In this post we cover how to make a loop wait in JavaScript
How to make a loop wait in JavaScript
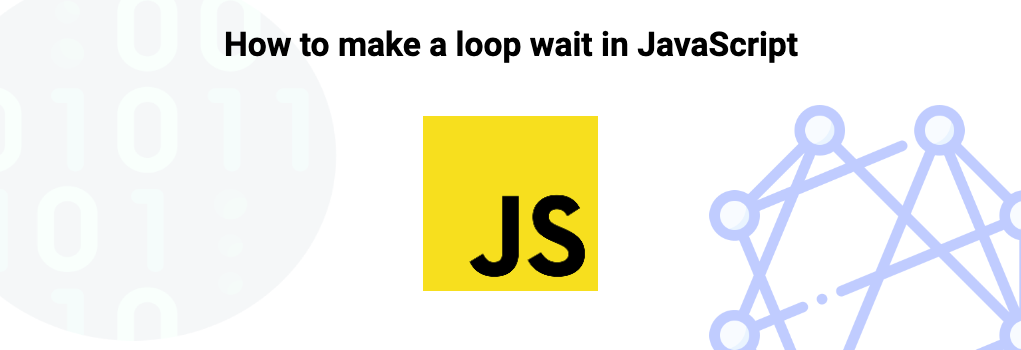
JavaScript allows for asynchronous code in a number of ways however they all make use of Promises to do so.
In order to make a loop wait in JavaScript, we just need to wait for a promise to resolve after a set timeout period.
It is worth noting at this point that whilst built in loops such as for loops do support asynchronous code, methods on Arrays such as Array.prorotype.map or Array.prorotype.forEach do not.
Here is an example of creating a delay function and then using it within a for loop in order to force the loop to wait:
1const delay = async (ms = 5000) =>
2 new Promise(resolve => setTimeout(resolve, ms));
3
4(async function() {
5 for (let i = 0; i < 10; i++) {
6 // before delay in loop
7 await delay();
8 // after delay in loop
9 }
10})();
The above code creates a function that resolves a promise after 5 seconds when invoked, and then calls this function from within a for loop using the await keyword which tells JavaScript that the promise needs to be resolved or rejected before carrying on.
One other small detail in the above code is that to run asynchronous code the loop has been wrapped in a IIFE (Immediately Invoked Function Expressions) prefixed with the async keyword which enabled the use of the await keyword from within the function.
The await keyword cannot be used outside of an asynchronous scope.
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
Asynchronous
When something is asynchronous it just means that something can happen at a later point in time and instead of waiting for it, other things can be done in the meantime.
In software engineering it means the same, but it is applied to code. When a function or block of code is asynchronous it is simply saying that we do not know when this asynchronous action will be completed so the rest of the code can continue being processed whilst we wait for it so that it does not block other actions.
IIFE
IIFE stands for "Immediately Invoked Function Expressions" which can also be referred to as "Self-invoking functions".
An IIFE is a normal function, the only difference is that it gets invoked or called immediately, and is usually used to contain a scope or to write asynchronous code that needs invoking right away.
To create an IIFE, a function is wrapped in parenthesis and then called just like any other function would be (function() { /* function body */ })();
.
Loop
A loop in software engineering is reoccurring code that runs a number of times based on what it has been provided.
There are a number of ways to run loops, however for the most part there will be a iterator variable that will be incremented in value for each iteration of the loop. The iterator is usually set to the number 0, to start and after each iteration or run of the loop the variable will be incremented or increased by 1.
In order to prevent an infinite loop which is where the code gets stuck endlessly running the loop over and over again there is often a condition set that when met will end or break out of the loop.
For example if the loop increments by 1 for each iteration, then the condition set could be that while the iterator is less than the value of 10, run the loop otherwise break it.
A common condition is to find the total length of an array and use that to determine when each item has been looped over.
Further resources
Related topics
Save code?
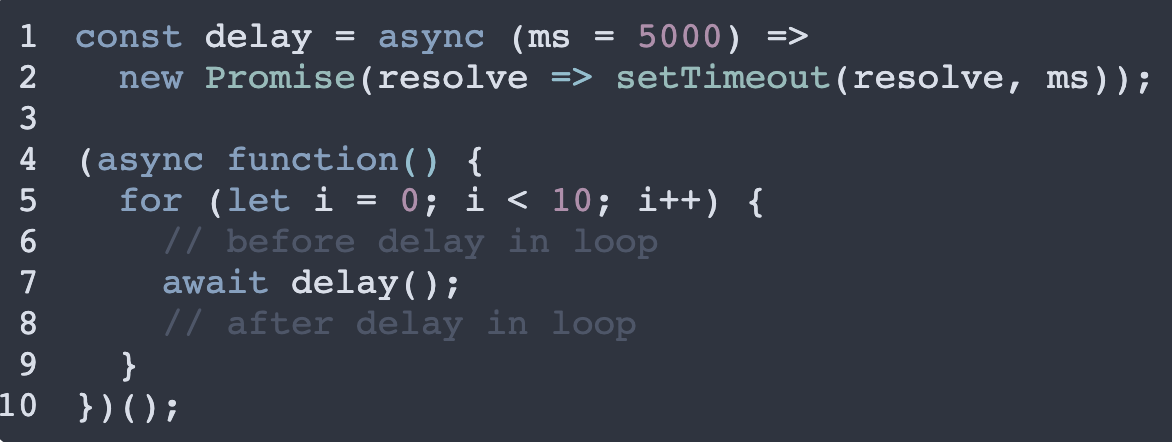