In this post we cover how to deep flatten an object in JavaScript
How to deep flatten an object in JavaScript
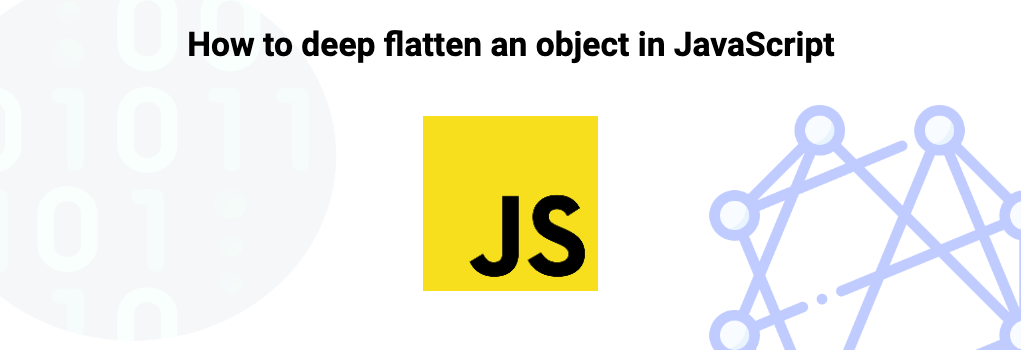
To perform a deep flatten in JavaScript from scratch we essentially need to loop over an object and replace each nested object with its values. We can do this by creating a recursive function and using the spread syntax.
Here is an example of how you can deep flatten an object in JavaScript:
1function deepFlatten(obj) {
2 const keys = Object.keys(obj);
3 let next = {};
4
5 for (let i = 0; i < keys.length; i += 1) {
6 const key = keys[i];
7 const value = obj[key];
8 if (typeof value === "object" && value !== null) {
9 next = {
10 ...next,
11 ...deepFlatten(value)
12 };
13 } else {
14 next = { ...next, [key]: value };
15 }
16 }
17
18 return next;
19}
1deepFlatten({ itemOne: "1", objItem: { itemTwo: "2", objItem2: { itemThree: "3" } } });
2// {itemOne: '1', itemTwo: '2', itemThree: '3'}
The above code should work as a good helper method to perform deep flattening of an object . But to summarise, in order to deep flatten an object you will need to loop over an object and replace each nested object with its values.
Spread Syntax
Spread syntax (...) comes from ES6 and it essentially lets you pull out the values and fields of any object, or array into something else.
For example when merging two objects you can use the spread syntax to pull all of the fields from one object into another. Or when using an array you can pull all of the array items into another array.
Another use would be in the arguments of a function.
If you want to get all arguments passed into a function as an array you can use the spread syntax to put all the arguments into an array that you name.
Lastly, as a working example, let's say you need a function that can accept many arrays of numbers as parameters and you expect it to find the sum of all the numbers across all arrays passed into it.
One way in which you could do this would be to find all the arrays passed in using spread syntax to give you an array of arrays with numbers and then combine all the arrays into a single array of numbers using a loop and spread syntax, where each iteration you would re-define the single array to be equal to the current items it has as well as the numbers from the current iteration's array.
After that you can loop over the single array of numbers adding each one and then returning the overall value.
Recursive Function
A recursive function in short is a function that calls itself. In computer science and software engineering recursive functions can be useful tools for building out algorithms in an effective way, and reducing the amount of code that needs writing.
All recursive functions will need a break to ensure it does not cause an infinite loop. This break usually happens when the criteria of the function is met or there is nothing left to recurse on.
Further resources
Related topics
Save code?
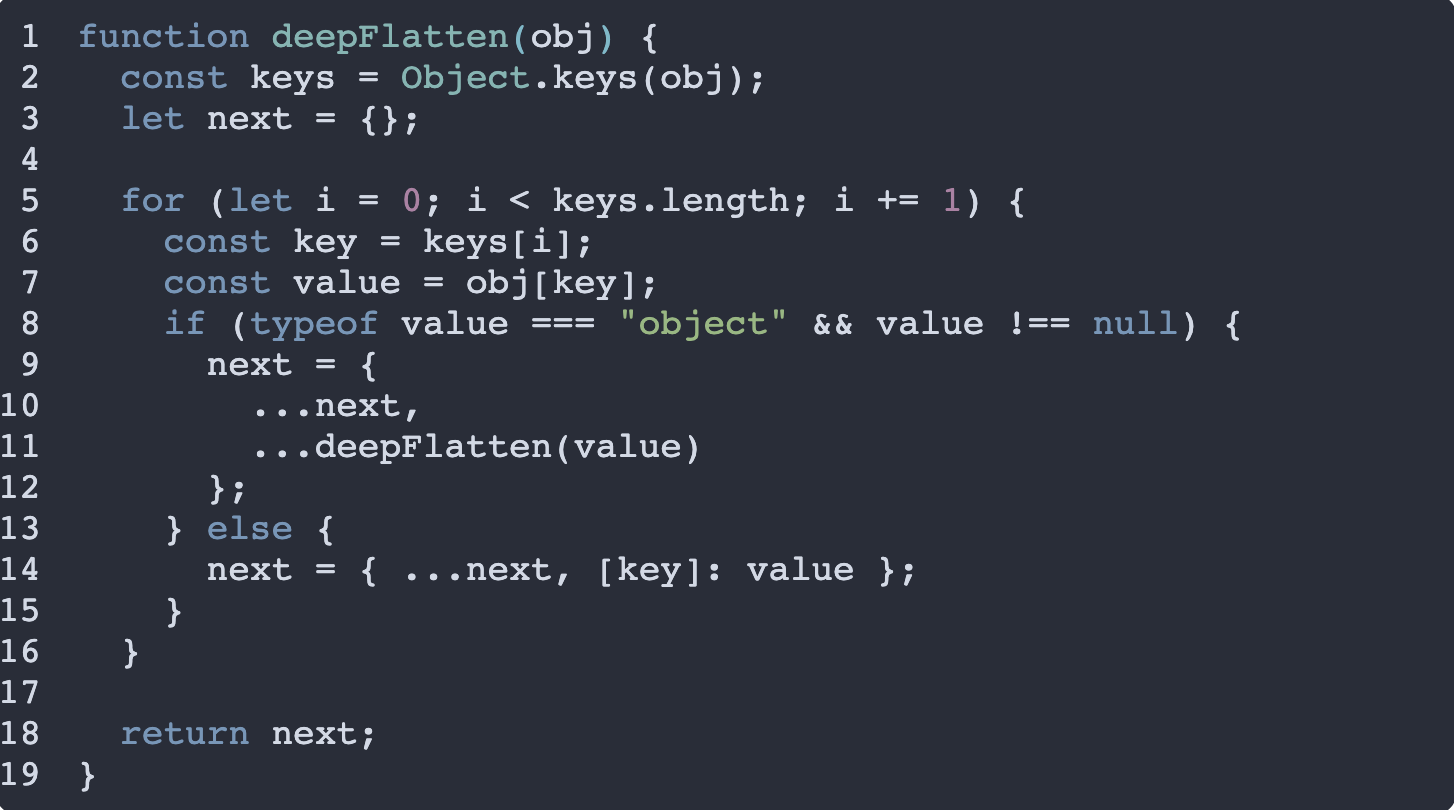