In this post we cover how to split an array in two in JavaScript
How to split an array in two in JavaScript
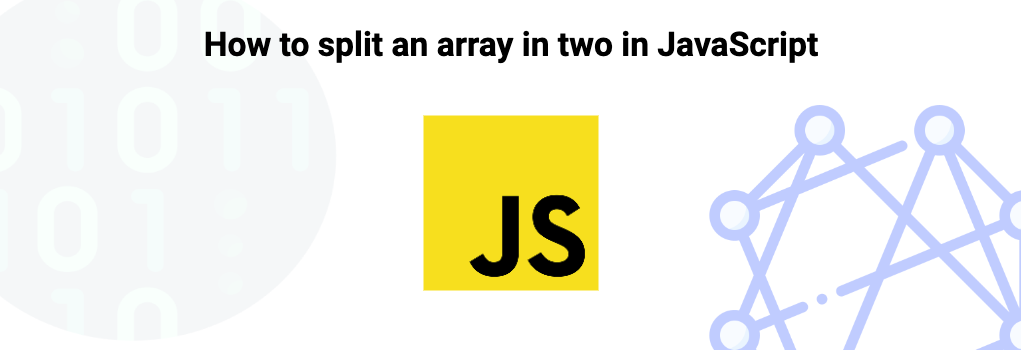
To split an array in two pieces in JavaScript the Array.prototype.slice method can be used.
Slice will return a selection of the array, so in order to split the array it can be called twice with different indexes.
Here is an example of splitting the array in half:
1const someArray = [1, 2, 3, 4];
2
3const middleIndex = Math.ceil(someArray.length / 2);
4
5const splitOne = someArray.slice(0, middleIndex);
6const splitTwo = someArray.slice(middleIndex);
7
8console.log(splitOne); // [1, 2]
9console.log(splitTwo); // [3, 4]
The same principal can be used when looking for a specific element within the array.
The only difference would be finding the index of the item needed which indexOf can be used to get.
1const someArray = [1, 2, "test", 3, 4];
2
3const itemIndex = someArray.indexOf("test");
4
5const splitOne = someArray.slice(0, middleIndex);
6const splitTwo = someArray.slice(middleIndex);
7
8console.log(splitOne); // [1, 2]
9console.log(splitTwo); // ["test", 3, 4]
JavaScript
JavaScript is a programming language that is primarily used to help build frontend applications.
It has many uses, such as making network requests, making dynamic or interactive pages, creating business logic and more.
VanillaJS
VanillaJS is a term often used to describe plain JavaScript without any additions, libraries or frameworks.
In other words when writing with VanilliaJS you are simply writing JavaScript as it comes out of the box.
Array.prototype.slice
Array.prototype.slice is a method on an array instance which can be used to return a shallow copy of a selection of the array items.
It accepts two indexes as arguments, the starting point and the ending point.
The starting point is the index that the selection starts from including the start index item in the selection.
The end index argument is where the selection will terminate but does not include the end index item.
The end index argument is optional and if not provided then the method will take the last element in the array as the final item in the selection.
A negative number can be passed into the start index argument to work backwards in the array to start the selection from the end rather than the start.
If no arguments are provided then the method will return a shallow copy of the full array.
Further resources
Related topics
Save code?
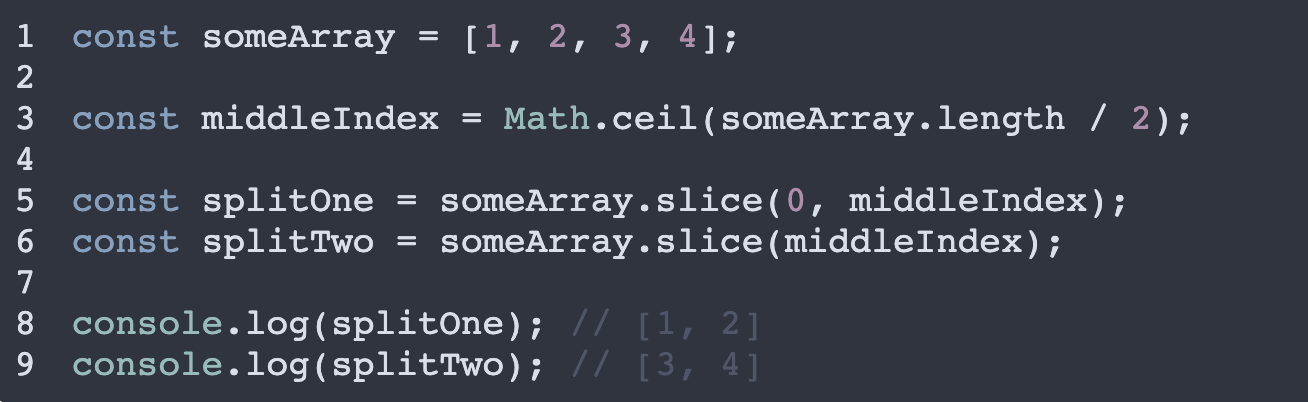