In this post we cover how to pass a React component as a prop in TypeScript
How to pass a React component as a prop in TypeScript
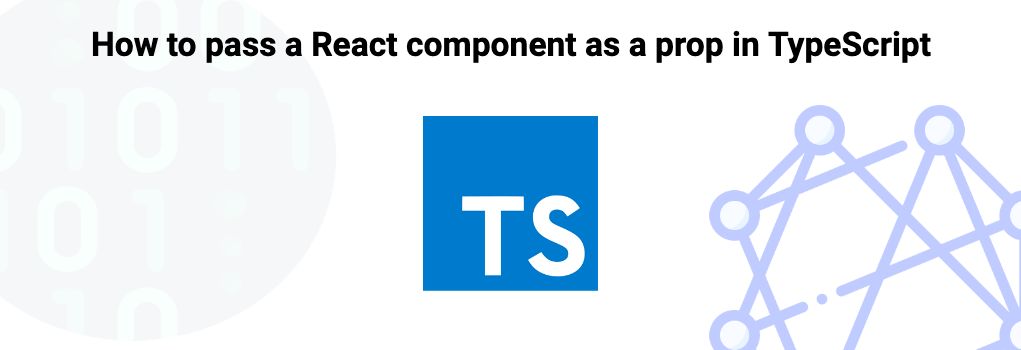
1interface ContainerProps {
2 children: React.ReactNode | React.ReactNode[];
3}
4
5export default function Container({ children }) {
6 return (
7 <div> {children} </div>
8 );
9}
In the above code we use the React.ReactNode type to describe what the "children" prop will look like.
1interface ContainerProps {
2 SomeComponent: React.FC<SomeComponentProps>;
3}
4
5export default function Container({ SomeComponent }) {
6 return (
7 <div>
8 <SomeComponent />
9 </div>
10 );
11}
In summary to pass a React component as a prop in TypeScript, you just need to provide the correct type in the props. In most cases this can be either React.ReactNode for React elements and nodes or React.FC for functional components.
Functional Component
A functional component in React refers to a normal JavaScript function, that is used as a component and can accept props and return React elements.
This is often used to differentiate between a functional component(ordinary function) and a Class Component in React.
React
React or ReactJs is a library used for developing frontend applications. Its main concepts are based around state, props, and renders.
When a state variable or prop gets updated or changed, they trigger a render.
When a render happens the appropriate parts of the application essentially get re-built using the new values to provide a new UI to the user.
It is worth noting that a prop that causes a render is just a state variable that gets passed into another component.
JSX
JSX stands for "JavaScript XML" which in short means that you can use XML in JavaScript which is syntactic sugar for instead of writing out many function calls to create elements, you can just write the elements as if you were writing HTML or XML.
JSX is a concept introduced by React and is used heavily in React applications to build out components.
It is worth noting that the use of JSX is optional and you can still create elements by calling the appropriate functions.
Further resources
Related topics
Save code?
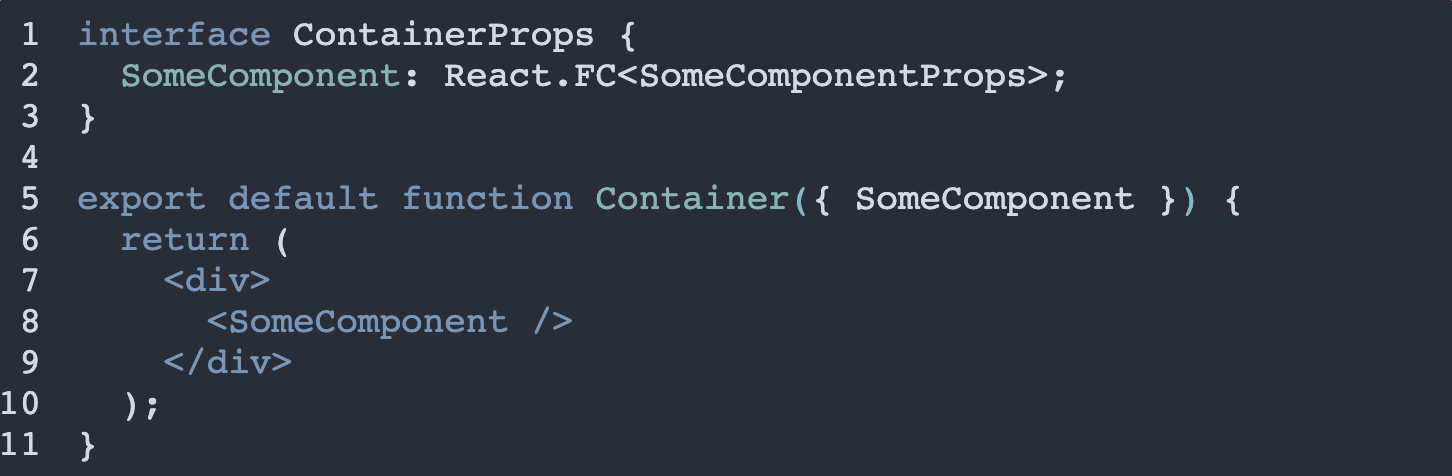