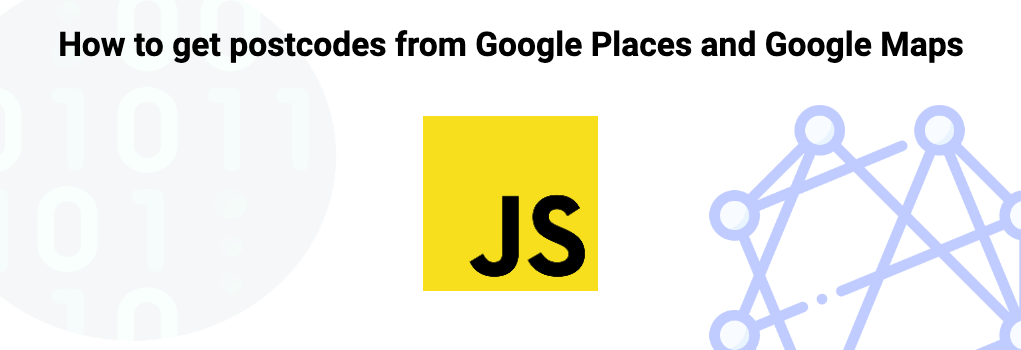
It is possible to get postcodes from the Google Places API, although it is not a large feature due to how postcodes work. Depending on the country you are in postcodes or zipcodes might be more or less specific and therefore have more or less importance when it comes to addresses.
In the UK for example it is common for the postcode to be an essential part of the address after which you only need to specify a house number to get the full address.
Here is the first option of getting postcodes from the Google Places API in JavaScript.
Firstly, enabling/importing the Google Places API:
1<script
2 type="text/javascript"
3 src="//maps.googleapis.com/maps/api/js?key=YOUR_API_KEY_GOES_HERE&language=en&libraries=places"
4></script>
Secondly some JavaScript code to get postcodes directly from the Google Places API:
1export async function placeIdToPostcode(placeId) {
2 return new Promise((resolve, reject) => {
3 if (!placeId) reject("placeId not provided");
4
5 try {
6 new window.google.maps.places.PlacesService(
7 document.createElement("div")
8 ).getDetails(
9 {
10 placeId,
11 fields: ["address_components"],
12 },
13 details => {
14 let postcode = null;
15 details?.address_components?.forEach(entry => {
16 if (entry.types?.[0] === "postal_code") {
17 postcode = entry.long_name;
18 }
19 })
20 return resolve(postcode);
21 }
22 )
23 } catch (e) {
24 reject(e);
25 }
26 });
27}
In the above code the function will convert a google placeId into a postcode. In the Google Places API all locations should have a placeId making it easy to provide.
An alternative method to getting the postcodes would be to get the latitude and longitude coordinates, and then passing them into an external API such as postcodes.io to then get back the postcodes.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Google Places API
The Google Places API is a HTTP service that can be accessed via network requests and offers location data such as establishments, geographic locations, or prominent points of interest.
Further resources
Related topics
Save code?
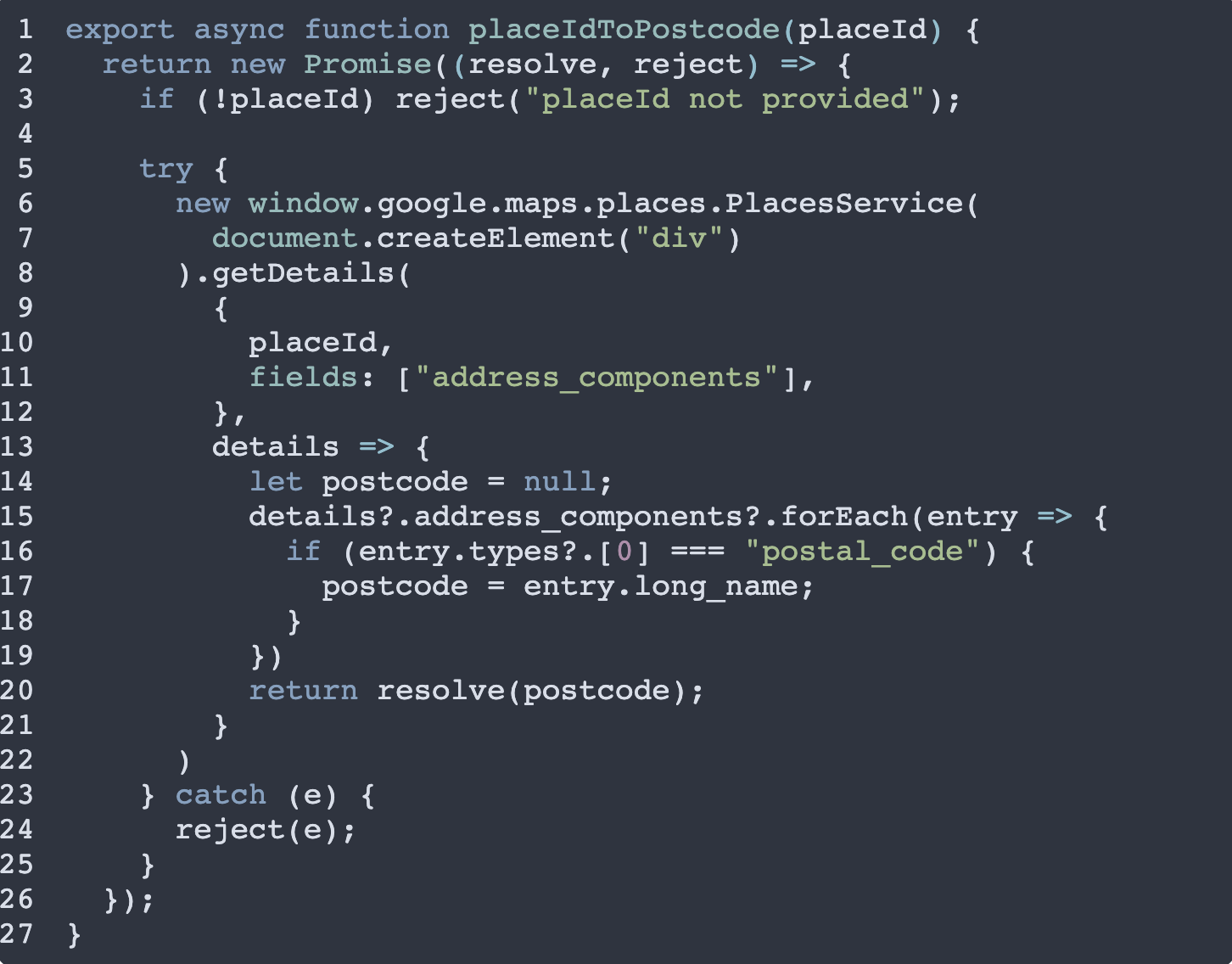